Certainly! Here’s a basic example of how you might create a simple API endpoint for each of the mentioned PHP frameworks: Laravel, Symfony, CodeIgniter, Yii, and Phalcon. We’ll focus on a RESTful API endpoint for retrieving data.
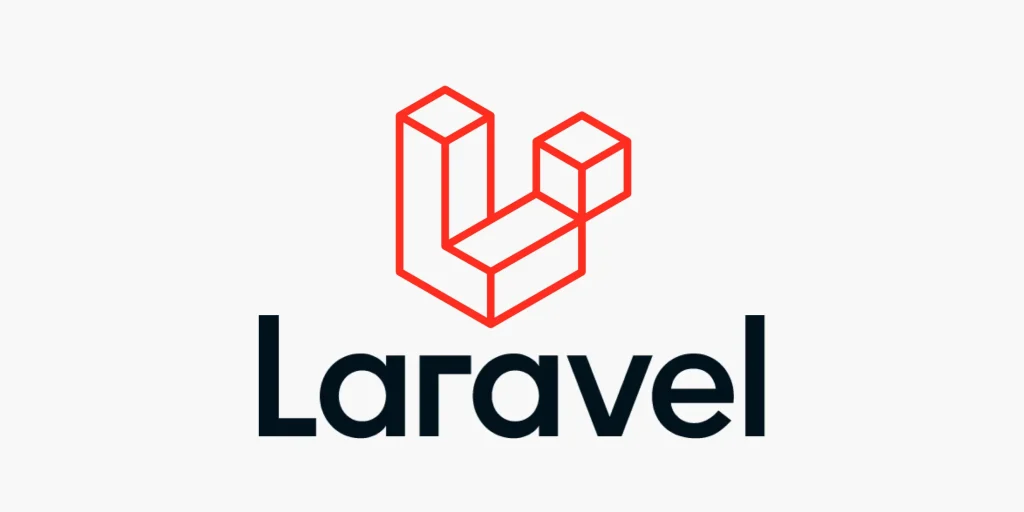
1. Laravel:
// Laravel - API endpoint for retrieving data Route::get('/api/data', 'ApiController@getData');
In Laravel, this route could be part of the routes/api.php
file, pointing to a controller method (getData
) that handles the API request.
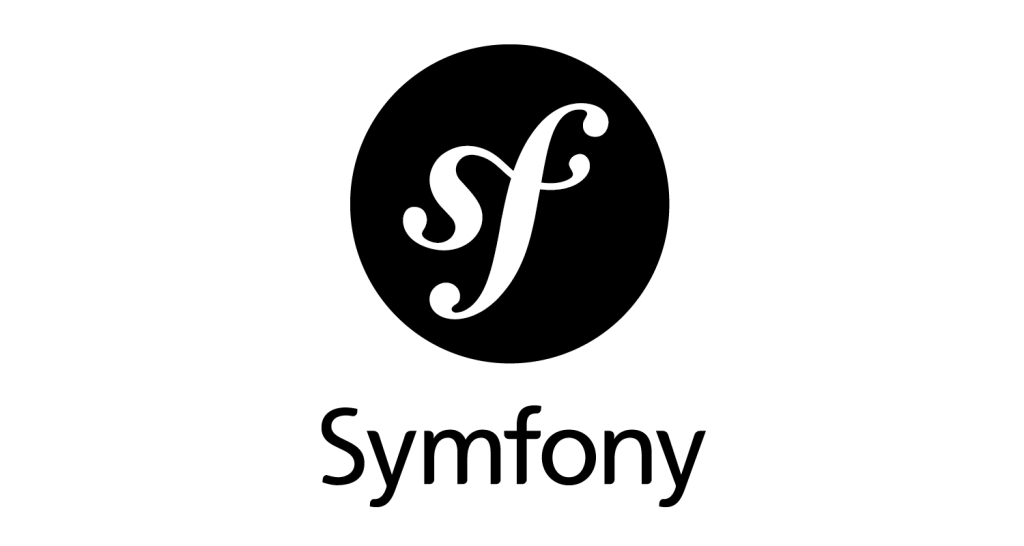
2. Symfony:
// Symfony - API endpoint for retrieving data /** * @Route("/api/data", name="api_get_data", methods={"GET"}) */ public function getData() { // Logic to retrieve and return data in JSON format }
In Symfony, this example uses annotations to define a route for an API endpoint that returns data in JSON format.
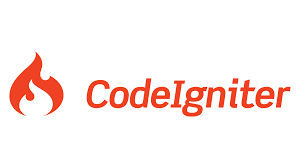
3. CodeIgniter:
// CodeIgniter - API endpoint for retrieving data public function getData() { // Logic to retrieve and return data in JSON format }
In CodeIgniter, this method could be part of a controller handling API requests, returning data in JSON format.
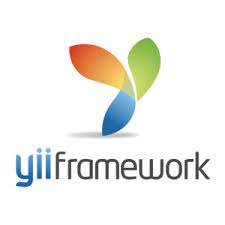
4. Yii:
// Yii - API endpoint for retrieving data public function actionGetData() { // Logic to retrieve and return data in JSON format }
In Yii, the action
prefix is used for controller actions. This example represents an action for retrieving data in JSON format.
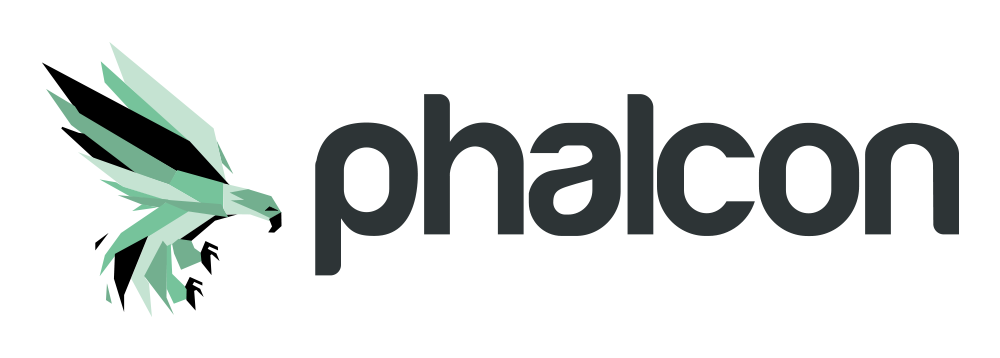
5. Phalcon:
// Phalcon - API endpoint for retrieving data public function getDataAction() { // Logic to retrieve and return data in JSON format }
In Phalcon, the Action
suffix is a convention for controller actions. This method handles the API request for retrieving data.
These examples focus on the route and controller method or action responsible for handling the API request. In a real-world scenario, you would likely integrate these with business logic, models, and potentially authentication mechanisms based on your specific API requirements.