Introduction Asynchronous
Asynchronous programming is a paradigm that allows tasks to be executed concurrently without waiting for each to complete before starting the next. This style of programming is particularly beneficial for I/O-bound operations, such as network requests or file system operations, where waiting for a response would otherwise introduce significant latency. In this article, we’ll explore asynchronous programming in PHP, including the challenges it addresses and the tools available for implementation.
Understanding Asynchronous Programming
Traditional synchronous programming involves executing tasks sequentially, one after the other. Asynchronous programming, on the other hand, enables overlapping of tasks, making better use of resources and reducing idle time. In PHP, this is achieved through non-blocking I/O operations and event-driven programming.
Challenges Addressed by Asynchronous Programming
1. Latency in I/O Operations
In web development, applications often need to perform tasks such as fetching data from a database or making API calls. Waiting for these operations to complete synchronously can introduce latency, making the application less responsive.
2. Scalability
Asynchronous programming allows servers to handle a large number of concurrent connections without the need for a separate thread or process for each connection. This scalability is crucial for handling a high volume of requests efficiently.
3. Improved Responsiveness
User interfaces in web applications benefit from asynchronous operations by remaining responsive even when performing time-consuming tasks in the background.
Implementing Asynchronous Programming in PHP
1. Callbacks
One way to perform asynchronous operations in PHP is through callbacks. Callbacks are functions passed as arguments to other functions, and they allow the execution of code after a specific event or operation.
Example:
<?php function fetchData($url, $callback) { // Simulate asynchronous data fetching // In a real-world scenario, this could be an HTTP request or database query usleep(2000000); // Simulate a delay of 2 seconds // Callback with the fetched data $data = "Data fetched from $url"; $callback($data); } // Asynchronous call fetchData('https://example.com', function($data) { echo $data . "\n"; }); echo "Code after asynchronous call.\n"; ?>
2. Promises
Promises provide a cleaner way to work with asynchronous code by representing a value that might be available now, or in the future, or never. Libraries like ReactPHP and Guzzle promise implementations to facilitate asynchronous operations.
Example using ReactPHP:
<?php require 'vendor/autoload.php'; use React\Promise\Promise; function fetchDataAsync($url): Promise { return new Promise(function ($resolve) use ($url) { // Simulate asynchronous data fetching // In a real-world scenario, this could be an HTTP request or database query usleep(2000000); // Simulate a delay of 2 seconds // Resolve the promise with the fetched data $data = "Data fetched from $url"; $resolve($data); }); } // Asynchronous call fetchDataAsync('https://example.com')->then(function ($data) { echo $data . "\n"; }); echo "Code after asynchronous call.\n";
3. Async/Await with Amp
Amp is a library that brings asynchronous programming and coroutines to PHP. It provides an async/await
syntax similar to other languages like JavaScript.
Example using Amp:
<?php require 'vendor/autoload.php'; use Amp\Loop; use Amp\Promise; function fetchDataAsync($url): Promise { return Amp\call(function () use ($url) { // Simulate asynchronous data fetching // In a real-world scenario, this could be an HTTP request or database query yield Amp\delay(2000); // Simulate a delay of 2 seconds // Return the fetched data return "Data fetched from $url"; }); } // Asynchronous call Loop::run(function () { $data = yield fetchDataAsync('https://example.com'); echo $data . "\n"; }); echo "Code after asynchronous call.\n";
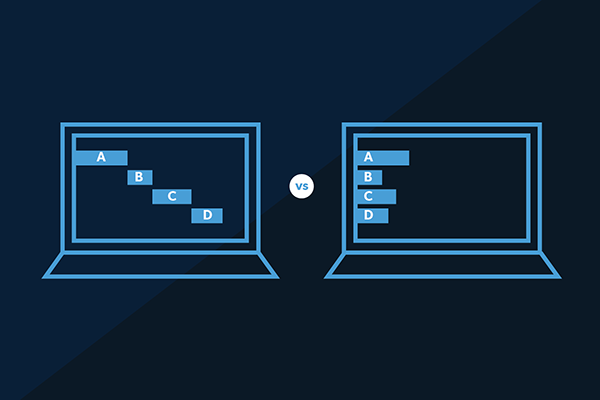
Conclusion
Asynchronous programming in PHP has evolved, providing developers with various tools and libraries to handle concurrency effectively. Whether using traditional callbacks, promises, or the modern async/await syntax, PHP developers can leverage these techniques to build responsive and scalable applications. Understanding the principles and choosing the right approach for your specific use case will empower you to harness the benefits of asynchronous programming in PHP.