Introduction
Perl, the Practical Extraction and Reporting Language, stands as a testament to the enduring power of a well-designed language. Created by Larry Wall in 1987, Perl has been a versatile and influential force in the world of programming. While it might not always be the first language that comes to mind for new developers, Perl’s unique features and capabilities make it an indispensable tool for many seasoned programmers.
- Versatility and Ubiquity: Perl gained widespread popularity due to its versatility and adaptability. Originally designed for text processing, it quickly evolved into a general-purpose programming language. Its regular expression support, string manipulation capabilities, and powerful file-handling functions make it particularly well-suited for tasks such as data extraction, report generation, and system administration.
- Regular Expressions: Perl’s handling of regular expressions is unparalleled. Larry Wall, the creator of Perl, has often emphasized the importance of making easy tasks easy and difficult tasks possible. Perl’s regular expression syntax allows developers to express complex patterns concisely, making it a go-to language for tasks involving pattern matching, parsing, and data validation.
- CPAN – Comprehensive Perl Archive Network: One of Perl’s strengths lies in its extensive collection of modules and libraries available through CPAN. CPAN simplifies the process of sharing and distributing Perl modules, enabling developers to leverage a vast array of pre-built solutions for common programming challenges. This robust ecosystem has contributed to Perl’s longevity and continued relevance.
- System Administration: Perl has been a favorite among system administrators for decades. Its concise syntax and powerful text-processing capabilities make it ideal for writing scripts to automate system tasks. From log file analysis to network configuration, Perl scripts have been the backbone of countless automation solutions.
- Web Development with CGI: Perl played a crucial role in the early days of web development through the Common Gateway Interface (CGI). Many dynamic websites were powered by Perl scripts, handling user input and generating HTML dynamically. While CGI has given way to more modern web development frameworks, Perl’s influence on the evolution of the web is undeniable.
- Object-Oriented Programming: Perl supports object-oriented programming (OOP) principles, allowing developers to organize code in a modular and structured manner. While not as strictly enforced as in some other languages, Perl’s OOP capabilities provide a flexible and scalable approach to software development.
- Community and Support: The Perl community, known for its friendliness and helpfulness, has been instrumental in the language’s enduring popularity. Active forums, mailing lists, and conferences provide a platform for developers to share knowledge, discuss best practices, and collaborate on projects.
Perl’s longevity is a testament to its adaptability, expressive syntax, and the strength of its community. While it may not be as flashy or trendy as some newer languages, Perl continues to play a vital role in various domains, from system administration to data analysis. Whether you’re a seasoned developer nostalgic for the early days of the web or a newcomer exploring the rich history of programming languages, Perl’s timeless elegance and functionality make it worth a closer look.
Simple examples of Perl code to give you a glimpse of the language’s syntax and features.
Example 1: Hello, World!
#!/usr/bin/perl use strict; use warnings; print "Hello, World!\n";
This classic “Hello, World!” example demonstrates the basic structure of a Perl script. The use strict;
and use warnings;
pragmas help catch common mistakes and enforce good coding practices.
Example 2: Variables and User Input
#!/usr/bin/perl use strict; use warnings; # Declare and initialize a scalar variable my $name = "John"; # Print a greeting using the variable print "Hello, $name!\n"; # Prompt the user for input print "What is your name? "; my $user_input = <STDIN>; # Read input from the user chomp($user_input); # Remove the newline character print "Nice to meet you, $user_input!\n";
This example introduces scalar variables and user input. The <STDIN>
operator reads a line of input from the user, and chomp
is used to remove the trailing newline character.
Example 3: Conditional Statements
#!/usr/bin/perl use strict; use warnings; # Prompt the user for a number print "Enter a number: "; my $number = <STDIN>; chomp($number); # Check if the number is positive, negative, or zero if ($number > 0) { print "The number is positive.\n"; } elsif ($number < 0) { print "The number is negative.\n"; } else { print "The number is zero.\n"; }
Here, a simple conditional statement is used to determine whether a user-entered number is positive, negative, or zero.
Example 4: Looping with a for
loop
#!/usr/bin/perl use strict; use warnings; # Print numbers from 1 to 5 using a for loop for my $i (1..5) { print "$i\n"; }
This example demonstrates a basic for
loop to iterate over a range of numbers and print them.
These examples cover some fundamental aspects of Perl, including variable usage, user input, conditional statements, and loops. Feel free to experiment and explore more features of the language based on your interests and requirements.
