Object-Oriented Design Patterns are reusable solutions to common problems encountered in software design. They provide a structured way to solve recurring design challenges and promote best practices in code organization, flexibility, and maintainability. In this article, we will explore some widely used Object-Oriented Design Patterns in the context of PHP.
1. Singleton Pattern
The Singleton Pattern ensures that a class has only one instance and provides a global point of access to it. This is particularly useful when exactly one object is needed to coordinate actions across the system.
Example:
<?php class Singleton { private static $instance; private function __construct() {} public static function getInstance() { if (self::$instance === null) { self::$instance = new self(); } return self::$instance; } } $instance1 = Singleton::getInstance(); $instance2 = Singleton::getInstance(); var_dump($instance1 === $instance2); // Output: true ?>
2. Factory Method Pattern
The Factory Method Pattern defines an interface for creating an object, but leaves the choice of its type to the subclasses, creating a way to delegate the instantiation responsibility to subclasses.
Example:
<?php interface Product { public function getName(); } class ConcreteProduct implements Product { public function getName() { return "Concrete Product"; } } interface Factory { public function createProduct(): Product; } class ConcreteFactory implements Factory { public function createProduct(): Product { return new ConcreteProduct(); } } $factory = new ConcreteFactory(); $product = $factory->createProduct(); echo $product->getName(); // Output: Concrete Product ?>
3. Observer Pattern
The Observer Pattern defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically.
Example:
<?php interface Observer { public function update(string $message); } class ConcreteObserver implements Observer { public function update(string $message) { echo "Received update: $message\n"; } } class Subject { private $observers = []; public function addObserver(Observer $observer) { $this->observers[] = $observer; } public function notifyObservers(string $message) { foreach ($this->observers as $observer) { $observer->update($message); } } } $observer = new ConcreteObserver(); $subject = new Subject(); $subject->addObserver($observer); $subject->notifyObservers("Hello observers!"); ?>
4. Strategy Pattern
The Strategy Pattern defines a family of algorithms, encapsulates each one, and makes them interchangeable. It lets the client vary the algorithm independently from the clients that use it.
Example:
<?php interface PaymentMethod { public function pay(float $amount); } class CreditCardPayment implements PaymentMethod { public function pay(float $amount) { echo "Paid $amount using Credit Card.\n"; } } class PayPalPayment implements PaymentMethod { public function pay(float $amount) { echo "Paid $amount using PayPal.\n"; } } class ShoppingCart { private $paymentMethod; public function setPaymentMethod(PaymentMethod $paymentMethod) { $this->paymentMethod = $paymentMethod; } public function checkout(float $totalAmount) { $this->paymentMethod->pay($totalAmount); } } $cart = new ShoppingCart(); // Client can switch between payment methods easily $cart->setPaymentMethod(new CreditCardPayment()); $cart->checkout(100.0); $cart->setPaymentMethod(new PayPalPayment()); $cart->checkout(50.0); ?>
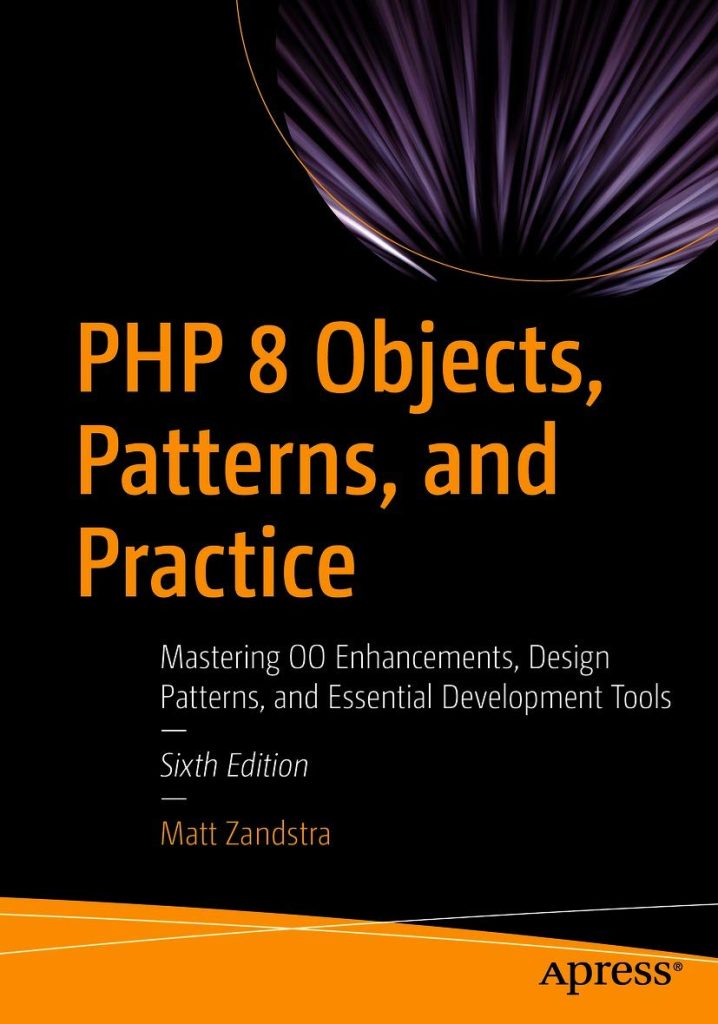
Conclusion
Object-Oriented Design Patterns play a crucial role in building scalable, maintainable, and flexible software systems. These patterns provide solutions to common design problems, offering a structured approach to software development. Understanding and applying these patterns can significantly improve the quality and maintainability of your PHP code. As you gain more experience, you’ll recognize situations where these patterns can be effectively applied, leading to more elegant and efficient solutions.