Introduction
Creating a CRUD (Create, Read, Update, Delete) application is a fundamental exercise for developers, and Node.js provides a versatile platform for building such applications efficiently. In this article, we’ll walk through the process of building a simple CRUD application using Node.js and Express, a popular web application framework.
Setting Up the Project
Before diving into the CRUD operations, let’s set up our Node.js project. First, ensure Node.js is installed on your machine. Then, follow these steps:
- Create a new directory for your project and navigate into it using the terminal:
mkdir node-crud-app cd node-crud-app
- Initialize a new Node.js project:
npm init -y
- Install Express:
npm install express
- Create the main file for your application, e.g.,
app.js
.
Implementing CRUD Operations
1. Setting Up Express
In your app.js
file, set up the basic structure for your Express application:
const express = require('express'); const app = express(); const port = 3000; app.use(express.json()); // Enable JSON parsing for request bodies app.listen(port, () => { console.log(`Server listening at http://localhost:${port}`); });
2. Creating a Data Store
For simplicity, we’ll use an array as our in-memory data store. Add this at the beginning of your app.js
file:
let dataStore = [ { id: 1, name: 'Item 1' }, { id: 2, name: 'Item 2' }, // ... more data ];
3. Implementing CRUD Endpoints
Now, let’s implement the CRUD endpoints:
Create (POST)
app.post('/items', (req, res) => { const newItem = req.body; newItem.id = dataStore.length + 1; dataStore.push(newItem); res.json(newItem); });
Read All (GET)
app.get('/items', (req, res) => { res.json(dataStore); });
Read One (GET)
app.get('/items/:id', (req, res) => { const itemId = parseInt(req.params.id); const item = dataStore.find(item => item.id === itemId); if (!item) { return res.status(404).json({ message: 'Item not found' }); } res.json(item); });
Update (PUT)
app.put('/items/:id', (req, res) => { const itemId = parseInt(req.params.id); const updatedItem = req.body; dataStore = dataStore.map(item => (item.id === itemId ? { ...item, ...updatedItem } : item)); res.json(updatedItem); });
Delete (DELETE)
app.delete('/items/:id', (req, res) => { const itemId = parseInt(req.params.id); dataStore = dataStore.filter(item => item.id !== itemId); res.json({ message: 'Item deleted successfully' }); });
Testing the CRUD Operations
- Run your Node.js application:
node app.js
- Use tools like Postman or curl to test your CRUD operations:
- Create: Send a POST request to
http://localhost:3000/items
. - Read All: Send a GET request to
http://localhost:3000/items
. - Read One: Send a GET request to
http://localhost:3000/items/:id
(replace:id
with an actual item ID). - Update: Send a PUT request to
http://localhost:3000/items/:id
(replace:id
with an actual item ID). - Delete: Send a DELETE request to
http://localhost:3000/items/:id
(replace:id
with an actual item ID).
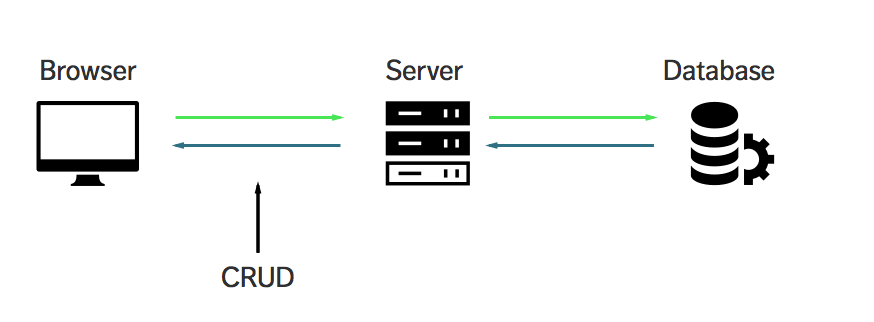
Congratulations! You’ve successfully built a simple CRUD application with Node.js and Express. This foundation can be extended to more complex scenarios with databases, authentication, and additional features based on your project requirements.