Firebase, a comprehensive mobile and web application development platform, provides a robust backend infrastructure that seamlessly integrates with various programming languages. In this article, we’ll explore the synergy between Python and Firebase, highlighting the steps to integrate Firebase services into your Python-based projects.
1. Introduction to Firebase:
Firebase, developed by Google, offers a suite of tools and services for building scalable and feature-rich applications. It includes real-time database, authentication, cloud functions, hosting, and more. Integrating Python with Firebase allows developers to harness the power of Firebase services while leveraging Python’s flexibility and readability.
2. Setting Up Firebase Project:
Before integrating Firebase with Python, create a Firebase project through the Firebase Console (https://console.firebase.google.com/). Follow the prompts to set up your project, and obtain the Firebase configuration settings, including the API key, project ID, and other necessary credentials.
3. Firebase Authentication with Python:
Firebase Authentication enables secure user sign-up, sign-in, and access control. While Firebase provides SDKs for various languages, Python developers can utilize the Firebase Admin SDK for server-side authentication. Install the firebase-admin
library using:
pip install firebase-admin
Now, initialize the Firebase Admin SDK in your Python application using the obtained credentials:
import firebase_admin from firebase_admin import credentials cred = credentials.Certificate('path/to/your/credentials.json') firebase_admin.initialize_app(cred)
4. Real-time Database with Python:
Firebase Realtime Database offers a NoSQL cloud database that synchronizes data between clients in real time. Python developers can interact with the Realtime Database using the pyrebase
library. Install it using:
pip install pyrebase
Now, configure and initialize Pyrebase in your Python script:
import pyrebase config = { "apiKey": "your_api_key", "authDomain": "your_project_id.firebaseapp.com", "databaseURL": "https://your_project_id.firebaseio.com", "storageBucket": "your_project_id.appspot.com" } firebase = pyrebase.initialize_app(config)
Interacting with the Realtime Database:
db = firebase.database() # Write data data = {"name": "John", "age": 28} db.child("users").push(data) # Read data users = db.child("users").get() for user in users.each(): print(user.key(), user.val())
5. Firebase Cloud Functions with Python:
Firebase Cloud Functions allow developers to run backend code in response to events triggered by Firebase features. While Cloud Functions primarily support Node.js, Python developers can use the functions-framework
library to write Cloud Functions in Python. Install it using:
pip install functions-framework
Define a simple function:
# main.py def hello_world(request): return "Hello, Firebase Cloud Functions!"
Run the function locally:
functions-framework --target=hello_world
Deploy the function to Firebase:
firebase deploy --only functions
6. Hosting Python Web Applications on Firebase:
Firebase Hosting allows developers to deploy web applications and serve content globally with a single command. While Firebase primarily supports static content, Python developers can use frameworks like Flask to deploy dynamic web applications.
# app.py from flask import Flask app = Flask(__name__) @app.route('/') def hello_world(): return 'Hello, Firebase Hosting with Python!' if __name__ == '__main__': app.run(host='127.0.0.1', port=8080, debug=True)
Deploy the Flask app to Firebase Hosting:
firebase deploy --only hosting
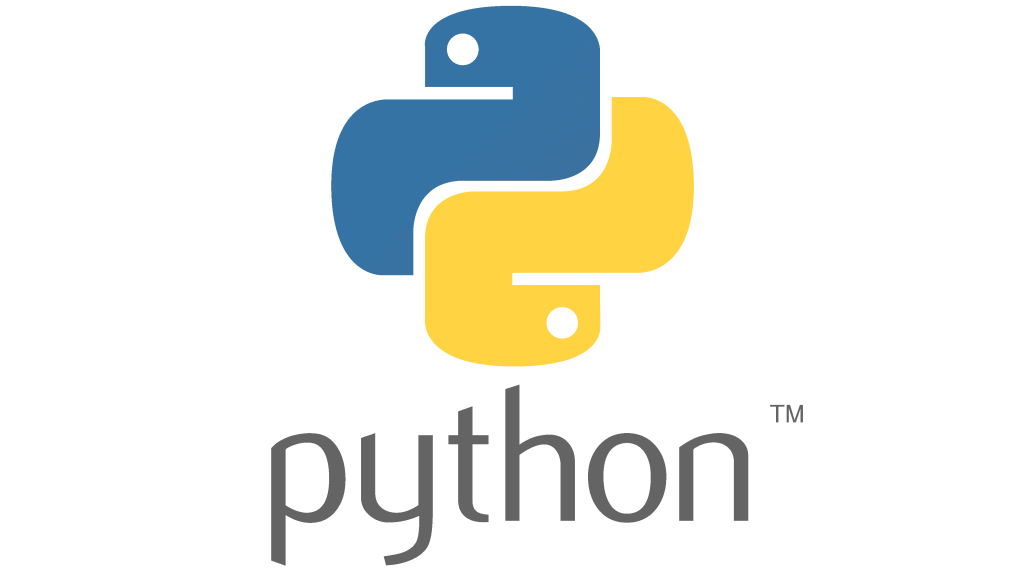
7. Conclusion:
Integrating Python with Firebase opens up a world of possibilities for developers aiming to build dynamic, real-time applications with a robust backend. Whether it’s handling authentication, interacting with the Realtime Database, deploying Cloud Functions, or hosting web applications, Firebase provides seamless integration points for Python developers. This synergy allows developers to leverage the strengths of both technologies, resulting in scalable, feature-rich applications that meet the demands of modern development. Explore the Firebase documentation and Python libraries to discover more capabilities and unlock the full potential of this powerful combination.