Introduction
Node.js and Firebase represent a powerful combination for developing modern web applications. Node.js, with its efficient and scalable server-side capabilities, pairs seamlessly with Firebase, a comprehensive backend-as-a-service (BaaS) platform provided by Google. In this article, we’ll explore how Node.js and Firebase work together to simplify the development process and empower developers to create feature-rich, real-time applications.
1. Introduction to Node.js and Firebase
Node.js:
Node.js is a JavaScript runtime built on the V8 JavaScript engine, commonly used for server-side development. Its non-blocking I/O and event-driven architecture make it well-suited for building scalable and high-performance applications.
Firebase:
Firebase is a mobile and web application development platform that provides a suite of tools and services for backend development. It includes real-time databases, authentication, cloud functions, hosting, and more, allowing developers to focus on building the frontend while Firebase handles the backend infrastructure.
2. Integrating Node.js with Firebase
Setting Up a Node.js Project:
To get started, create a new Node.js project using npm (Node Package Manager). Run the following commands in your terminal:
mkdir node-firebase-app cd node-firebase-app npm init -y
Installing Firebase:
Install the Firebase CLI globally to access Firebase tools and services:
npm install -g firebase-tools
Initializing Firebase:
Initialize Firebase in your project:
firebase init
Follow the prompts to select Firebase features for your project. This will generate necessary configuration files and folders.
3. Using Firebase Services in a Node.js Application
Firebase Authentication:
Enable user authentication easily with Firebase. Initialize the Firebase SDK in your Node.js application:
const admin = require('firebase-admin'); const serviceAccount = require('./path/to/serviceAccountKey.json'); admin.initializeApp({ credential: admin.credential.cert(serviceAccount), databaseURL: 'https://your-project-id.firebaseio.com', }); // Now you can use admin.auth() for Firebase Authentication
Real-time Database:
Firebase’s real-time database is seamlessly integrated with Node.js. Use the Firebase Admin SDK to interact with the database:
const admin = require('firebase-admin'); const serviceAccount = require('./path/to/serviceAccountKey.json'); admin.initializeApp({ credential: admin.credential.cert(serviceAccount), databaseURL: 'https://your-project-id.firebaseio.com', }); const db = admin.database(); const ref = db.ref('your-data'); // Example: Reading data ref.once('value', (snapshot) => { console.log(snapshot.val()); }); // Example: Writing data ref.set({ message: 'Hello, Firebase!' });
Firebase Cloud Functions:
Leverage Firebase Cloud Functions to run backend code in response to events. For example, automatically send a welcome email upon user registration:
const functions = require('firebase-functions'); const nodemailer = require('nodemailer'); exports.sendWelcomeEmail = functions.auth.user().onCreate((user) => { const transporter = nodemailer.createTransport({ // Configure email transport }); const mailOptions = { // Configure email content }; return transporter.sendMail(mailOptions); });
4. Hosting a Node.js Application on Firebase
Firebase Hosting allows you to deploy and host your Node.js application easily. Configure hosting in your firebase.json
file:
{ "hosting": { "public": "public", "rewrites": [ { "source": "**", "function": "app" } ] } }
Deploy your application to Firebase Hosting:
firebase deploy
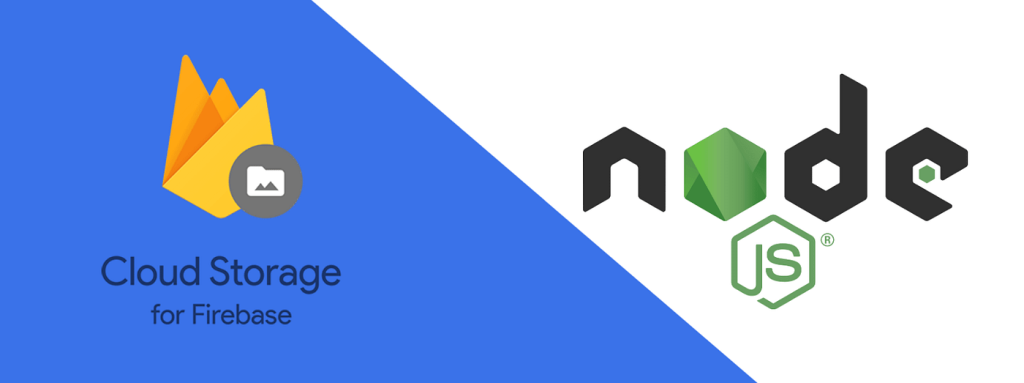
5. Conclusion
Combining Node.js with Firebase provides a comprehensive and efficient solution for developing modern web applications. Node.js handles server-side logic with its non-blocking I/O, while Firebase offers a suite of backend services, eliminating the need for extensive backend infrastructure development.
This powerful synergy allows developers to focus on building innovative features and delivering exceptional user experiences. Whether it’s real-time databases, authentication, or cloud functions, Node.js and Firebase together form a robust stack for developing scalable and dynamic web applications. As technology continues to evolve, the Node.js and Firebase combination remains a compelling choice for developers aiming to streamline their workflow and create cutting-edge applications.