Sure, here are brief descriptions of each language:
- Rust:
Rust is a systems programming language known for its focus on safety, concurrency, and performance. It aims to prevent common programming errors, such as null pointer dereferencing and data races, through its ownership system. Rust provides memory safety without garbage collection, making it suitable for low-level systems programming while also being expressive and efficient. Its strong type system and pattern matching features make it an attractive choice for building reliable and high-performance software. - Golang (Go):
Go, often referred to as Golang, is a statically typed, compiled programming language developed by Google. It is designed for simplicity and efficiency, with a focus on concurrency and scalability. Go’s syntax is clean and easy to read, making it suitable for building large-scale, distributed systems. It includes built-in support for concurrency through goroutines and channels, which simplifies parallelism without compromising safety. Go’s standard library is extensive, covering a wide range of tasks from networking to cryptography, making it a versatile choice for various applications. - C++:
C++ is a general-purpose programming language known for its high performance, flexibility, and wide range of applications. It is an extension of the C programming language with added features such as classes, templates, and exceptions. C++ allows both low-level memory manipulation and high-level abstractions, making it suitable for systems programming, game development, and high-performance computing. It provides a balance between performance and productivity, allowing developers to write efficient code without sacrificing expressiveness. C++ is widely used in industries where performance and efficiency are critical, such as finance, gaming, and embedded systems.
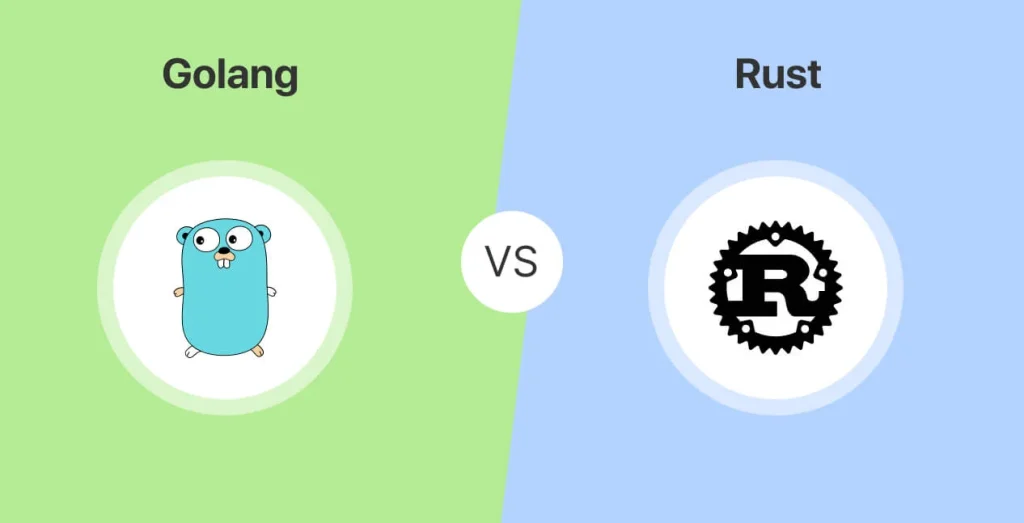
Compare between Rust, Golang, and C++ with side by side
Here’s a side-by-side comparison of Rust, Golang (Go), and C++:
Feature | Rust | Golang (Go) | C++ |
---|---|---|---|
Type System | – Strong, static typing | – Strong, static typing | – Strong, static typing |
– Type inference | – Type inference | – Type inference | |
– Algebraic data types (enums) | – Structs, interfaces | – Classes, structs, unions | |
– Pattern matching | – No pattern matching | – Limited pattern matching (via libraries) | |
Memory Safety | – Ownership system prevents data races | – Garbage collection (optional) | – Manual memory management |
– No null pointers | – No null pointers | – Null pointers allowed (unless using smart pointers) | |
– Borrow checker enforces ownership rules | – No borrowing concept | – Smart pointers for memory safety (e.g., unique_ptr, shared_ptr) | |
Concurrency | – Fearless concurrency through ownership | – Goroutines and channels | – Threading and libraries (e.g., std::thread) |
– No data races | – Built-in support for concurrency | – Potential for data races | |
Performance | – Comparable to C/C++ in most cases | – Efficient, but slightly behind C/C++ | – High performance, often used as a benchmark |
– No runtime overhead (except for safety features) | – Low latency, suitable for web services | – Highly optimized compilers | |
Usage | – Systems programming, embedded systems | – Web development, cloud services | – Systems programming, game development |
– Command-line tools, performance-critical applications | – DevOps, microservices | – Performance-sensitive applications | |
Learning Curve | – Steeper due to ownership and borrowing | – Gentle learning curve | – Steep learning curve, especially for beginners |
– Requires understanding of lifetimes | – Simple syntax and built-in tools | – Complex syntax and language features | |
Ecosystem | – Growing ecosystem with libraries for various tasks | – Mature ecosystem with extensive standard library | – Rich ecosystem with many third-party libraries |
– Cargo package manager | – Go module system | – CMake, Conan, and other build systems |
Each language has its strengths and weaknesses, making them suitable for different use cases and preferences. Rust excels in memory safety and concurrency, making it ideal for systems programming. Go prioritizes simplicity, efficiency, and concurrency, making it a popular choice for web development and cloud services. C++ offers high performance and flexibility, making it suitable for a wide range of applications, especially those that require low-level control and optimization. Ultimately, the choice between these languages depends on the specific requirements of the project and the preferences of the developers.
