Introduction:
Golang, with its focus on simplicity, efficiency, and concurrency, is an excellent choice for network programming. In this article, we’ll explore the fundamentals of network programming using Golang. From basic socket programming to more advanced concepts like HTTP servers and WebSocket communication, we’ll cover various aspects of building network applications with Golang.
1. TCP and UDP Socket Programming:
TCP Socket Programming:
Golang provides a robust net
package for TCP socket programming. Below is a simple example of a TCP server and client.
// TCP Server package main import ( "fmt" "net" ) func main() { listener, _ := net.Listen("tcp", ":8080") defer listener.Close() for { conn, _ := listener.Accept() go handleConnection(conn) } } func handleConnection(conn net.Conn) { buffer := make([]byte, 1024) conn.Read(buffer) fmt.Println("Received:", string(buffer)) conn.Close() }
// TCP Client package main import ( "fmt" "net" ) func main() { conn, _ := net.Dial("tcp", "localhost:8080") defer conn.Close() message := []byte("Hello, TCP Server!") conn.Write(message) fmt.Println("Sent:", string(message)) }
UDP Socket Programming:
UDP is another common protocol for network communication. Golang’s net
package supports UDP socket programming as well.
// UDP Server package main import ( "fmt" "net" ) func main() { serverAddr, _ := net.ResolveUDPAddr("udp", ":8080") conn, _ := net.ListenUDP("udp", serverAddr) defer conn.Close() buffer := make([]byte, 1024) conn.ReadFromUDP(buffer) fmt.Println("Received:", string(buffer)) }
// UDP Client package main import ( "fmt" "net" ) func main() { serverAddr, _ := net.ResolveUDPAddr("udp", "localhost:8080") conn, _ := net.DialUDP("udp", nil, serverAddr) defer conn.Close() message := []byte("Hello, UDP Server!") conn.Write(message) fmt.Println("Sent:", string(message)) }
2. HTTP Servers and Clients:
HTTP Server:
Golang’s standard library includes a powerful http
package, making it easy to create HTTP servers.
package main import ( "fmt" "net/http" ) func main() { http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, HTTP Server!") }) http.ListenAndServe(":8080", nil) }
HTTP Client:
Golang also provides an HTTP client for making requests to servers.
package main import ( "fmt" "io/ioutil" "net/http" ) func main() { resp, _ := http.Get("http://localhost:8080") defer resp.Body.Close() body, _ := ioutil.ReadAll(resp.Body) fmt.Println("Response:", string(body)) }
3. WebSocket Communication:
WebSocket is a protocol providing full-duplex communication channels over a single TCP connection. The gorilla/websocket
package in Golang simplifies WebSocket implementation.
// WebSocket Server package main import ( "fmt" "net/http" "github.com/gorilla/websocket" ) var upgrader = websocket.Upgrader{ CheckOrigin: func(r *http.Request) bool { return true }, } func handler(w http.ResponseWriter, r *http.Request) { conn, _ := upgrader.Upgrade(w, r, nil) defer conn.Close() for { messageType, p, _ := conn.ReadMessage() if err := conn.WriteMessage(messageType, p); err != nil { return } } } func main() { http.HandleFunc("/ws", handler) http.ListenAndServe(":8080", nil) }
// WebSocket Client package main import ( "fmt" "log" "os" "os/signal" "github.com/gorilla/websocket" ) func main() { interrupt := make(chan os.Signal, 1) signal.Notify(interrupt, os.Interrupt) c, _, _ := websocket.DefaultDialer.Dial("ws://localhost:8080/ws", nil) defer c.Close() go func() { for { _, message, _ := c.ReadMessage() fmt.Println("Received:", string(message)) } }() for { select { case <-interrupt: log.Println("Interrupt signal received, closing connection.") err := c.WriteMessage(websocket.CloseMessage, websocket.FormatCloseMessage(websocket.CloseNormalClosure, "")) if err != nil { log.Println("Error closing connection:", err) return } return } } }
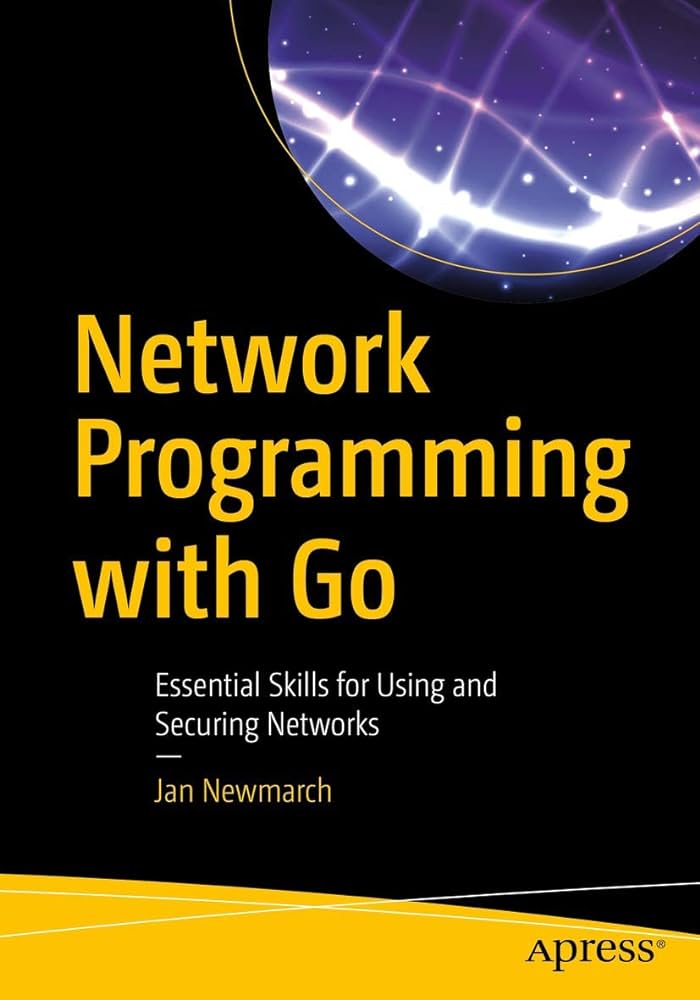
Conclusion:
Golang’s simplicity, concurrency model, and excellent standard library make it a strong choice for network programming. Whether you’re working with basic socket communication, building HTTP servers and clients, or implementing WebSocket communication, Golang provides powerful tools to streamline the development of network applications. Experiment with these examples and integrate them into your projects to leverage the full potential of Golang in network programming. Happy coding!