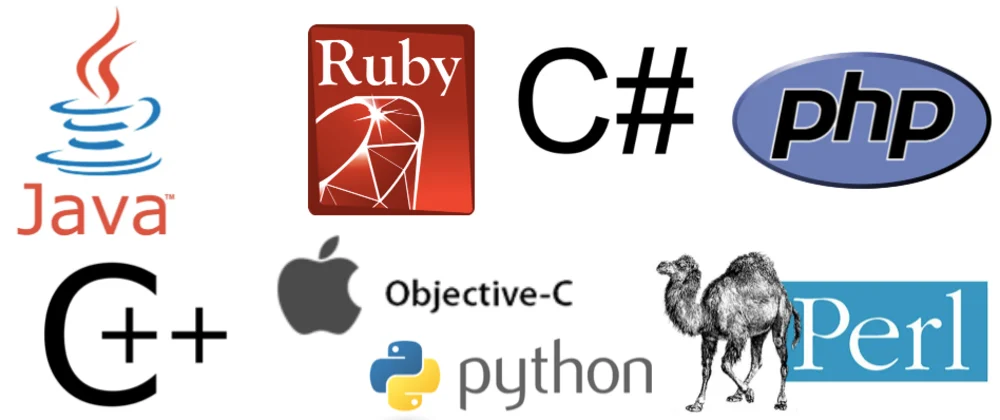
Harnessing the Power of Perl in Web Application Development
Perl, the versatile programming language renowned for its text-processing capabilities and system administration scripts, has found its way into the realm of web application development. Despite the proliferation of newer languages and frameworks, Perl continues to thrive in creating robust, scalable, and efficient web applications. In this article, we will explore the unique features of Perl that make it well-suited for web development and delve into how developers leverage its strengths to build dynamic and interactive web applications.
- CGI (Common Gateway Interface): Perl first made its mark in web development through the Common Gateway Interface (CGI). CGI allows a web server to communicate with external programs, and Perl scripts were among the earliest and most popular choices for dynamic content generation. Even though modern web development has moved beyond CGI, its historical significance showcases Perl’s adaptability to new challenges.
# Example CGI script to display a dynamic web page # (Note: Modern web development prefers frameworks over CGI) # filename: dynamic_page.cgi #!/usr/bin/perl use strict; use warnings; print "Content-type: text/html\n\n"; print "<html><head><title>Dynamic Page</title></head><body>"; print "<h1>Hello, World!</h1>"; print "<p>Current date and time: " . localtime() . "</p>"; print "</body></html>";
- Web Frameworks: While CGI laid the foundation, modern Perl web development often revolves around frameworks. Catalyst, Dancer, and Mojolicious are among the popular web frameworks that simplify the process of building web applications in Perl. These frameworks provide tools for routing, templating, and database interaction, offering a structured approach to application development.
# Example using Mojolicious framework # filename: myapp.pl use Mojolicious::Lite; get '/' => sub { my $c = shift; $c->render(text => 'Hello, Mojolicious!'); }; app->start;
- Database Connectivity: Perl seamlessly integrates with various databases, allowing developers to create web applications with robust data storage and retrieval capabilities. Database abstraction layers like DBI (Database Interface) make it easy to connect to different database systems.
# Example using DBI to connect to a SQLite database use DBI; my $dbh = DBI->connect("dbi:SQLite:dbname=test.db", "", ""); my $sth = $dbh->prepare("SELECT * FROM users"); $sth->execute(); while (my $row = $sth->fetchrow_hashref) { print "User: $row->{username}, Email: $row->{email}\n"; } $dbh->disconnect();
- Template Engines: Perl web frameworks often include template engines, simplifying the separation of code and presentation. Template Toolkit (TT) is a popular choice, enabling developers to create dynamic HTML pages with embedded Perl code.
# Example using Template Toolkit # filename: template.tt <html> <head><title>Template Example</title></head> <body> <h1>Hello, [% name %]!</h1> <p>Your email is: [% email %]</p> </body> </html>
- RESTful Web Services: Perl’s flexibility extends to building RESTful web services. Lightweight frameworks like Dancer make it easy to create APIs for applications that need to expose data or functionality to other systems.
# Example using Dancer for a simple RESTful endpoint # filename: myapp.pm package MyApp; use Dancer; get '/api/greeting/:name' => sub { my $name = route_parameters->get('name'); return { greeting => "Hello, $name!" }; }; 1;
Conclusion
Perl’s enduring legacy in web development is a testament to its adaptability and versatility. While the landscape of web application development has evolved, Perl continues to offer a reliable and efficient solution for building dynamic, database-driven applications. Whether through its historical use of CGI or modern frameworks like Mojolicious and Dancer, Perl remains a powerful choice for developers seeking a balance between expressive code and robust functionality in the world of web development.