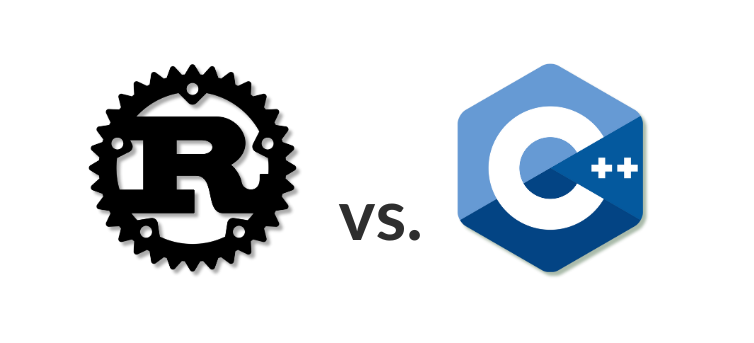
How Many Framework of Rust, Golang, and C++
Rust Frameworks:
Rust, known for its focus on safety and performance, has a growing ecosystem of frameworks and libraries to support various use cases. Some popular Rust frameworks include:
- Actix Web: Actix Web is a powerful, actor-based web framework for Rust. It provides a robust foundation for building high-performance, asynchronous web applications. Actix Web leverages Rust’s concurrency features to handle thousands of connections concurrently while remaining memory safe.
- Rocket: Rocket is a web framework for Rust that prioritizes simplicity and ease of use. It offers a clean and intuitive API for building web applications quickly. Rocket’s strong type system and compile-time checks help developers catch errors early in the development process.
- Tokio: Tokio is an asynchronous runtime for Rust that enables developers to write non-blocking, event-driven applications. It provides a powerful foundation for building network services, microservices, and distributed systems. Tokio leverages Rust’s async/await syntax to simplify asynchronous programming.
- Yew: Yew is a modern web framework for Rust that leverages WebAssembly to enable frontend development in Rust. It provides a familiar component-based architecture similar to React.js, allowing developers to build interactive web applications using Rust’s type safety and performance.
- Diesel: Diesel is a powerful ORM (Object-Relational Mapping) framework for Rust that simplifies database interactions. It provides a type-safe query builder and schema migration tools, making it easy to work with databases in Rust projects. Diesel supports various database backends, including PostgreSQL, MySQL, and SQLite.
These are just a few examples of the frameworks available in the Rust ecosystem. As Rust continues to gain popularity, developers can expect to see more frameworks and libraries emerge to support a wide range of applications and use cases.
Golang Frameworks:
Go, known for its simplicity, efficiency, and built-in support for concurrency, has a mature ecosystem of frameworks and libraries. Some popular Go frameworks include:
- Gin: Gin is a high-performance web framework for Go that prioritizes minimalism and speed. It provides a fast HTTP router and middleware support, making it ideal for building RESTful APIs and microservices. Gin’s simplicity and performance make it a popular choice among Go developers.
- Echo: Echo is a lightweight and fast web framework for Go that aims to provide a minimalistic API for building web applications. It offers features such as route grouping, middleware chaining, and parameter parsing, making it easy to develop robust web services in Go.
- Beego: Beego is a full-featured MVC (Model-View-Controller) web framework for Go that includes built-in support for ORM, session management, and internationalization. It follows the convention over configuration principle, allowing developers to quickly build scalable web applications with minimal boilerplate code.
- