Object-Oriented Programming (OOP) is a paradigm that revolutionized software development by introducing the concept of objects and their interactions. In the world of C#, OOP is a fundamental and powerful approach. In this article, we’ll explore the principles of Object-Oriented Programming and demonstrate how they are applied in C#.
Introduction to Object-Oriented Programming:
OOP is a programming paradigm based on the concept of “objects,” which can encapsulate data and behavior. Objects are instances of classes, and classes define the blueprint for creating objects. The four main principles of OOP are encapsulation, inheritance, polymorphism, and abstraction.
1. Encapsulation:
Encapsulation is the bundling of data (attributes) and methods (functions) that operate on the data into a single unit called a class. In C#, a class is a blueprint for creating objects.
Example:
public class Car { // Private fields encapsulated within the class private string make; private string model; // Public methods to access and modify the encapsulated data public void SetMake(string make) { this.make = make; } public string GetMake() { return make; } public void SetModel(string model) { this.model = model; } public string GetModel() { return model; } }
2. Inheritance:
Inheritance allows a class (subclass or derived class) to inherit the properties and behaviors of another class (base class or superclass). This promotes code reuse and establishes a hierarchy of classes.
Example:
public class Animal { public void Eat() { Console.WriteLine("Animal is eating."); } } public class Dog : Animal { public void Bark() { Console.WriteLine("Dog is barking."); } }
3. Polymorphism:
Polymorphism allows objects of different types to be treated as objects of a common base type. It enables flexibility and extensibility in code.
Example:
public class Shape { public virtual void Draw() { Console.WriteLine("Drawing a shape."); } } public class Circle : Shape { public override void Draw() { Console.WriteLine("Drawing a circle."); } } public class Square : Shape { public override void Draw() { Console.WriteLine("Drawing a square."); } }
4. Abstraction:
Abstraction involves simplifying complex systems by modeling classes based on their essential features. Abstract classes and interfaces are key components of abstraction in C#.
Example:
// Abstract class public abstract class Shape { // Abstract method (no implementation in the abstract class) public abstract void Draw(); // Regular method with implementation public void Move() { Console.WriteLine("Moving the shape."); } }
Putting it All Together:
Let’s create a practical example using OOP principles in C# to model a library system.
public abstract class LibraryItem { public string Title { get; set; } public int Year { get; set; } public abstract void DisplayInfo(); } public class Book : LibraryItem { public string Author { get; set; } public override void DisplayInfo() { Console.WriteLine($"Book: {Title} ({Year}), Author: {Author}"); } } public class DVD : LibraryItem { public string Director { get; set; } public override void DisplayInfo() { Console.WriteLine($"DVD: {Title} ({Year}), Director: {Director}"); } }
In this example, LibraryItem
is an abstract class, and Book
and DVD
are concrete classes that inherit from it. Each class encapsulates its properties and behaviors, demonstrating encapsulation, inheritance, polymorphism, and abstraction.
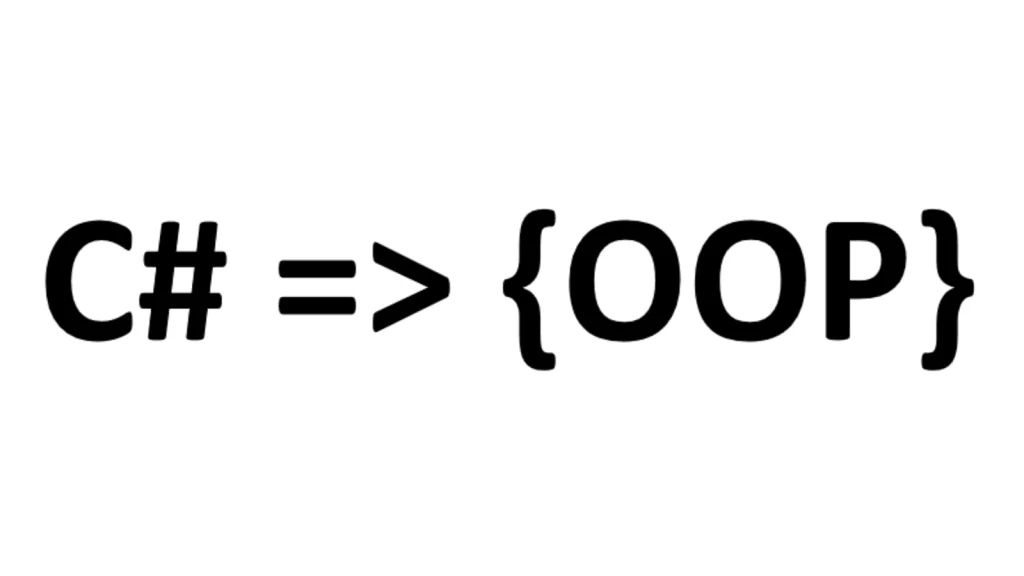
Conclusion: Building Robust Solutions with OOP in C#:
Object-Oriented Programming is a powerful paradigm that promotes modular, extensible, and maintainable code. In C#, OOP principles are seamlessly integrated, allowing developers to create well-organized and efficient applications. Whether you are designing simple classes or complex class hierarchies, understanding and applying OOP principles in C# will empower you to build robust and scalable solutions.