Introduction:
Integrating a database into your Golang application is a fundamental aspect of web development. In this article, we’ll explore how to implement CRUD (Create, Read, Update, Delete) operations with some of the top Golang frameworks: Gin, Echo, Revel, Buffalo, and Fiber. We’ll provide sample code snippets to demonstrate how each framework handles database interactions.
- Gin: Sample Code for CRUD with Gin and Gorm (ORM):
package main import ( "github.com/gin-gonic/gin" "gorm.io/gorm" "gorm.io/driver/sqlite" ) type User struct { gorm.Model Name string Email string } func main() { db, _ := gorm.Open(sqlite.Open("test.db"), &gorm.Config{}) db.AutoMigrate(&User{}) router := gin.Default() router.GET("/users", func(c *gin.Context) { var users []User db.Find(&users) c.JSON(200, users) }) // Implement other CRUD routes... router.Run(":8080") }
- Echo: Sample Code for CRUD with Echo and Gorm:
package main import ( "github.com/labstack/echo/v4" "gorm.io/gorm" "gorm.io/driver/sqlite" ) type User struct { gorm.Model Name string Email string } func main() { db, _ := gorm.Open(sqlite.Open("test.db"), &gorm.Config{}) db.AutoMigrate(&User{}) e := echo.New() e.GET("/users", func(c echo.Context) error { var users []User db.Find(&users) return c.JSON(200, users) }) // Implement other CRUD routes... e.Start(":8080") }
- Revel: Sample Code for CRUD with Revel and Gorm:
package controllers import ( "github.com/revel/revel" "gorm.io/gorm" "gorm.io/driver/sqlite" ) type App struct { *revel.Controller } type User struct { gorm.Model Name string Email string } func (c App) ListUsers() revel.Result { var users []User db.Find(&users) return c.RenderJSON(users) } // Implement other CRUD actions...
- Buffalo: Sample Code for CRUD with Buffalo and Pop (ORM):
package actions import ( "github.com/gobuffalo/buffalo" "github.com/gobuffalo/pop" ) type User struct { pop.Model Name string Email string } func ListUsers(c buffalo.Context) error { tx := c.Value("tx").(*pop.Connection) users := []User{} tx.All(&users) return c.Render(200, r.JSON(users)) } // Implement other CRUD actions...
- Fiber: Sample Code for CRUD with Fiber and Gorm:
package main import ( "github.com/gofiber/fiber/v2" "gorm.io/gorm" "gorm.io/driver/sqlite" ) type User struct { gorm.Model Name string Email string } func main() { db, _ := gorm.Open(sqlite.Open("test.db"), &gorm.Config{}) db.AutoMigrate(&User{}) app := fiber.New() app.Get("/users", func(c *fiber.Ctx) error { var users []User db.Find(&users) return c.JSON(users) }) // Implement other CRUD routes... app.Listen(":8080") }
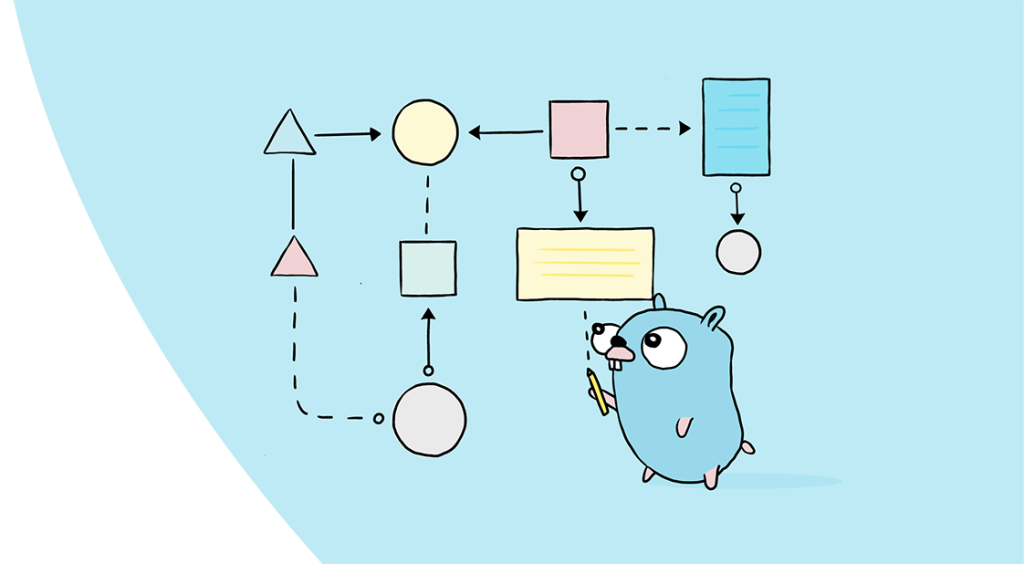
Conclusion:
These sample code snippets demonstrate how to integrate CRUD operations with popular Golang frameworks. Whether you choose Gin, Echo, Revel, Buffalo, or Fiber, each framework provides mechanisms for interacting with databases, making it easier to build robust and scalable applications. Tailor your choice based on your project’s requirements, the framework’s features, and your familiarity with its syntax and conventions. Happy coding!