Introduction
Node.js has revolutionized server-side development, and Express.js stands out as a powerful and widely adopted framework for building web applications. In this article, we’ll delve into the key features of Express.js, its benefits, and how it simplifies the process of creating scalable and feature-rich web applications.
1. Introduction to Express.js
1.1 What is Express.js?
Express.js is a minimalist web application framework for Node.js, designed to simplify the process of building robust and scalable web applications. It provides a set of features for web and mobile application development, allowing developers to create APIs, handle routes, manage middleware, and more.
1.2 Key Features:
Express.js offers several key features that contribute to its popularity:
- Routing: Express simplifies the definition of routes, making it easy to handle HTTP methods (GET, POST, etc.) and parameters.
- Middleware: Middleware functions can be seamlessly integrated into the request-response cycle, allowing developers to perform actions before the final response is sent.
- Templating Engines: Express supports various templating engines (such as EJS, Pug, and Handlebars) to dynamically generate HTML content.
- Error Handling: Express provides a robust error-handling mechanism, making it easier to manage errors during application development.
2. Getting Started with Express.js
2.1 Installation:
To get started with Express.js, you need to install it using npm (Node Package Manager). Open your terminal and run the following command:
npm install express
2.2 Creating an Express App:
Now, let’s create a simple Express application. Create a file (e.g., app.js
) and add the following code:
// app.js const express = require('express'); const app = express(); const port = 3000; app.get('/', (req, res) => { res.send('Hello, Express!'); }); app.listen(port, () => { console.log(`Server listening at http://localhost:${port}`); });
Run the application using:
node app.js
Visit http://localhost:3000
in your browser, and you should see “Hello, Express!”
3. Handling Routes with Express.js
Express simplifies route handling, making it intuitive to respond to different HTTP methods and URL patterns. Here’s an example:
// Handling routes in Express app.get('/', (req, res) => { res.send('Home Page'); }); app.post('/api/user', (req, res) => { // Handle POST request to create a new user res.send('User Created'); }); app.put('/api/user/:id', (req, res) => { // Handle PUT request to update user details by ID res.send(`User ${req.params.id} Updated`); }); app.delete('/api/user/:id', (req, res) => { // Handle DELETE request to delete user by ID res.send(`User ${req.params.id} Deleted`); });
Express supports various HTTP methods like GET, POST, PUT, DELETE, and more, providing flexibility in handling different types of requests.
4. Middleware in Express.js
Middleware functions in Express are essential for handling tasks during the request-response cycle. They can perform operations such as authentication, logging, or modifying the request or response objects. Here’s an example:
// Middleware example const logMiddleware = (req, res, next) => { console.log(`[${new Date().toISOString()}] ${req.method} ${req.url}`); next(); }; app.use(logMiddleware); app.get('/', (req, res) => { res.send('Hello, Express with Middleware!'); });
In this example, the logMiddleware
function logs the timestamp, HTTP method, and URL of every incoming request.
5. Template Engines in Express.js
Express allows you to use template engines to dynamically generate HTML content. Here’s a basic example using the EJS (Embedded JavaScript) template engine:
5.1 Installation of EJS:
npm install ejs
5.2 Using EJS in Express:
// Using EJS as the template engine app.set('view engine', 'ejs'); app.get('/greet/:name', (req, res) => { const { name } = req.params; res.render('greet', { name }); });
Create a file named greet.ejs
in a folder named views
:
<!-- greet.ejs --> <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Greeting</title> </head> <body> <h1>Hello, <%= name %>!</h1> </body> </html>
Now, when you visit /greet/John
, it will dynamically render a greeting for the name “John.”
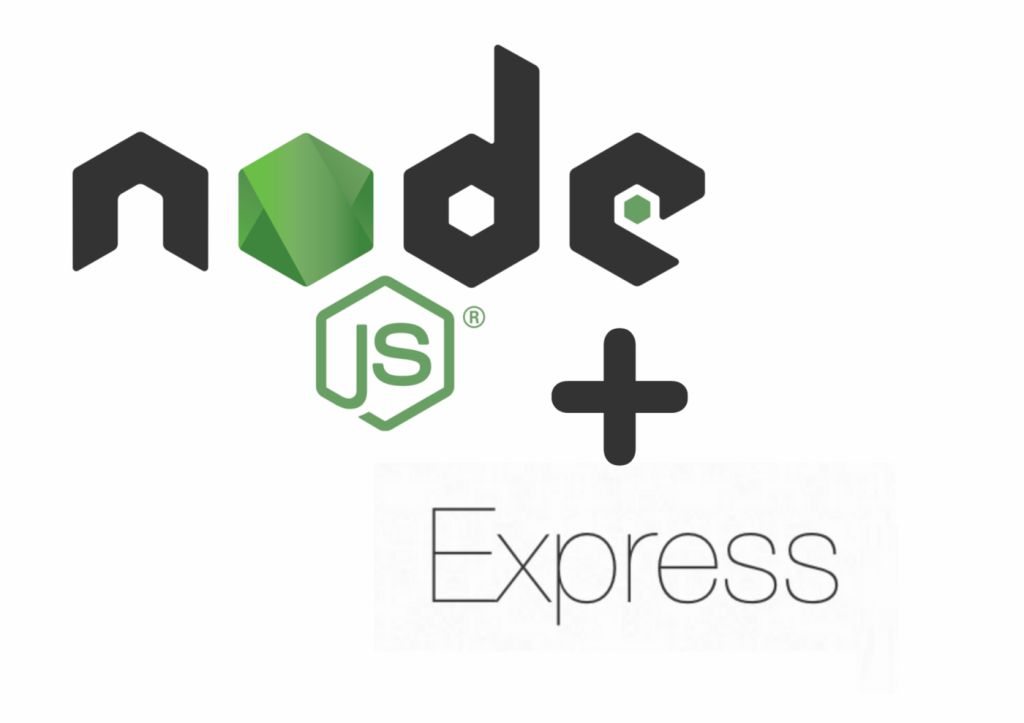
6. Conclusion
Express.js remains a dominant force in Node.js web development due to its simplicity, flexibility, and extensive ecosystem. Whether you’re building a RESTful API, a full-fledged web application, or a microservice, Express provides the tools needed to streamline development.
By understanding the basics of Express.js, handling routes, incorporating middleware, and leveraging template engines, you can harness the full potential of this framework. Explore the Express.js documentation, experiment with its features, and embark on a journey to build scalable and efficient web applications with Node.js.