Introduction
Node.js has gained immense popularity for its efficiency and scalability in building server-side applications. However, like any technology, developers can encounter challenges and make mistakes during development. In this article, we’ll explore the top five most common mistakes in Node.js development and how to avoid them.
1. Blocking the Event Loop with Synchronous Operations
One of Node.js’s key features is its event-driven, non-blocking I/O model. However, developers sometimes inadvertently block the event loop by performing synchronous operations or using blocking functions. This can lead to poor performance and defeat the purpose of using Node.js for scalable applications.
Avoidance Strategy:
Whenever possible, favor asynchronous operations and use non-blocking functions. Leverage callbacks, Promises, or the async/await syntax to handle asynchronous code effectively. Be cautious with functions that have synchronous versions, and opt for their asynchronous counterparts.
// Blocking code const fs = require('fs'); const data = fs.readFileSync('file.txt'); // Synchronous read // Non-blocking code const fs = require('fs'); fs.readFile('file.txt', (err, data) => { if (err) throw err; // Handle data });
2. Not Handling Errors Properly
Node.js applications can encounter errors, whether due to I/O operations, network issues, or other reasons. Neglecting to handle errors appropriately can result in unhandled exceptions, crashes, and unreliable applications.
Avoidance Strategy:
Always implement error handling in your Node.js applications. Use try-catch blocks for synchronous code and error-first callbacks or Promises for asynchronous operations. Leverage tools like process.on('uncaughtException')
or third-party libraries to catch unhandled exceptions.
// Error handling with try-catch try { // Code that may throw an error } catch (error) { // Handle the error } // Error handling with callbacks fs.readFile('file.txt', (err, data) => { if (err) { console.error(err); // Handle the error } else { // Process data } });
3. Neglecting Proper Dependency Management
Managing dependencies is a crucial aspect of Node.js development. Neglecting to define and update dependencies properly can lead to compatibility issues, security vulnerabilities, and challenges in maintaining the project.
Avoidance Strategy:
Use a package.json file to specify project dependencies and versions. Regularly update dependencies to benefit from bug fixes and security patches. Consider using tools like npm audit
to identify and address security vulnerabilities in your dependencies.
// Example package.json { "name": "my-node-app", "version": "1.0.0", "dependencies": { "express": "^4.17.1", "lodash": "^4.17.21" } }
4. Ignoring Memory Leaks and Scalability
Node.js’s single-threaded event loop architecture doesn’t automatically free up memory between requests. Ignoring memory leaks and neglecting scalability considerations can lead to application crashes and degraded performance, especially in long-running processes.
Avoidance Strategy:
Regularly monitor and profile your application for memory leaks using tools like Node.js’s built-in --inspect
flag, or third-party tools like heapdump
and clinic.js
. Implement effective memory management practices, such as releasing resources and avoiding global variables.
node --inspect your-app.js
5. Using Callback Hell (Pyramid of Doom)
Nested callbacks, often referred to as “Callback Hell” or the “Pyramid of Doom,” can result in unreadable and hard-to-maintain code. This commonly occurs when handling multiple asynchronous operations sequentially.
Avoidance Strategy:
Adopt asynchronous control flow patterns to avoid callback hell. Utilize Promises, async/await syntax, or consider using libraries like async
or Bluebird
to organize and flatten your asynchronous code.
// Callback hell example fs.readFile('file1.txt', (err, data1) => { if (err) throw err; fs.readFile('file2.txt', (err, data2) => { if (err) throw err; fs.readFile('file3.txt', (err, data3) => { if (err) throw err; // Process data1, data2, and data3 }); }); }); // Using Promises to flatten the code const readFile = (file) => new Promise((resolve, reject) => { fs.readFile(file, (err, data) => { if (err) reject(err); else resolve(data); }); }); readFile('file1.txt') .then((data1) => readFile('file2.txt')) .then((data2) => readFile('file3.txt')) .then((data3) => { // Process data1, data2, and data3 }) .catch((err) => console.error(err));
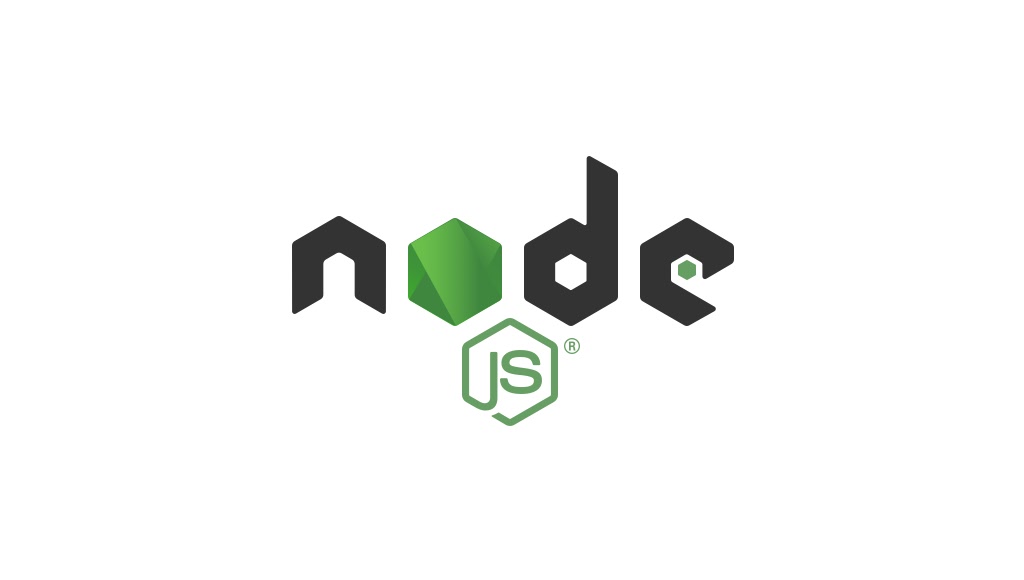
Conclusion
Node.js is a powerful platform for building scalable and efficient applications, but developers must be mindful of common mistakes that can impact performance, reliability, and maintainability. By avoiding these