Java’s portability, versatility, strong community support, and features such as object-oriented design, security, and automatic memory management make it a vital programming language for a wide range of applications and industries.
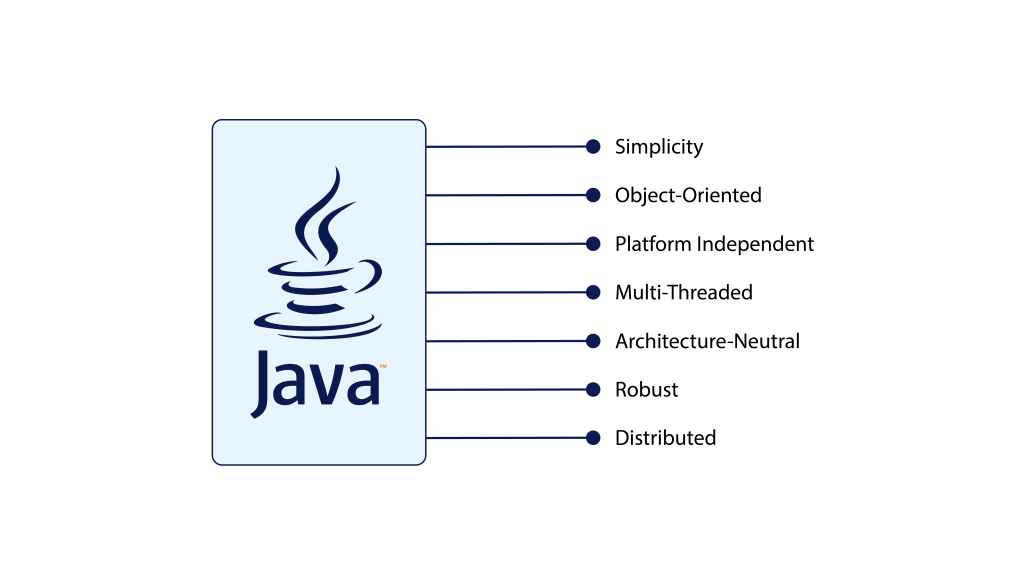
Java programming is essential for several reasons:
- Platform Independence: Java’s “write once, run anywhere” principle allows developers to create applications that can run on any device with a Java Virtual Machine (JVM). This platform independence is crucial for reaching a wide audience and simplifies software deployment.
- Versatility: Java is used in a variety of domains, including web development, enterprise applications, mobile app development (Android), scientific and research projects, embedded systems, and more. Its versatility makes it applicable to a broad range of development scenarios.
- Object-Oriented Approach: Java’s object-oriented programming (OOP) paradigm promotes modular and reusable code, making it easier to design, develop, and maintain complex software systems. OOP principles like encapsulation, inheritance, and polymorphism contribute to code organization and maintainability.
- Community and Ecosystem: Java has a vast and active developer community. This results in a rich ecosystem of libraries, frameworks, and tools that simplify and accelerate development. Developers can leverage existing resources and collaborate on open-source projects.
- Enterprise-Level Applications: Java is widely used in building large-scale, enterprise-level applications due to its scalability, reliability, and robustness. Many business-critical systems, including banking, healthcare, and e-commerce applications, rely on Java.
- Web Development: Java supports various technologies for web development, such as JavaServer Pages (JSP) and Servlets. Frameworks like Spring and Struts further enhance web application development, making it efficient and maintainable.
- Mobile Development with Android: Java is the primary programming language for Android app development. Its use in the Android ecosystem has led to a massive number of mobile applications written in Java.
- Multithreading and Concurrency: Java’s built-in support for multithreading enables developers to create concurrent applications. This is particularly valuable for performance optimization, responsiveness, and efficient resource utilization.
- Security Features: Java includes built-in security features that help protect systems from various security threats. The design of the language incorporates measures to prevent unauthorized access and execution of malicious code.
- Automatic Memory Management: Java’s garbage collector automates memory management, reducing the risk of memory leaks and making it easier for developers to focus on application logic rather than manual memory handling.
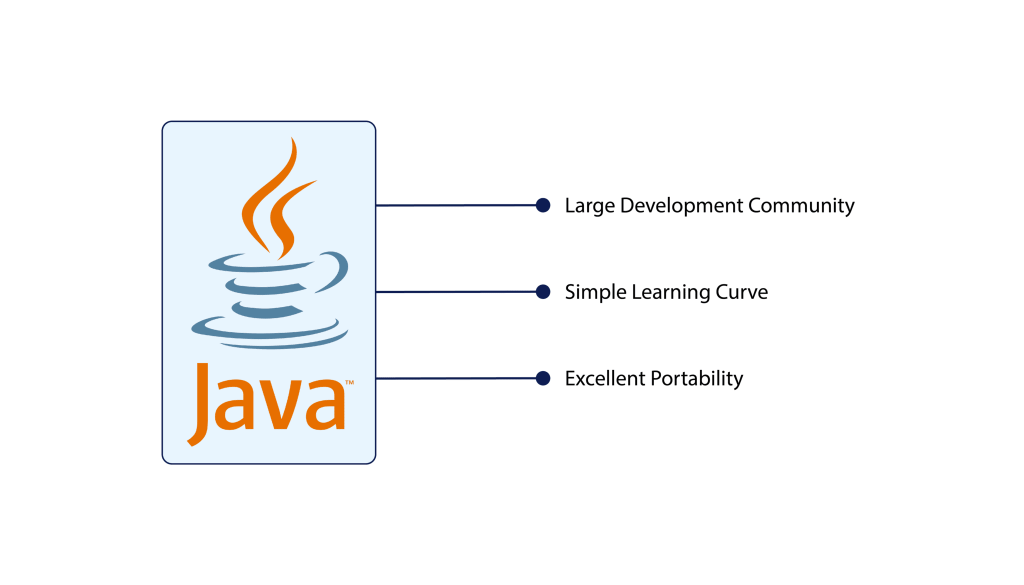
Here’s a simple example of a Java SE program.
This program prompts the user to enter their name and then prints a personalized greeting:
import java.util.Scanner;
public class GreetingProgram {
public static void main(String[] args) {
// Create a Scanner object to read input from the console
Scanner scanner = new Scanner(System.in);
// Prompt the user to enter their name
System.out.print("Enter your name: ");
// Read the user's input
String userName = scanner.nextLine();
// Close the scanner to avoid resource leaks
scanner.close();
// Print a personalized greeting
System.out.println("Hello, " + userName + "! Welcome to the Java SE Greeting Program.");
}
}
In this example:
- The
Scanner
class is used to read input from the console. It’s part of thejava.util
package. - The
main
method is the entry point of the program. - The program prompts the user to enter their name using
System.out.print
and reads the input withscanner.nextLine()
. - The entered name is stored in the
userName
variable. - The program then prints a personalized greeting to the console.
To run this program, you can compile the code using a Java compiler (e.g., javac
) and then execute it using the Java Virtual Machine (e.g., java
):
javac GreetingProgram.java
java GreetingProgram
Upon execution, the program will wait for user input, and after entering a name, it will print a personalized greeting message.