Exploring Data Science in C#: A Comprehensive Guide with Practical Examples
Data Science is a multidisciplinary field that leverages various techniques to extract knowledge and insights from data. While languages like Python and R are widely associated with Data Science, C# has been gaining traction in this space. In this article, we’ll delve into the world of Data Science using C#, covering key concepts and demonstrating practical examples.
Introduction to Data Science in C#:
Data Science in C# involves using the language’s powerful features to manipulate, analyze, and visualize data. Developers can leverage libraries, frameworks, and tools to perform tasks such as data cleaning, exploration, statistical analysis, machine learning, and visualization.
Key Libraries for Data Science in C#:
- Math.NET Numerics:
- Math.NET Numerics provides numerical computing capabilities, making it suitable for tasks like linear algebra, statistics, and optimization.
- Accord.NET:
- Accord.NET is a machine learning framework that supports various algorithms for classification, regression, clustering, and more.
- Deedle:
- Deedle is a library for exploratory data analysis and time series manipulation.
- Microsoft.ML:
- Microsoft.ML is part of the ML.NET framework, providing machine learning capabilities for tasks like regression, classification, clustering, and recommendation.
Data Science Workflow in C#:
Let’s walk through a simplified Data Science workflow using C#. We’ll perform basic data analysis using Math.NET Numerics, exploratory data analysis with Deedle, and build a simple machine learning model with Microsoft.ML.
1. Data Loading and Exploration with Deedle:
using Deedle; class Program { static void Main() { // Load a CSV file into a Deedle DataFrame var dataFrame = Frame.ReadCsv("iris_dataset.csv"); // Display summary statistics Console.WriteLine(dataFrame.Summary()); } }
2. Data Analysis with Math.NET Numerics:
using MathNet.Numerics.LinearAlgebra; class Program { static void Main() { // Load a matrix from a CSV file var matrix = Matrix<double>.Build.DenseOfArray(new double[,] { /* data */ }); // Perform statistical analysis var mean = matrix.RowSums() / matrix.RowCount; var standardDeviation = matrix.EnumerateRows().Select(row => row.PointwisePower(2).Sum()).ToPointwisePower(0.5); } }
3. Machine Learning with Microsoft.ML:
using Microsoft.ML; using Microsoft.ML.Data; public class IrisData { [LoadColumn(0)] public float SepalLength; [LoadColumn(1)] public float SepalWidth; [LoadColumn(2)] public float PetalLength; [LoadColumn(3)] public float PetalWidth; [LoadColumn(4)] public string Label; } public class IrisPrediction { [ColumnName("PredictedLabel")] public string PredictedLabel; } class Program { static void Main() { // Create MLContext var mlContext = new MLContext(); // Load data var data = mlContext.Data.LoadFromTextFile<IrisData>("iris_dataset.csv", separatorChar: ','); // Define data processing pipeline var pipeline = mlContext.Transforms.Conversion.MapValueToKey("Label") .Append(mlContext.Transforms.Conversion.MapKeyToValue("PredictedLabel")); // Choose a machine learning algorithm var trainer = mlContext.Transforms.Conversion.MapKeyToValue("Label") .Append(mlContext.Transforms.Conversion.MapValueToKey("Label")) .Append(mlContext.Transforms.Conversion.MapKeyToValue("Label")) .Append(mlContext.BinaryClassification.Trainers.SdcaLogisticRegression()); // Create and train the model var model = pipeline.Append(trainer).Fit(data); // Make predictions var predictionEngine = mlContext.Model.MakePredictionFunction<IrisData, IrisPrediction>(model); var prediction = predictionEngine.Predict(new IrisData { SepalLength = 5.1f, SepalWidth = 3.5f, PetalLength = 1.4f, PetalWidth = 0.2f }); Console.WriteLine($"Predicted label: {prediction.PredictedLabel}"); } }
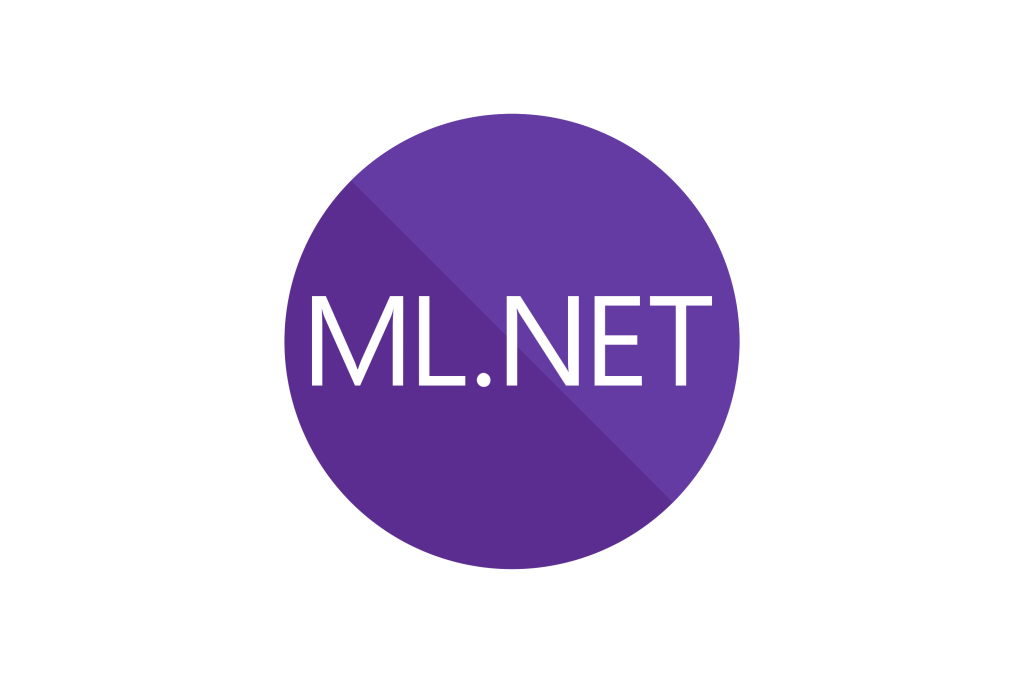
Conclusion: Empowering Data Science in C#:
While C# may not be as synonymous with Data Science as Python or R, it offers a robust set of tools and libraries for developers to perform data analysis and machine learning tasks. Whether you’re manipulating data with Math.NET Numerics, exploring datasets with Deedle, or building machine learning models with Microsoft.ML, C# provides a versatile platform for data-driven development. As the ecosystem continues to evolve, C# is increasingly becoming a viable option for those exploring the exciting field of Data Science.