Unlocking Natural Language Processing (NLP) in C#: A Journey into Text Analysis
Natural Language Processing (NLP) is a fascinating field that empowers computers to understand, interpret, and generate human-like language. In the realm of C#, developers can harness the power of NLP through libraries and frameworks. In this article, we’ll explore the basics of NLP and demonstrate how to perform text analysis using the C# language and popular NLP libraries.
Introduction to NLP:
NLP involves the intersection of computer science, artificial intelligence, and linguistics. It aims to enable machines to process and understand human language, facilitating tasks like sentiment analysis, named entity recognition, language translation, and more.
Using NLP Libraries in C#:
Two popular NLP libraries for C# are SpaCy.NET and NLTK.NET. For this example, we’ll focus on SpaCy.NET, a .NET wrapper for the SpaCy library, a powerful and efficient library for NLP tasks.
Installing SpaCy.NET:
To get started, install the SpaCy.NET NuGet package:
Install-Package SpaCy.NET
Performing Named Entity Recognition (NER):
Named Entity Recognition is a common NLP task that involves identifying entities such as names, locations, and organizations in text. Let’s use SpaCy.NET for NER in C#.
using SpacyDotNet; using SpacyDotNet.Models; class Program { static void Main() { // Load SpaCy model using (var spacy = Spacy.Load("en_core_web_sm")) { // Perform Named Entity Recognition var result = spacy.GetNamedEntities("Microsoft is headquartered in Redmond."); // Display the results foreach (var entity in result) { Console.WriteLine($"Entity: {entity.Text}, Type: {entity.EntityType}"); } } } }
In this example, the SpaCy.NET library is used to load the English language model (en_core_web_sm
) and perform NER on a sample text.
Sentiment Analysis with ML.NET:
While SpaCy.NET is excellent for certain NLP tasks, ML.NET can be employed for more complex tasks like sentiment analysis. Here’s a brief example:
using Microsoft.ML; using Microsoft.ML.Transforms.Text; class Program { static void Main() { // Create MLContext var mlContext = new MLContext(); // Define data class public class SentimentData { [LoadColumn(0)] public bool Sentiment; [LoadColumn(1)] public string Text; } // Load data var data = mlContext.Data.LoadFromTextFile<SentimentData>("sentiment_data.csv", separatorChar: ','); // Define data processing pipeline var pipeline = mlContext.Transforms.Text.FeaturizeText("Features", "Text") .Append(mlContext.Transforms.Conversion.MapValueToKey("Label")) .Append(mlContext.Transforms.Conversion.MapKeyToValue("PredictedLabel")) .Append(mlContext.Transforms.Conversion.MapValueToKey("PredictedLabel")); // Choose a machine learning algorithm var trainer = mlContext.Transforms.Conversion.MapKeyToValue("PredictedLabel") .Append(mlContext.Transforms.Conversion.MapValueToKey("Label")) .Append(mlContext.Transforms.Conversion.MapKeyToValue("Label")) .Append(mlContext.BinaryClassification.Trainers.SdcaLogisticRegression()); // Create and train the model var model = pipeline.Append(trainer).Fit(data); // Make predictions var sentiment = new SentimentData { Text = "I love NLP in C#!" }; var prediction = mlContext.Model.MakePredictionFunction<SentimentData, SentimentPrediction>(model).Predict(sentiment); Console.WriteLine($"Predicted sentiment: {prediction.Prediction}"); } }
This example uses ML.NET to create a sentiment analysis model based on a dataset of labeled sentiments (sentiment_data.csv
).
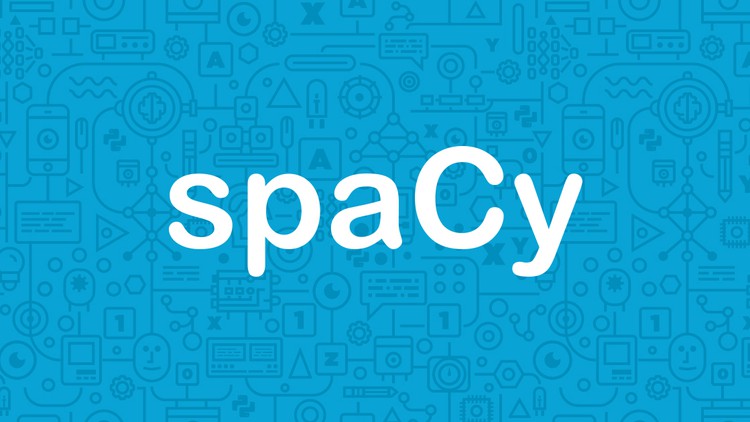
Conclusion: Empowering C# Development with NLP:
Natural Language Processing opens up a world of possibilities for C# developers, from understanding and analyzing text to extracting meaningful insights. Whether you’re working with SpaCy.NET for specific NLP tasks or leveraging ML.NET for sentiment analysis and more, integrating NLP into your C# applications can enhance their capabilities and provide a richer user experience.