Introduction to PHP Amp
Asynchronous programming is a powerful paradigm that allows developers to handle concurrent tasks efficiently, making applications more responsive and scalable. In PHP, the Amp library provides a comprehensive set of tools for implementing asynchronous programming. In this article, we’ll delve into the fundamentals of asynchronous programming using Amp and provide sample code to illustrate its implementation.
Understanding Amp Library
Amp is a library for asynchronous programming in PHP. It introduces the concept of coroutines, which are functions that can be paused and resumed, allowing for non-blocking I/O operations. Amp provides an event loop, promises, and other utilities to simplify the development of asynchronous applications.
Setting Up Amp
To get started with Amp, you need to install the library using Composer. Create a new directory for your project and run the following commands in your terminal:
mkdir my-amp-project cd my-amp-project composer require amphp/amp
This will create a vendor
directory containing the Amp library.
Sample Code: Asynchronous File Reading
Let’s create a simple example to demonstrate asynchronous file reading using Amp. We’ll read the contents of two files concurrently and display the results when the reading is complete.
Create a file named async_file_reading.php
with the following content:
<?php require 'vendor/autoload.php'; use Amp\File\File; use Amp\File\Driver\UvDriver; use Amp\Loop; // Use the UvDriver for file operations Loop::set('driver', new UvDriver()); // Define the files to read $file1 = 'file1.txt'; $file2 = 'file2.txt'; // Asynchronous file reading function function readFileAsync($file): \Generator { /** @var File $fileHandle */ $fileHandle = yield File\open($file, 'r'); // Read the contents asynchronously $contents = yield $fileHandle->read(); // Close the file handle yield $fileHandle->close(); return $contents; } // Read files concurrently [$contents1, $contents2] = Amp\Promise\wait(Amp\Promise\all([ readFileAsync($file1), readFileAsync($file2), ])); // Display the contents of each file echo "Contents of $file1: $contents1\n"; echo "Contents of $file2: $contents2\n";
This code uses Amp’s File
component to read the contents of two files concurrently. The readFileAsync
function is a coroutine, indicated by the use of yield
, allowing for asynchronous file reading.
Save this file and create file1.txt
and file2.txt
with some content for testing.
Run the script using:
php async_file_reading.php
You should see the contents of both files displayed on the console.
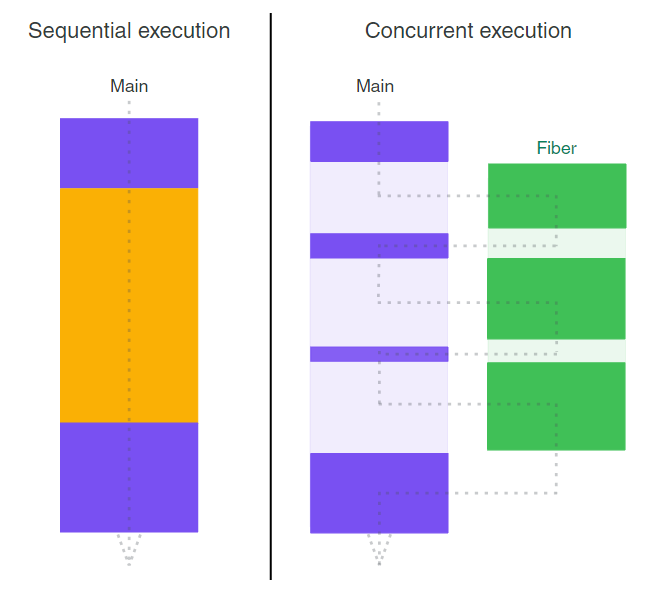
Conclusion
Amp brings the power of asynchronous programming to PHP, allowing developers to write efficient and responsive applications. This article introduced the basics of asynchronous file reading using Amp, demonstrating the use of coroutines and promises. As you explore Amp further, you’ll discover additional features and utilities for handling various asynchronous tasks. Experiment with different scenarios, explore the Amp documentation for more advanced features, and leverage the capabilities of asynchronous programming to enhance your PHP applications.
Reference
https://php.watch/versions/8.1/fibers