Implementing Continuous Integration and Continuous Deployment (CI/CD) is crucial for automating the process of building, testing, and deploying your applications. Below are simplified examples for setting up CI/CD pipelines for web services in C# (.NET Core), Java (Spring Boot), and Golang using GitHub Actions as the CI/CD platform. These examples assume your source code is hosted on GitHub.
C# (.NET Core) CI/CD with GitHub Actions:
Create a .github/workflows/dotnet.yml
file in your project:
name: CI/CD for .NET Core on: push: branches: - main jobs: build: runs-on: ubuntu-latest steps: - name: Checkout repository uses: actions/checkout@v2 - name: Setup .NET Core uses: actions/setup-dotnet@v1 with: dotnet-version: 5.0 - name: Restore dependencies run: dotnet restore - name: Build run: dotnet build --configuration Release deploy: runs-on: ubuntu-latest needs: build steps: - name: Checkout repository uses: actions/checkout@v2 - name: Deploy to AWS Elastic Beanstalk run: | aws configure set aws_access_key_id ${{ secrets.AWS_ACCESS_KEY }} aws configure set aws_secret_access_key ${{ secrets.AWS_SECRET_KEY }} aws elasticbeanstalk create-application-version --application-name MyApp --version-label $GITHUB_SHA --source-bundle S3Bucket=my-s3-bucket,S3Key=$GITHUB_SHA.zip aws elasticbeanstalk update-environment --environment-name MyEnvironment --version-label $GITHUB_SHA env: AWS_REGION: us-west-2
In this example, you need to replace MyApp
, MyEnvironment
, my-s3-bucket
, AWS_ACCESS_KEY
, and AWS_SECRET_KEY
with your specific values.
Java (Spring Boot) CI/CD with GitHub Actions:
Create a .github/workflows/java.yml
file in your project:
name: CI/CD for Java on: push: branches: - main jobs: build: runs-on: ubuntu-latest steps: - name: Checkout repository uses: actions/checkout@v2 - name: Setup Java uses: actions/setup-java@v2 with: distribution: 'adopt' java-version: '11' - name: Build with Maven run: mvn clean install deploy: runs-on: ubuntu-latest needs: build steps: - name: Checkout repository uses: actions/checkout@v2 - name: Deploy to AWS Elastic Beanstalk run: | aws configure set aws_access_key_id ${{ secrets.AWS_ACCESS_KEY }} aws configure set aws_secret_access_key ${{ secrets.AWS_SECRET_KEY }} aws elasticbeanstalk create-application-version --application-name MyApp --version-label $GITHUB_SHA --source-bundle S3Bucket=my-s3-bucket,S3Key=$GITHUB_SHA.zip aws elasticbeanstalk update-environment --environment-name MyEnvironment --version-label $GITHUB_SHA env: AWS_REGION: us-west-2
In this example, you need to replace MyApp
, MyEnvironment
, my-s3-bucket
, AWS_ACCESS_KEY
, and AWS_SECRET_KEY
with your specific values.
Golang CI/CD with GitHub Actions:
Create a .github/workflows/go.yml
file in your project:
name: CI/CD for Golang on: push: branches: - main jobs: build: runs-on: ubuntu-latest steps: - name: Checkout repository uses: actions/checkout@v2 - name: Setup Go uses: actions/setup-go@v2 with: go-version: 1.17 - name: Build run: go build deploy: runs-on: ubuntu-latest needs: build steps: - name: Checkout repository uses: actions/checkout@v2 - name: Deploy to AWS Elastic Beanstalk run: | aws configure set aws_access_key_id ${{ secrets.AWS_ACCESS_KEY }} aws configure set aws_secret_access_key ${{ secrets.AWS_SECRET_KEY }} aws elasticbeanstalk create-application-version --application-name MyApp --version-label $GITHUB_SHA --source-bundle S3Bucket=my-s3-bucket,S3Key=$GITHUB_SHA.zip aws elasticbeanstalk update-environment --environment-name MyEnvironment --version-label $GITHUB_SHA env: AWS_REGION: us-west-2
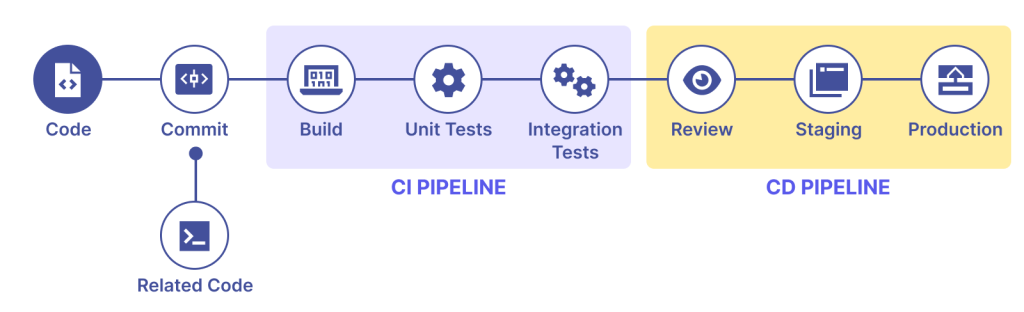
In this example, you need to replace MyApp
, MyEnvironment
, my-s3-bucket
, AWS_ACCESS_KEY
, and AWS_SECRET_KEY
with your specific values.
These examples assume AWS Elastic Beanstalk as the deployment target, but you can modify the deployment step based on your chosen hosting provider or deployment target. Also, ensure that you set up the necessary secrets in your GitHub repository for sensitive information like AWS credentials. Adjustments may be needed based on your specific project structure and requirements.