XML (eXtensible Markup Language) is a widely used format for storing and exchanging structured data. PHP provides robust support for working with XML through various functions and libraries. In this article, we’ll explore the basics of handling XML in PHP and provide sample code for common use cases.
Creating XML in PHP
To create XML in PHP, you can use the DOM extension. The DOM extension provides a set of classes for working with XML documents. Let’s create a simple XML document using PHP:
<?php // Create a new DOMDocument $doc = new DOMDocument('1.0', 'UTF-8'); // Create the root element $root = $doc->createElement('books'); $doc->appendChild($root); // Add book elements $book1 = $doc->createElement('book'); $book1->setAttribute('id', '1'); $book1->appendChild($doc->createElement('title', 'The Great Gatsby')); $book1->appendChild($doc->createElement('author', 'F. Scott Fitzgerald')); $root->appendChild($book1); $book2 = $doc->createElement('book'); $book2->setAttribute('id', '2'); $book2->appendChild($doc->createElement('title', 'To Kill a Mockingbird')); $book2->appendChild($doc->createElement('author', 'Harper Lee')); $root->appendChild($book2); // Save the XML to a file $doc->save('books.xml'); ?>
This PHP script creates a simple XML document with two book elements, each containing a title and an author. The resulting XML file (books.xml
) looks like this:
<?xml version="1.0" encoding="UTF-8"?> <books> <book id="1"> <title>The Great Gatsby</title> <author>F. Scott Fitzgerald</author> </book> <book id="2"> <title>To Kill a Mockingbird</title> <author>Harper Lee</author> </book> </books>
Parsing XML in PHP
To parse XML in PHP, you can use SimpleXML, which provides an easy-to-use API for reading and manipulating XML documents. Let’s read the XML file we created in the previous example:
<?php // Load the XML file $xml = simplexml_load_file('books.xml'); // Iterate over book elements foreach ($xml->book as $book) { echo 'Book ID: ' . $book['id'] . "\n"; echo 'Title: ' . $book->title . "\n"; echo 'Author: ' . $book->author . "\n\n"; } ?>
This PHP script loads the books.xml
file using SimpleXML and iterates over the book elements, displaying the book ID, title, and author.
Modifying XML in PHP
Let’s modify the XML file by adding a new book:
<?php // Load the XML file $xml = simplexml_load_file('books.xml'); // Add a new book $newBook = $xml->addChild('book'); $newBook->addAttribute('id', '3'); $newBook->addChild('title', '1984'); $newBook->addChild('author', 'George Orwell'); // Save the modified XML to a file $xml->asXML('books_modified.xml'); ?>
This script adds a new book element to the XML file and saves the modified XML as books_modified.xml
.
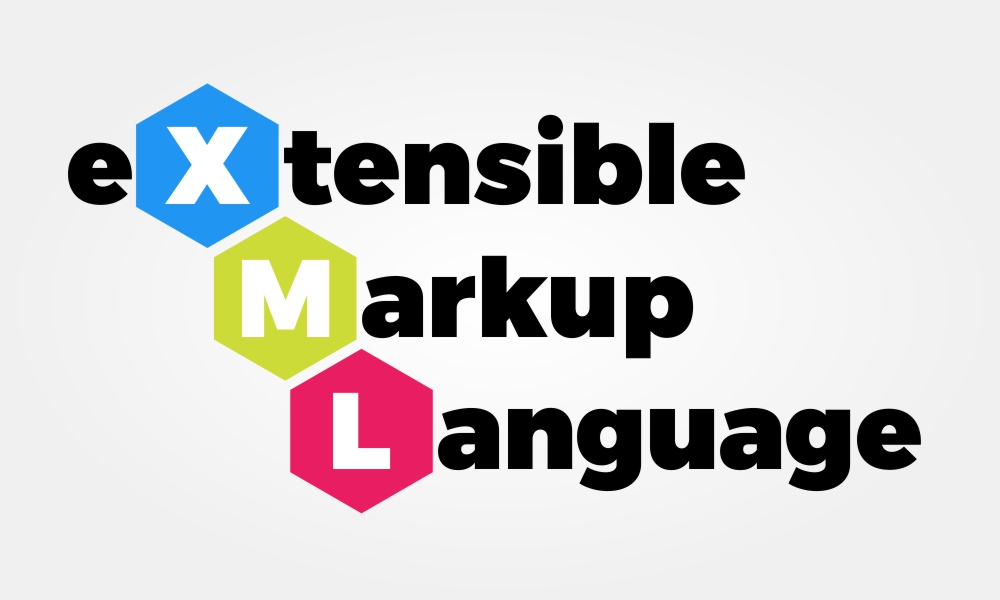
Conclusion
Handling XML in PHP is a fundamental skill for web developers, especially when dealing with data exchange or configuration settings. Whether you’re creating, parsing, or modifying XML documents, PHP provides powerful tools for working with XML data. In this article, we’ve covered the basics of creating, parsing, and modifying XML in PHP, and the provided sample code serves as a foundation for more advanced XML-related tasks in your PHP projects.