Introduction
Python boasts a variety of powerful frameworks that cater to different application domains. Here are articles on some popular Python frameworks:
1.Django: A Comprehensive Guide to Web Development
- An in-depth look at Django, a high-level web framework that follows the model-view-controller (MVC) pattern. Covers project setup, creating models, views, and templates, and handling URLs.
2. Flask: Building Web Applications with Simplicity
- Exploring Flask, a lightweight web framework that emphasizes simplicity and flexibility. Covers routing, templates, and creating RESTful APIs with Flask.
3. FastAPI: Modern, Fast, and Web APIs with Python
- An introduction to FastAPI, a modern, fast web framework for building APIs with Python. Explores its automatic interactive documentation and dependency injection features.
4. Pyramid: Flexible and Modular Web Applications
- Delving into Pyramid, a full-featured web framework known for its flexibility and modularity. Covers URL dispatch, views, and building scalable applications.
5. Tornado: Asynchronous Web Framework for Python
- Exploring Tornado, an asynchronous web framework that excels in handling long-lived connections. Covers asynchronous programming and building real-time applications.
6. Flask-RESTful: Building REST APIs with Flask
- Focusing on Flask-RESTful, an extension for Flask that simplifies the creation of RESTful APIs. Covers resource creation, request parsing, and authentication.
7. Dash: Building Interactive Web Applications with Python
- An overview of Dash, a framework for building interactive web applications with Python. Covers creating dashboards, handling callbacks, and integrating data visualizations.
8. Scrapy: Web Scraping with Python
- A guide to Scrapy, a powerful and extensible framework for extracting data from websites. Covers crawling, spider creation, and data extraction.
9. Celery: Distributed Task Queue for Python
- Exploring Celery, a distributed task queue framework for handling asynchronous tasks in Python. Covers task creation, scheduling, and integrating with different backends.
10. PyQt and Tkinter: GUI Development with Python
- Comparing PyQt and Tkinter, two popular frameworks for building graphical user interfaces (GUIs) in Python. Covers widget creation, event handling, and designing user interfaces.
Whether you’re interested in web development, data extraction, or building GUI applications, Python offers a diverse range of frameworks to suit your needs.
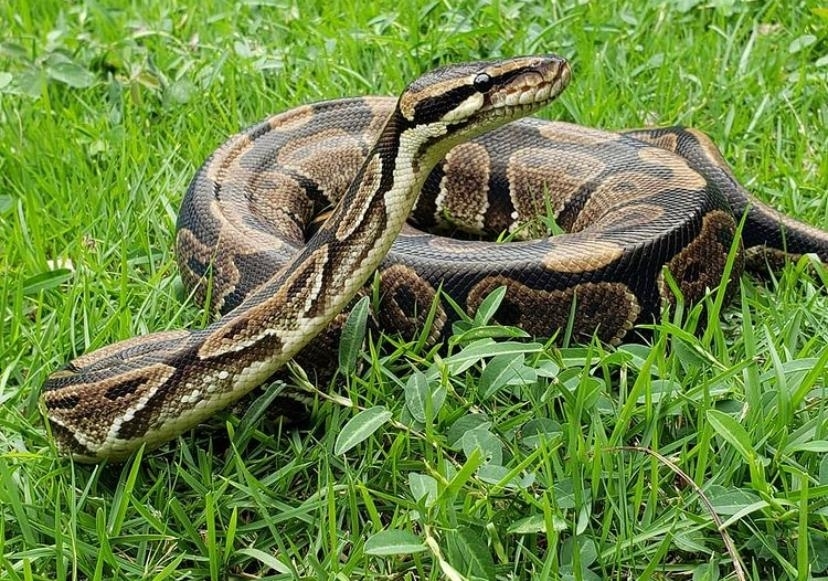
Certainly! Here are brief sample codes to give you a taste of each mentioned framework:
- Django:
# models.py from django.db import models class Post(models.Model): title = models.CharField(max_length=200) content = models.TextField() # views.py from django.shortcuts import render def post_list(request): posts = Post.objects.all() return render(request, 'blog/post_list.html', {'posts': posts})
- Flask:
from flask import Flask, render_template app = Flask(__name__) @app.route('/') def index(): return render_template('index.html') if __name__ == '__main__': app.run(debug=True)
- FastAPI:
from fastapi import FastAPI app = FastAPI() @app.get("/") def read_root(): return {"Hello": "World"}
- Pyramid:
from pyramid.config import Configurator from pyramid.response import Response def hello_world(request): return Response('Hello, World!') config = Configurator() config.add_route('hello', '/') config.add_view(hello_world, route_name='hello') app = config.make_wsgi_app()
- Tornado:
import tornado.ioloop import tornado.web class MainHandler(tornado.web.RequestHandler): def get(self): self.write("Hello, World!") app = tornado.web.Application([(r'/', MainHandler)]) app.listen(8888) tornado.ioloop.IOLoop.current().start()
Certainly! Here are sample codes for frameworks 6 to 10:
- Flask-RESTful:
from flask import Flask from flask_restful import Resource, Api app = Flask(__name__) api = Api(app) class HelloWorld(Resource): def get(self): return {'hello': 'world'} api.add_resource(HelloWorld, '/') if __name__ == '__main__': app.run(debug=True)
- Dash:
import dash from dash import dcc, html app = dash.Dash(__name__) app.layout = html.Div(children=[ html.H1(children='Hello Dash'), dcc.Graph( id='example-graph', figure={ 'data': [ {'x': [1, 2, 3], 'y': [4, 1, 2], 'type': 'bar', 'name': 'SF'}, ], 'layout': { 'title': 'Dash Data Visualization' } } ) ]) if __name__ == '__main__': app.run_server(debug=True)
- Scrapy:
import scrapy class MySpider(scrapy.Spider): name = 'my_spider' start_urls = ['http://example.com'] def parse(self, response): self.log('Visited %s' % response.url) # Your scraping logic here
- Celery:
from celery import Celery app = Celery('tasks', broker='pyamqp://guest@localhost//') @app.task def add(x, y): return x + y
- PyQt and Tkinter (Simple GUI):
# PyQt from PyQt5.QtWidgets import QApplication, QLabel app = QApplication([]) label = QLabel('Hello, World!') label.show() app.exec_() # Tkinter import tkinter as tk root = tk.Tk() label = tk.Label(root, text='Hello, World!') label.pack() root.mainloop()
These snippets provide a glimpse into the structure and simplicity of each framework. For more comprehensive understanding, it’s recommended to refer to the respective framework’s documentation and tutorials.