Introduction to Java EE
Java EE’s features such as scalability, security, transaction management, component-based development, connectivity, web services support, standardization, JMS, and management tools make it a preferred choice for building robust and scalable enterprise applications.
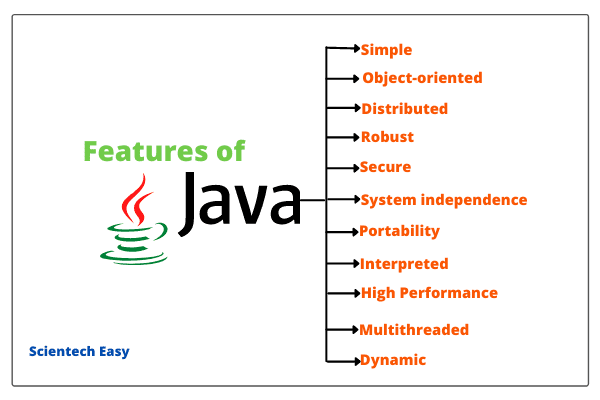
Enterprise development often uses Java EE (Enterprise Edition) for several reasons:
- Scalability: Java EE is designed to handle large-scale, enterprise-level applications. It provides features like load balancing, clustering, and distributed computing, making it well-suited for applications that need to scale horizontally to meet increasing demands.
- Security: Java EE includes robust security mechanisms, such as role-based access control, encryption, and authentication. This is crucial for enterprise applications dealing with sensitive data and transactions.
- Transaction Management: Java EE provides a sophisticated and standardized approach to transaction management. This ensures that complex business processes involving multiple operations maintain data consistency and integrity, even in the case of failures.
- Component-Based Development: Java EE supports component-based development through technologies like Enterprise JavaBeans (EJB), which allows developers to create modular and reusable components. This promotes a more efficient and maintainable codebase, especially in large-scale applications.
- Connectivity: Java EE facilitates integration with various databases, messaging systems, and other enterprise technologies. It supports the Java Connector Architecture (JCA), enabling seamless communication with different backend systems.
- Web Services Support: Java EE provides built-in support for creating and consuming web services, making it easier to integrate and communicate with other applications and services in an enterprise environment.
- Standardization: Java EE is a set of standardized specifications, ensuring consistency and compatibility across different implementations. This standardization promotes interoperability and allows developers to build portable applications that can run on different Java EE-compliant servers.
- Java Message Service (JMS): For asynchronous communication between components, Java EE includes JMS, which is essential for building scalable and loosely coupled systems in enterprise applications.
- Management and Monitoring: Java EE offers tools and APIs for managing and monitoring applications. This includes features for performance monitoring, logging, and debugging, which are crucial for maintaining the health and reliability of enterprise systems.
- Community and Ecosystem: Java EE has a large and active community of developers, along with a well-established ecosystem of libraries, frameworks, and tools. This support network is valuable for enterprise developers seeking solutions to common challenges and staying updated on best practices.
