Introduction
Node.js, known for its efficiency and scalability, owes much of its power to its event-driven, non-blocking I/O model. In this article, we will unravel the concepts of the event loop and asynchronous programming in Node.js, shedding light on how they contribute to the platform’s ability to handle concurrent operations.
1. Understanding the Event Loop
1.1 Single Threaded Nature:
Node.js operates on a single-threaded event loop, which means it uses a single process to handle multiple operations concurrently. While traditional multi-threaded environments create a new thread for each incoming request, Node.js leverages a single thread for handling all requests, making it highly efficient and scalable.
1.2 Non-Blocking I/O:
Node.js achieves non-blocking I/O through an asynchronous, event-driven architecture. When an asynchronous operation, such as reading from a file or making a network request, is initiated, Node.js doesn’t wait for it to complete. Instead, it continues executing other tasks. Once the operation is finished, a callback is triggered, and the result is processed.
2. The Event Loop in Action
2.1 Phases of the Event Loop:
The event loop consists of several phases, each responsible for different tasks. These phases include timers, I/O callbacks, idle, prepare, poll, check, and close callbacks. Understanding these phases helps in comprehending the flow of execution in a Node.js application.
- Timers: Handles scheduled functions using
setTimeout
andsetInterval
. - I/O Callbacks: Executes I/O-related callbacks, including those from network operations or file system events.
- Idle, Prepare: Internal phases with less significance for most applications.
- Poll: Retrieves new I/O events and executes their associated callbacks.
- Check: Executes
setImmediate
callbacks. - Close Callbacks: Executes callbacks associated with closed connections, e.g.,
socket.on('close')
.
2.2 Event Queues:
Node.js maintains multiple event queues corresponding to different phases of the event loop. When an asynchronous operation completes, its callback is placed in the appropriate queue. The event loop iterates through these queues, executing the callbacks in a non-blocking manner.
3. Asynchronous Programming in Node.js
3.1 Callbacks:
Callbacks are a fundamental concept in asynchronous programming with Node.js. A callback is a function passed as an argument to another function, which is then invoked once the operation completes. Callbacks allow developers to handle asynchronous operations without blocking the execution flow.
// Example of a callback const fetchData = (callback) => { // Simulating an asynchronous operation setTimeout(() => { const data = 'Async data'; callback(data); }, 1000); }; fetchData((result) => { console.log(result); // Output: Async data });
3.2 Promises:
Promises provide a cleaner and more structured way to handle asynchronous operations. They represent the eventual completion or failure of an asynchronous operation and allow chaining multiple operations together.
// Example using Promises const fetchData = () => { return new Promise((resolve, reject) => { // Simulating an asynchronous operation setTimeout(() => { const data = 'Async data'; resolve(data); }, 1000); }); }; fetchData() .then((result) => { console.log(result); // Output: Async data }) .catch((error) => { console.error(error); });
3.3 Async/Await:
Introduced in ECMAScript 2017 (ES8), async/await provides a more synchronous style of writing asynchronous code, making it more readable and maintainable.
// Example using async/await const fetchData = async () => { return new Promise((resolve) => { // Simulating an asynchronous operation setTimeout(() => { const data = 'Async data'; resolve(data); }, 1000); }); }; const fetchDataAndProcess = async () => { const result = await fetchData(); console.log(result); // Output: Async data }; fetchDataAndProcess();
4. Benefits and Considerations
4.1 Benefits of the Event Loop and Asynchronous Programming:
- Scalability: Enables handling a large number of concurrent connections efficiently.
- Responsiveness: Facilitates real-time applications without blocking operations.
- Efficiency: Maximizes the utilization of system resources.
4.2 Considerations:
- Callback Hell: Nesting too many callbacks can lead to unreadable code. Consider using Promises or async/await to mitigate this issue.
- Error Handling: Proper error handling is crucial in asynchronous code to avoid unhandled exceptions.
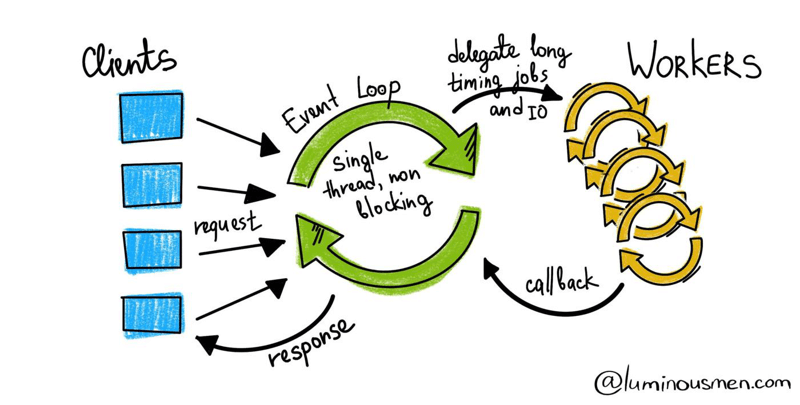
5. Conclusion
Understanding the event loop and asynchronous programming is essential for unleashing the full potential of Node.js. Leveraging its single-threaded, non-blocking architecture allows developers to create highly performant and scalable applications. With the evolution of JavaScript, the introduction of Promises and async/await has further enhanced the readability and maintainability of asynchronous code.
Embracing these concepts empowers developers to build responsive, efficient, and scalable applications that can handle the demands of modern web development. As Node.js continues to evolve, mastering these fundamental principles becomes even more crucial for developers seeking to harness the true power of asynchronous programming in their applications.