Sessions and cookies are fundamental concepts in web development, enabling developers to manage user data and maintain state across multiple requests. In this guide, we’ll delve into PHP sessions and cookies, exploring their functionalities, usage, and best practices.
PHP Sessions
What are Sessions?
A session is a way to store information across multiple pages or requests. It allows you to preserve user data, such as login status, preferences, or any other information, throughout a user’s interaction with a website.
Starting a Session
In PHP, starting a session is simple. Use the session_start()
function at the beginning of your script:
<?php session_start(); // Now you can set or retrieve session variables $_SESSION['username'] = 'john_doe'; ?>
Setting and Retrieving Session Variables
You can set and retrieve session variables using the $_SESSION
superglobal:
<?php session_start(); // Set a session variable $_SESSION['user_id'] = 123; // Retrieve a session variable $userID = $_SESSION['user_id']; ?>
Destroying a Session
To end a session and clear all associated session data, use session_destroy()
:
<?php session_start(); // Destroy the session session_destroy(); ?>
Session Security Considerations
- Session Hijacking: Use
session_regenerate_id(true)
to regenerate the session ID periodically or after user authentication. - Session Fixation: Avoid using session IDs from user inputs and regenerate the session ID after login.
PHP Cookies
What are Cookies?
Cookies are small pieces of data stored on the client-side (user’s browser). They are often used to store user preferences, track user behavior, and maintain state between requests.
Setting Cookies
You can set cookies in PHP using the setcookie()
function:
<?php // Set a cookie with a name, value, and expiration time (in seconds) setcookie('user_id', '123', time() + 3600, '/'); ?>
Retrieving Cookies
Retrieve cookies using the $_COOKIE
superglobal:
<?php // Retrieve a cookie $userID = $_COOKIE['user_id']; ?>
Deleting Cookies
To delete a cookie, set its expiration time to a past date:
<?php // Delete a cookie setcookie('user_id', '', time() - 3600, '/'); ?>
Cookie Security Considerations
- Secure Flag: Set the
secure
flag to true for cookies transmitted over HTTPS only. - HttpOnly Flag: Set the
HttpOnly
flag to true to prevent JavaScript access, enhancing security against XSS attacks. - SameSite Attribute: Use the
SameSite
attribute to control when cookies are sent. Options include'None'
,'Lax'
, and'Strict'
.
Combining Sessions and Cookies
Often, sessions use cookies to store the session ID on the client side. The session ID is then used to associate subsequent requests with the ongoing session. PHP handles this process behind the scenes when you use session_start()
.
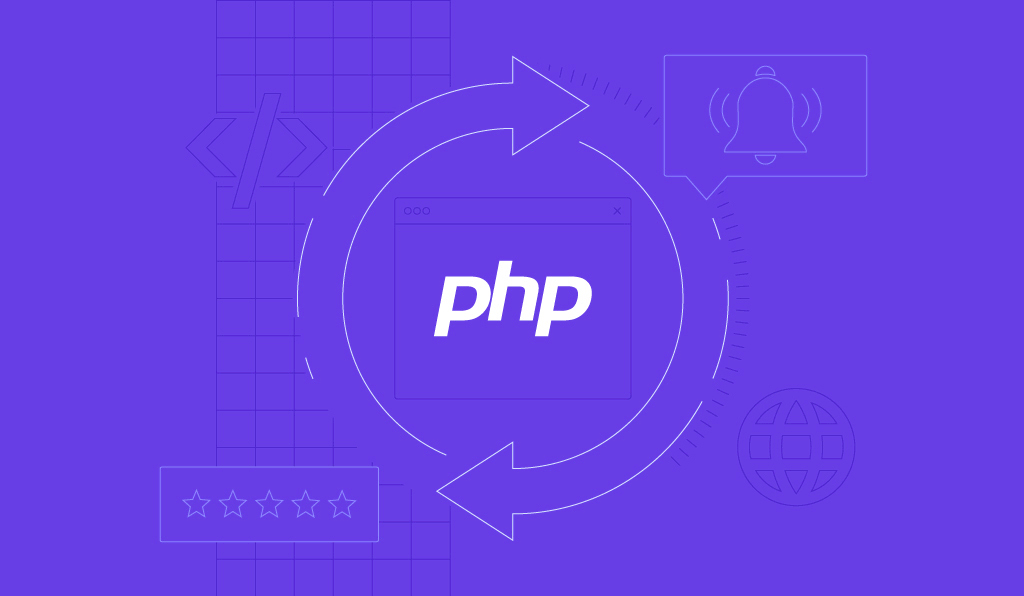
Conclusion
Understanding PHP sessions and cookies is crucial for building dynamic and interactive web applications. Sessions help maintain user-specific data throughout their visit, while cookies enable data persistence across requests. By implementing best practices and considering security measures, you can create robust and secure web applications that deliver a seamless user experience.