Introduction to
The PHP GD library is a powerful tool for image processing and manipulation in PHP. It provides a wide range of functions to create, edit, and output images in various formats. In this article, we’ll explore the basics of using the GD library and provide sample code for common image manipulation tasks.
Getting Started with GD
To use the GD library in PHP, ensure it is enabled in your PHP installation. You can check this by looking for the GD section in the output of phpinfo()
.
If GD is not enabled, you may need to install or enable it based on your server configuration. For example, on Debian-based systems, you can install the GD library with:
sudo apt-get install php-gd
Once GD is enabled, you can start using its functions for image manipulation.
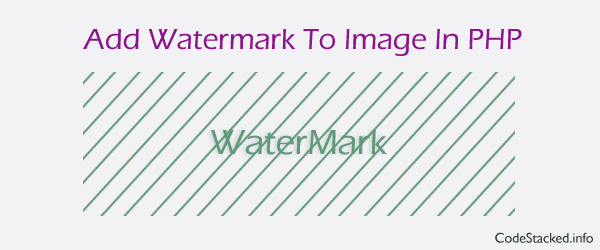
Sample Code: Creating and Displaying an Image
Let’s begin by creating a simple PHP script that generates an image and displays it in the browser.
<?php // Create a blank image with dimensions 300x150 $image = imagecreatetruecolor(300, 150); // Set a background color (white) $backgroundColor = imagecolorallocate($image, 255, 255, 255); imagefill($image, 0, 0, $backgroundColor); // Set a text color (black) $textColor = imagecolorallocate($image, 0, 0, 0); // Add text to the image $text = "Hello, GD!"; imagettftext($image, 20, 0, 50, 80, $textColor, 'arial.ttf', $text); // Set the content type header header('Content-Type: image/png'); // Output the image to the browser imagepng($image); // Free up memory by destroying the image resource imagedestroy($image); ?>
This script creates a 300×150 image, sets a white background, adds black text, and outputs it as a PNG image. Ensure that the arial.ttf
font file is available in the same directory as your script.
Save this code in a file (e.g., create_image.php
) and access it through a web browser. You should see the generated image with the text “Hello, GD!”.
Common GD Functions for Image Manipulation
1. Resizing an Image
// Load an existing image $originalImage = imagecreatefromjpeg('original.jpg'); // Get original dimensions $originalWidth = imagesx($originalImage); $originalHeight = imagesy($originalImage); // Calculate new dimensions $newWidth = 150; $newHeight = ($originalHeight / $originalWidth) * $newWidth; // Create a new blank image with the new dimensions $resizedImage = imagecreatetruecolor($newWidth, $newHeight); // Resize the original image to the new dimensions imagecopyresampled($resizedImage, $originalImage, 0, 0, 0, 0, $newWidth, $newHeight, $originalWidth, $originalHeight); // Save or display the resized image imagejpeg($resizedImage, 'resized.jpg'); // Free up memory imagedestroy($originalImage); imagedestroy($resizedImage);
2. Adding Watermark to an Image
// Load the main image $mainImage = imagecreatefrompng('main_image.png'); // Load the watermark image $watermark = imagecreatefrompng('watermark.png'); // Get dimensions of the main image and watermark $mainWidth = imagesx($mainImage); $mainHeight = imagesy($mainImage); $watermarkWidth = imagesx($watermark); $watermarkHeight = imagesy($watermark); // Set the position for the watermark $positionX = $mainWidth - $watermarkWidth - 10; $positionY = $mainHeight - $watermarkHeight - 10; // Apply the watermark to the main image imagecopy($mainImage, $watermark, $positionX, $positionY, 0, 0, $watermarkWidth, $watermarkHeight); // Save or display the image with the watermark imagepng($mainImage, 'watermarked_image.png'); // Free up memory imagedestroy($mainImage); imagedestroy($watermark);
These examples demonstrate some common tasks using the GD library. Experiment with the provided code and explore the extensive set of functions in the GD library to enhance your image manipulation capabilities in PHP. Whether you’re creating thumbnails, adding text, or applying filters, GD provides a versatile set of tools for working with images in PHP.
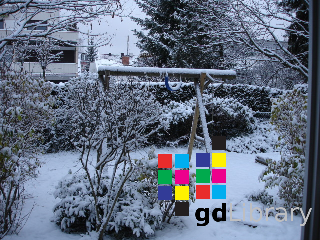