Introduction
Firebase, a mobile and web application development platform, provides a wide range of services to build powerful and scalable applications. While Firebase is often associated with frontend technologies like JavaScript, it can also be seamlessly integrated with backend technologies such as PHP. In this article, we will explore how to integrate PHP with Firebase, unlocking a myriad of possibilities for real-time data synchronization, authentication, and more.
What is Firebase?
Firebase is a comprehensive suite of cloud-based tools and services provided by Google. It includes features like real-time databases, authentication, hosting, cloud functions, and more. Firebase simplifies the development process by offering a set of tools that work seamlessly together, allowing developers to focus on building features rather than managing infrastructure.
Integrating PHP with Firebase:
1. Firebase Realtime Database:
Firebase Realtime Database is a NoSQL cloud-hosted database that allows developers to store and sync data in real-time. To interact with the Firebase Realtime Database using PHP, you can use the Firebase REST API.
#### Sample PHP code to push data to Firebase Realtime Database:
<?php $firebaseUrl = 'https://your-firebase-project-id.firebaseio.com'; $path = '/users.json'; $data = [ 'name' => 'John Doe', 'email' => '[email protected]', ]; $firebaseUrl .= $path; $ch = curl_init($firebaseUrl); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_CUSTOMREQUEST, 'POST'); curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data)); $response = curl_exec($ch); curl_close($ch); echo 'Data pushed to Firebase Realtime Database.';
2. Firebase Authentication:
Firebase Authentication provides secure authentication and user management. While Firebase Authentication is often used on the frontend, you can also integrate it with your PHP backend.
#### Sample PHP code to verify a Firebase ID token:
<?php require 'vendor/autoload.php'; use Firebase\JWT\JWT; $idToken = 'YOUR_FIREBASE_ID_TOKEN'; $projectId = 'your-firebase-project-id'; $firebasePublicKey = file_get_contents("https://www.googleapis.com/robot/v1/metadata/x509/[email protected]"); $decodedToken = JWT::decode($idToken, $firebasePublicKey, ['RS256']); // Verify the project ID if ($decodedToken->aud !== $projectId) { die('Invalid project ID'); } echo 'User authenticated successfully.';
Make sure to install the required JWT library using Composer:
composer require firebase/php-jwt
3. Firebase Cloud Messaging (FCM):
Firebase Cloud Messaging allows you to send messages and notifications to users. You can integrate FCM with your PHP backend to send push notifications.
#### Sample PHP code to send a push notification:
<?php $serverKey = 'YOUR_FIREBASE_SERVER_KEY'; $title = 'Notification Title'; $body = 'Notification Body'; $data = [ 'title' => $title, 'body' => $body, ]; $notification = [ 'title' => $title, 'body' => $body, ]; $fields = [ 'to' => '/topics/all', // Send to a specific topic or device 'notification' => $notification, 'data' => $data, ]; $headers = [ 'Authorization: key=' . $serverKey, 'Content-Type: application/json', ]; $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, 'https://fcm.googleapis.com/fcm/send'); curl_setopt($ch, CURLOPT_POST, true); curl_setopt($ch, CURLOPT_HTTPHEADER, $headers); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($fields)); $result = curl_exec($ch); curl_close($ch); echo 'Push notification sent successfully.';
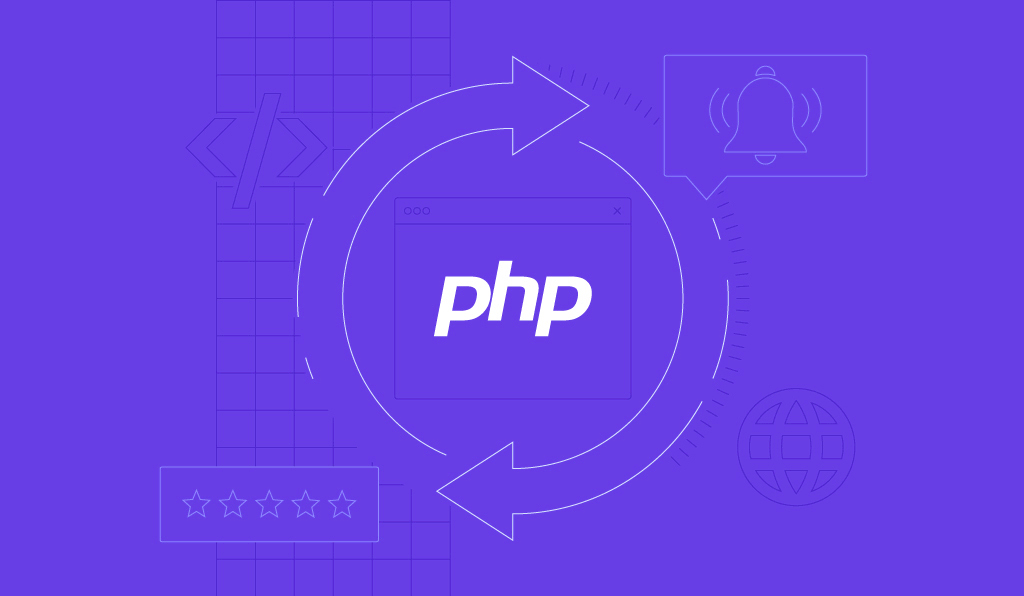
Conclusion:
Integrating PHP with Firebase opens up a world of possibilities for building dynamic, real-time applications with features like data synchronization, user authentication, and push notifications. The examples provided cover Firebase Realtime Database, Authentication, and Cloud Messaging, showcasing how PHP developers can harness the power of Firebase services within their server-side applications. As Firebase continues to evolve, exploring additional features and integrations can further enhance the capabilities of PHP applications in the Firebase ecosystem.