Introduction
Node.js, often simply referred to as Node, has become a fundamental player in the world of web development. It’s not just a framework or library; it’s a runtime environment that allows developers to execute JavaScript code server-side. Let’s explore what Node.js is, its key features, and its impact on modern web development.
Introduction to Node.js
Node.js was created by Ryan Dahl in 2009 and is built on the V8 JavaScript runtime, the same engine that powers the Google Chrome browser. Unlike traditional JavaScript, which runs in the browser, Node.js allows developers to run JavaScript on the server side. This shift in perspective has significant implications for web development.
Key Features of Node.js
1. Asynchronous I/O
One of the standout features of Node.js is its event-driven, non-blocking I/O model. This means that instead of waiting for operations to complete before moving on, Node.js uses callbacks to signal the completion of a task. This asynchronous nature makes it well-suited for handling concurrent connections and scalable applications.
2. Single-threaded Event Loop
Node.js operates on a single-threaded event loop, yet it can handle a large number of simultaneous connections. This is achieved through non-blocking I/O operations, making Node.js highly performant, especially in scenarios with high concurrency.
3. NPM (Node Package Manager)
Node.js comes bundled with npm, a powerful package manager that simplifies the process of installing, managing, and sharing third-party libraries and tools. This has contributed to the vibrant Node.js ecosystem, with a wealth of open-source packages available for developers.
4. Cross-platform Compatibility
Node.js is cross-platform and can be run on various operating systems, including Windows, macOS, and Linux. This ensures consistency in development environments and facilitates easy deployment across different platforms.
Use Cases of Node.js
1. Web Servers
Node.js is commonly used to build scalable and high-performance web servers. Its non-blocking I/O allows handling a large number of simultaneous connections efficiently, making it an excellent choice for real-time applications.
2. API Development
With its lightweight and fast nature, Node.js is well-suited for building RESTful APIs. Many developers choose Node.js for creating the backend of web applications due to its efficiency in handling JSON data and its simplicity in building APIs.
3. Real-time Applications
Node.js shines in real-time applications like chat applications, online gaming, and collaborative tools. Its event-driven architecture enables real-time communication between the server and clients, providing a seamless user experience.
4. Microservices Architecture
Node.js is often used in microservices architectures, where modular and independent services communicate with each other. Its scalability and efficiency make it an ideal choice for building microservices-based applications.
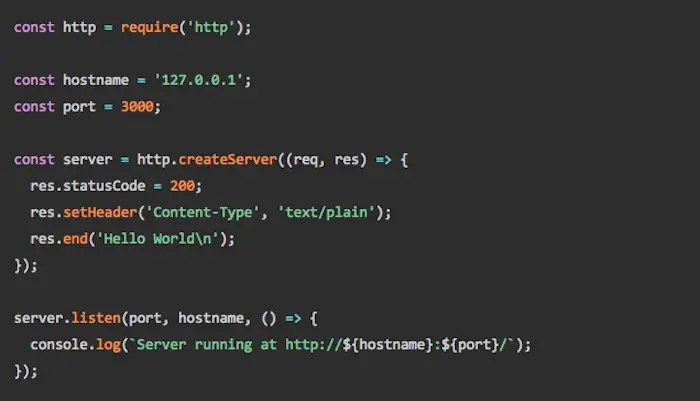
Simple Code of a Node.js
With server using the Express framework, one of the popular frameworks for building web applications with Node.js:
// Import required modules const express = require('express'); const app = express(); const port = 3000; // Define a route for the root URL app.get('/', (req, res) => { res.send('Hello, World! This is a simple Node.js server.'); }); // Start the server on the specified port app.listen(port, () => { console.log(`Server listening at http://localhost:${port}`); });
In this example:
- We import the
express
module, which is a fast, unopinionated, minimalist web framework for Node.js. - We create an instance of the Express application.
- We define a route for the root URL (
'/'
). When a user accesses the root URL, the server responds with the message “Hello, World!” - The server listens on port 3000, and once it’s running, a message is logged to the console.
To run this code, you’ll need to have Node.js installed on your machine. Save the code in a file (e.g., app.js
) and run the following commands in your terminal:
npm init -y # Initializes a new Node.js project (creates a package.json file) npm install express # Installs the Express framework node app.js # Runs the Node.js server
Now, if you open your browser and navigate to http://localhost:3000
, you should see the “Hello, World!” message. This is a basic example, but it demonstrates the simplicity and expressiveness of Node.js for building web applications.
Conclusion
Node.js has revolutionized the way developers approach server-side development with its asynchronous, event-driven architecture. Its popularity continues to grow, fueled by its performance, scalability, and a vibrant community. As web development evolves, Node.js remains a powerful tool in the arsenal of developers, driving innovation and efficiency in building modern web applications.