Firebase, a mobile and web application development platform by Google, provides a comprehensive set of tools and services to streamline the development process. Combining the versatility of Java with the real-time capabilities of Firebase opens up new possibilities for creating dynamic and interactive applications. In this article, we’ll explore how to integrate Java with Firebase and showcase a simple example.
Firebase Overview
Firebase offers a suite of services for various aspects of app development:
- Realtime Database: A NoSQL cloud database that allows you to store and sync data in real-time.
- Authentication: Provides user authentication services using email/password, social media logins, and more.
- Cloud Firestore: A scalable and flexible NoSQL database for building web, mobile, and server applications.
- Cloud Functions: Serverless functions that respond to events triggered by Firebase features and HTTP requests.
- Cloud Storage: Securely upload and download files directly from your mobile and web apps.
- Hosting: Fast and secure web hosting for your static assets.
- Firebase SDKs: Libraries and SDKs for various platforms, including Android, iOS, and web.
Setting Up Firebase with Java
Before diving into the code, make sure you have a Firebase project set up on the Firebase Console. Obtain the configuration file (google-services.json
for Android or google-services.plist
for iOS) and add the Firebase SDK to your project.
Adding Firebase SDK to Java Project
For Java projects, Firebase primarily provides SDKs for Android. Follow these steps:
- Initialize Firebase:
- Download the Firebase Admin SDK for Java.
- Add the SDK to your project.
// Example Gradle dependency implementation 'com.google.firebase:firebase-admin:8.0.0'
- Initialize Firebase in your Java code:
import com.google.auth.oauth2.GoogleCredentials; import com.google.firebase.FirebaseApp; import com.google.firebase.FirebaseOptions; public class FirebaseInitializer { public static void initialize() { try { FileInputStream serviceAccount = new FileInputStream("path/to/your/serviceAccountKey.json"); FirebaseOptions options = new FirebaseOptions.Builder() .setCredentials(GoogleCredentials.fromStream(serviceAccount)) .setDatabaseUrl("https://your-project-id.firebaseio.com") .build(); FirebaseApp.initializeApp(options); } catch (IOException e) { e.printStackTrace(); } } }
Replace "path/to/your/serviceAccountKey.json"
with the path to your Firebase Admin SDK JSON file and "https://your-project-id.firebaseio.com"
with your Firebase project’s database URL.
Using Firebase Realtime Database with Java
Let’s create a simple Java program that interacts with the Firebase Realtime Database. In this example, we’ll write data to the database and then read it back.
import com.google.firebase.database.*; public class FirebaseExample { public static void main(String[] args) { FirebaseInitializer.initialize(); DatabaseReference databaseReference = FirebaseDatabase.getInstance().getReference("messages"); // Writing data to the database databaseReference.setValue("Hello, Firebase!"); // Reading data from the database databaseReference.addValueEventListener(new ValueEventListener() { @Override public void onDataChange(DataSnapshot dataSnapshot) { String message = dataSnapshot.getValue(String.class); System.out.println("Message from Firebase: " + message); } @Override public void onCancelled(DatabaseError databaseError) { System.err.println("Failed to read value: " + databaseError.toException()); } }); } }
This example demonstrates basic interaction with the Firebase Realtime Database using Java. The setValue
method writes data to the database, and the addValueEventListener
method listens for changes and reads the data when it’s updated.
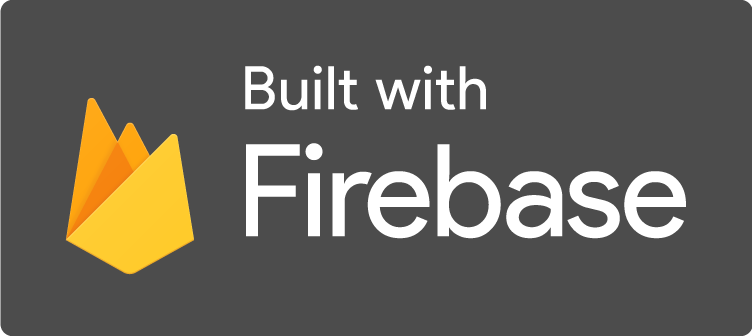
Integrating Firebase with Java Web Applications
For Java web applications, Firebase can be integrated using the Firebase JavaScript SDK. Add the SDK to your HTML page:
<!-- Add Firebase products that you want to use --> <script defer src="/__/firebase/8.0.0/firebase-app.js"></script> <script defer src="/__/firebase/8.0.0/firebase-auth.js"></script> <script defer src="/__/firebase/8.0.0/firebase-database.js"></script> <!-- ... other Firebase SDKs --> <script defer src="/__/firebase/init.js"></script>
Then, use JavaScript to interact with Firebase services in your web application.
Conclusion
Integrating Java with Firebase opens up a world of possibilities for building powerful and dynamic applications. Whether you’re developing Android apps, Java-based microservices, or web applications, Firebase provides a scalable and real-time backend solution. The combination of Java’s robustness and Firebase’s features empowers developers to create modern and responsive applications with ease. Explore more Firebase services and tailor them to your application’s specific needs for a seamless and efficient development experience.