Introduction
Node.js has gained widespread adoption in the realm of server-side development due to its event-driven architecture and non-blocking I/O model. These characteristics make it well-suited for building scalable and efficient applications. In this article, we’ll delve into the concepts of event-driven architecture and non-blocking I/O in Node.js, exploring how they contribute to the platform’s success.
Understanding Event-Driven Architecture
At its core, event-driven architecture revolves around the concept of events and event handlers. In a traditional synchronous program, the flow of execution is determined by the sequence of statements, and one operation must complete before the next begins. However, in an event-driven model, the flow is determined by events that occur asynchronously.
In Node.js, events are a fundamental part of the system. The Event Emitter module allows objects to emit named events that cause Function objects (listeners) to be invoked. This decoupling of components through events enables a more modular and flexible architecture.
Example of Event-Driven Programming in Node.js:
const EventEmitter = require('events'); // Create an event emitter instance const myEmitter = new EventEmitter(); // Define an event handler const myEventHandler = () => { console.log('Event occurred!'); }; // Attach the event handler to the event myEmitter.on('myEvent', myEventHandler); // Emit the event myEmitter.emit('myEvent');
In this example, when the event named ‘myEvent’ is emitted, the associated event handler (myEventHandler
) is executed.
Non-blocking I/O in Node.js
Node.js is built on the V8 JavaScript runtime and employs a non-blocking I/O model. This means that while I/O operations are being executed, the program doesn’t wait for their completion. Instead, it continues to execute other tasks, making efficient use of resources.
Traditionally, with synchronous (blocking) I/O, if one operation takes time (e.g., reading from a file or making a network request), the entire program is blocked until that operation completes. In contrast, Node.js allows developers to perform I/O operations asynchronously, making it possible to handle a large number of concurrent connections without creating a new thread for each.
Example of Non-blocking I/O in Node.js:
const fs = require('fs'); // Asynchronous file read fs.readFile('example.txt', 'utf8', (err, data) => { if (err) { console.error(err); return; } console.log('File content:', data); }); console.log('Reading file asynchronously...');
In this example, the readFile
function initiates the file read operation and provides a callback function that will be executed once the operation is completed. Meanwhile, the program continues to execute the console.log
statement, showcasing non-blocking behavior.
Advantages of Event-Driven Architecture and Non-blocking I/O in Node.js
- Scalability: Node.js can handle a large number of concurrent connections due to its non-blocking I/O. This makes it particularly suitable for applications requiring high scalability, such as real-time applications and microservices.
- Efficiency: The asynchronous nature of Node.js allows for more efficient use of resources. While waiting for one operation to complete, Node.js can execute other tasks, preventing unnecessary delays.
- Real-time Capabilities: Event-driven architecture is well-suited for real-time applications like chat applications, online gaming, and collaborative tools. The ability to respond to events in real-time enhances user experience.
- Modularity: Event-driven architecture encourages a modular and decoupled design. Components can communicate through events, making the codebase more maintainable and extensible.
- Developer Productivity: Non-blocking I/O simplifies the development of scalable applications by eliminating the need for complex threading mechanisms. Developers can focus on writing asynchronous code, leading to more streamlined and readable code.
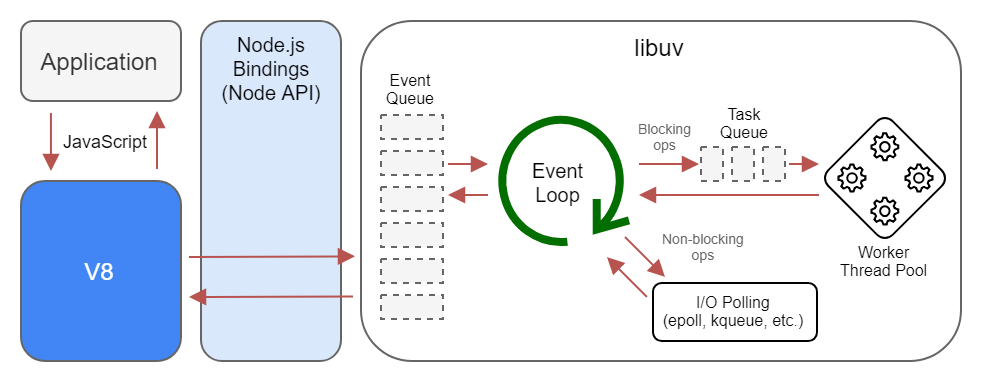
Conclusion
Node.js’s event-driven architecture and non-blocking I/O are fundamental aspects of its design that contribute to its success in building scalable and efficient applications. By embracing asynchronous programming and event-driven paradigms, Node.js provides a powerful platform for developing real-time applications and handling a large number of concurrent connections with ease. Understanding and leveraging these concepts are key to harnessing the full potential of Node.js in modern web development.