Introduction to ReactPHP
ReactPHP is a low-level library that enables asynchronous programming in PHP. It brings the concept of event-driven programming to PHP, allowing developers to perform non-blocking I/O operations efficiently. In this article, we will explore the fundamentals of asynchronous programming using ReactPHP and provide sample code to illustrate its implementation.
Understanding ReactPHP
ReactPHP is inspired by Node.js and follows a similar event-driven programming model. It allows PHP developers to build scalable and high-performance applications by handling multiple asynchronous operations concurrently. ReactPHP achieves this through the use of event loops, promises, and streams.
Setting Up ReactPHP
To get started with ReactPHP, you need to install the library using Composer. Create a new directory for your project and run the following commands in your terminal:
mkdir my-reactphp-project cd my-reactphp-project composer require react/event-loop
This will create a vendor
directory containing the ReactPHP library.
Sample Code: Asynchronous File Reading
Let’s create a simple example to demonstrate asynchronous file reading using ReactPHP. We’ll read the contents of two files concurrently and display the results when the reading is complete.
Create a file named async_file_reading.php
with the following content:
<?php require 'vendor/autoload.php'; use React\EventLoop\Factory; use React\Filesystem\Filesystem; use React\Filesystem\Stream\ReadableStream; // Create the event loop $loop = Factory::create(); // Create the filesystem object $filesystem = Filesystem::create($loop); // Define the files to read $file1 = 'file1.txt'; $file2 = 'file2.txt'; // Asynchronous file reading function function readFileAsync($file, $filesystem) { return $filesystem->file($file)->getContents(); } // Read files concurrently $promises = [ readFileAsync($file1, $filesystem), readFileAsync($file2, $filesystem), ]; // When all promises are fulfilled \React\Promise\all($promises)->then( function ($contents) { // Display the contents of each file echo "Contents of $file1: $contents[0]\n"; echo "Contents of $file2: $contents[1]\n"; }, function ($error) { // Handle errors echo "Error: $error\n"; } ); // Run the event loop $loop->run();
This code uses ReactPHP’s filesystem component to read the contents of two files concurrently. The getContents
method returns a promise, and we use React\Promise\all
to wait for both promises to be fulfilled before displaying the contents.
Save this file and create file1.txt
and file2.txt
with some content for testing.
Run the script using:
php async_file_reading.php
You should see the contents of both files displayed on the console.
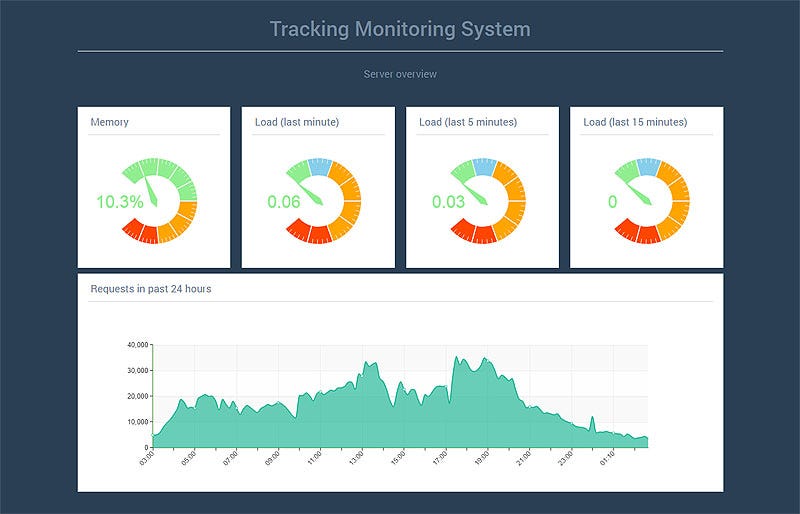
Conclusion
ReactPHP empowers PHP developers to embrace asynchronous programming, providing a set of tools and components to handle concurrency efficiently. This article introduced the basics of asynchronous file reading using ReactPHP, demonstrating how event loops and promises work together to achieve non-blocking I/O operations. As you delve deeper into ReactPHP, you’ll discover its versatility in handling various asynchronous tasks and building high-performance applications. Experiment with different scenarios and explore the ReactPHP documentation for more advanced features and components.