cURL (Client URL) is a powerful library and command-line tool for making HTTP requests. In PHP, the cURL extension provides a convenient way to interact with web services, APIs, and perform various HTTP-related tasks. In this article, we’ll explore the basics of using cURL in PHP and provide sample code for common use cases.
Installing cURL
Before using cURL in PHP, ensure that the cURL extension is installed and enabled. Most PHP installations include cURL by default, but you can confirm its presence by checking the output of phpinfo()
.
Making Simple HTTP Requests
Making a GET Request
<?php // Initialize cURL session $ch = curl_init(); // Set cURL options curl_setopt($ch, CURLOPT_URL, 'https://api.example.com/data'); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // Execute cURL session and fetch the response $response = curl_exec($ch); // Check for cURL errors if (curl_errno($ch)) { echo 'cURL error: ' . curl_error($ch); } // Close cURL session curl_close($ch); // Process the response echo $response; ?>
Making a POST Request
<?php // Initialize cURL session $ch = curl_init(); // Set cURL options for a POST request curl_setopt($ch, CURLOPT_URL, 'https://api.example.com/post'); curl_setopt($ch, CURLOPT_POST, true); curl_setopt($ch, CURLOPT_POSTFIELDS, ['key' => 'value']); // Execute cURL session and fetch the response $response = curl_exec($ch); // Check for cURL errors if (curl_errno($ch)) { echo 'cURL error: ' . curl_error($ch); } // Close cURL session curl_close($ch); // Process the response echo $response; ?>
Handling HTTP Headers
<?php // Initialize cURL session $ch = curl_init(); // Set cURL options with custom headers $headers = [ 'Content-Type: application/json', 'Authorization: Bearer YourAccessToken', ]; curl_setopt($ch, CURLOPT_URL, 'https://api.example.com/resource'); curl_setopt($ch, CURLOPT_HTTPHEADER, $headers); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // Execute cURL session and fetch the response $response = curl_exec($ch); // Check for cURL errors if (curl_errno($ch)) { echo 'cURL error: ' . curl_error($ch); } // Close cURL session curl_close($ch); // Process the response echo $response; ?>
Handling HTTP Cookies
<?php // Initialize cURL session $ch = curl_init(); // Set cURL options to handle cookies curl_setopt($ch, CURLOPT_URL, 'https://api.example.com/login'); curl_setopt($ch, CURLOPT_POST, true); curl_setopt($ch, CURLOPT_POSTFIELDS, ['username' => 'user', 'password' => 'pass']); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // Execute cURL session and fetch the response $response = curl_exec($ch); // Check for cURL errors if (curl_errno($ch)) { echo 'cURL error: ' . curl_error($ch); } // Close cURL session curl_close($ch); // Process the response echo $response; ?>
Handling SSL/TLS Connections
<?php // Initialize cURL session $ch = curl_init(); // Set cURL options for SSL/TLS curl_setopt($ch, CURLOPT_URL, 'https://secured-site.com/data'); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, 0); // Set to 1 for verification curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false); // Set to true for verification // Execute cURL session and fetch the response $response = curl_exec($ch); // Check for cURL errors if (curl_errno($ch)) { echo 'cURL error: ' . curl_error($ch); } // Close cURL session curl_close($ch); // Process the response echo $response; ?>
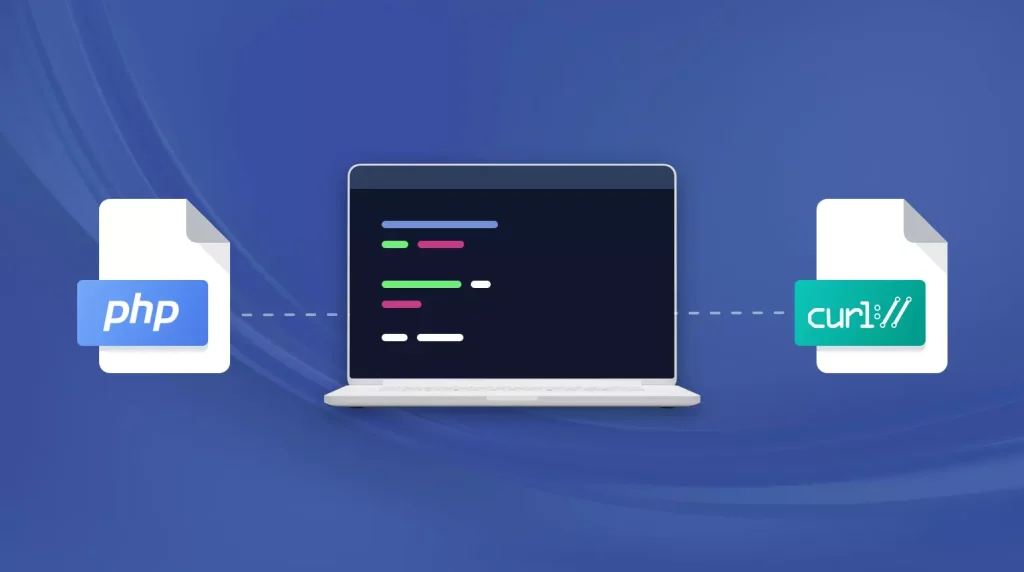
Conclusion
cURL is a versatile tool for handling HTTP requests, and the PHP cURL extension makes it accessible for developers. In this guide, we’ve covered the basics of making GET and POST requests, handling headers, cookies, and dealing with SSL/TLS connections using cURL in PHP. These examples serve as a solid foundation for incorporating cURL into your PHP projects, whether you’re interacting with APIs, consuming web services, or performing other HTTP-related tasks.