Introduction:
User authentication is a fundamental aspect of web development, and JSON-based authentication is a common method to secure user access. In this article, we’ll explore how to implement user login functionality using JSON-based authentication in five popular Golang frameworks: Gin, Echo, Revel, Buffalo, and Fiber. We’ll provide sample code snippets to guide you through the implementation process.
- Gin: Sample Code for JSON-Based Authentication with Gin:
package main import ( "github.com/gin-gonic/gin" "gorm.io/gorm" "gorm.io/driver/sqlite" ) type User struct { gorm.Model Username string `json:"username"` Password string `json:"password"` } func main() { db, _ := gorm.Open(sqlite.Open("test.db"), &gorm.Config{}) db.AutoMigrate(&User{}) router := gin.Default() router.POST("/login", func(c *gin.Context) { var userInput User if err := c.BindJSON(&userInput); err != nil { c.JSON(400, gin.H{"error": "Invalid JSON"}) return } var storedUser User if err := db.Where("username = ?", userInput.Username).First(&storedUser).Error; err != nil { c.JSON(401, gin.H{"error": "Invalid credentials"}) return } // Compare passwords, implement your authentication logic here... c.JSON(200, gin.H{"message": "Login successful"}) }) // Implement other routes... router.Run(":8080") }
- Echo: Sample Code for JSON-Based Authentication with Echo:
package main import ( "github.com/labstack/echo/v4" "gorm.io/gorm" "gorm.io/driver/sqlite" ) type User struct { gorm.Model Username string `json:"username"` Password string `json:"password"` } func main() { db, _ := gorm.Open(sqlite.Open("test.db"), &gorm.Config{}) db.AutoMigrate(&User{}) e := echo.New() e.POST("/login", func(c echo.Context) error { var userInput User if err := c.Bind(&userInput); err != nil { return c.JSON(400, map[string]string{"error": "Invalid JSON"}) } var storedUser User if err := db.Where("username = ?", userInput.Username).First(&storedUser).Error; err != nil { return c.JSON(401, map[string]string{"error": "Invalid credentials"}) } // Compare passwords, implement your authentication logic here... return c.JSON(200, map[string]string{"message": "Login successful"}) }) // Implement other routes... e.Start(":8080") }
- Revel: Sample Code for JSON-Based Authentication with Revel:
package controllers import ( "github.com/revel/revel" "gorm.io/gorm" "gorm.io/driver/sqlite" ) type User struct { gorm.Model Username string `json:"username"` Password string `json:"password"` } func (c App) Login() revel.Result { var userInput User if err := c.Params.BindJSON(&userInput); err != nil { return c.RenderJSON(map[string]string{"error": "Invalid JSON"}) } var storedUser User if err := db.Where("username = ?", userInput.Username).First(&storedUser).Error; err != nil { return c.RenderJSON(map[string]string{"error": "Invalid credentials"}) } // Compare passwords, implement your authentication logic here... return c.RenderJSON(map[string]string{"message": "Login successful"}) }
- Buffalo: Sample Code for JSON-Based Authentication with Buffalo:
package actions import ( "github.com/gobuffalo/buffalo" "github.com/gobuffalo/pop" ) type User struct { pop.Model Username string `json:"username"` Password string `json:"password"` } func Login(c buffalo.Context) error { var userInput User if err := c.BindJSON(&userInput); err != nil { return c.Render(400, r.JSON(map[string]string{"error": "Invalid JSON"})) } var storedUser User if err := tx.Where("username = ?", userInput.Username).First(&storedUser).Error; err != nil { return c.Render(401, r.JSON(map[string]string{"error": "Invalid credentials"})) } // Compare passwords, implement your authentication logic here... return c.Render(200, r.JSON(map[string]string{"message": "Login successful"})) }
- Fiber: Sample Code for JSON-Based Authentication with Fiber:
package main import ( "github.com/gofiber/fiber/v2" "gorm.io/gorm" "gorm.io/driver/sqlite" ) type User struct { gorm.Model Username string `json:"username"` Password string `json:"password"` } func main() { db, _ := gorm.Open(sqlite.Open("test.db"), &gorm.Config{}) db.AutoMigrate(&User{}) app := fiber.New() app.Post("/login", func(c *fiber.Ctx) error { var userInput User if err := c.BodyParser(&userInput); err != nil { return c.Status(400).JSON(map[string]string{"error": "Invalid JSON"}) } var storedUser User if err := db.Where("username = ?", userInput.Username).First(&storedUser).Error; err != nil { return c.Status(401).JSON(map[string]string{"error": "Invalid credentials"}) } // Compare passwords, implement your authentication logic here... return c.JSON(map[string]string{"message": "Login successful"}) }) // Implement other routes... app.Listen(":8080")
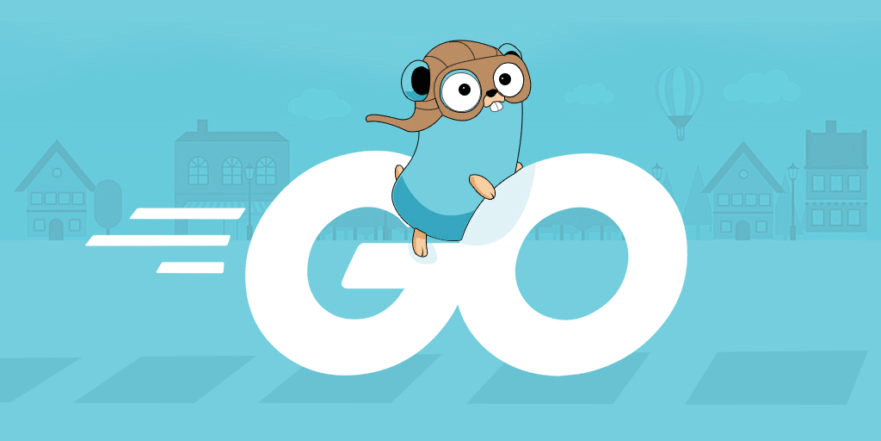
Conclusion:
Integrating JSON-based user authentication in your Golang application is a crucial step toward securing your endpoints. Whether you choose Gin, Echo, Revel, Buffalo, or Fiber, the provided sample code snippets demonstrate how to handle user login functionality. Customize these examples according to your specific authentication logic and project requirements. Always ensure secure password handling, such as using hashed passwords and proper encryption techniques. Happy coding!