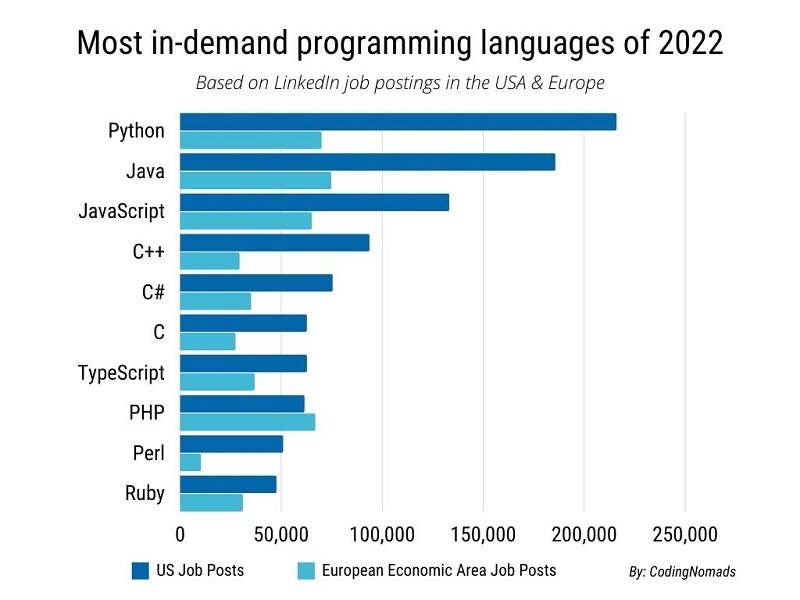
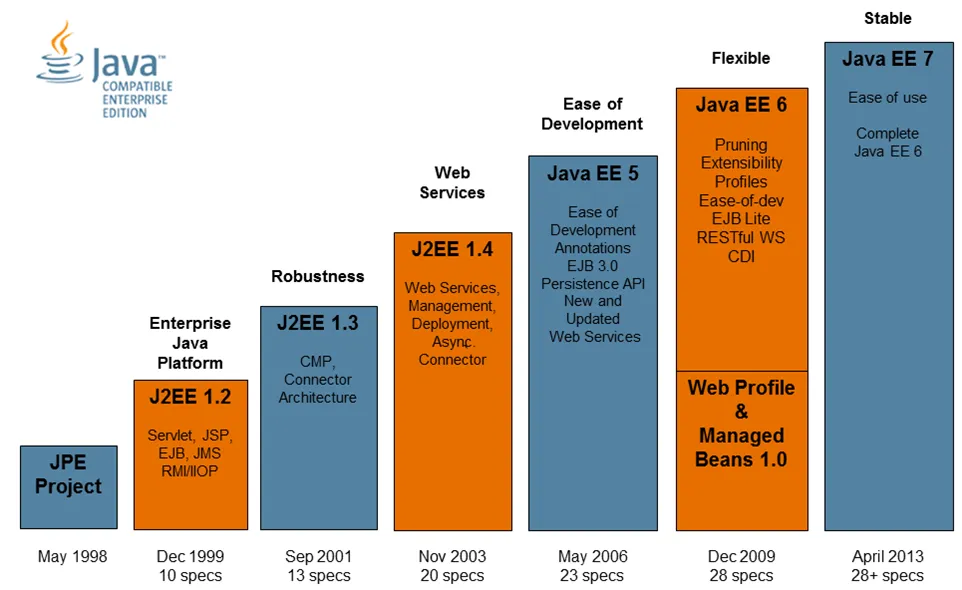
Sample Code of Java EE
Java EE (Enterprise Edition), now known as Jakarta EE, is typically used for building large-scale enterprise applications. Unlike Java SE, Java EE projects often involve the use of application servers and frameworks. Below is a simple example of a Java EE web application using Servlets. Note that this example assumes you have a basic understanding of Java EE and Servlets.
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/SimpleServlet")
public class SimpleServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// Set the response content type
response.setContentType("text/html");
// Get a PrintWriter object to send HTML response
PrintWriter out = response.getWriter();
// HTML content
out.println("<html>");
out.println("<head><title>Simple Java EE Servlet</title></head>");
out.println("<body>");
out.println("<h2>Hello from SimpleServlet!</h2>");
out.println("<p>This is a simple Java EE Servlet example.</p>");
out.println("</body>");
out.println("</html>");
}
}
In this example:
- We create a servlet by extending the
HttpServlet
class. - The
doGet
method is called when a GET request is made to the servlet. - We use the
@WebServlet
annotation to specify the URL pattern (“/SimpleServlet”) for accessing the servlet. - The
response.setContentType("text/html");
sets the response content type to HTML. - We use a
PrintWriter
to send HTML content as the response.
To run this Java EE example:
- Create a dynamic web project in your favorite Java EE IDE (e.g., Eclipse, IntelliJ).
- Copy the
SimpleServlet
code into the appropriate package in your project. - Deploy the project to a Java EE application server (e.g., Apache Tomcat).
- Access the servlet through a web browser using the specified URL pattern (e.g.,
http://localhost:8080/your-web-app-context/SimpleServlet
).
Keep in mind that more complex Java EE applications often involve additional technologies like JSP, EJB, JDBC, and frameworks like Spring or Jakarta EE specifications for building enterprise-level applications.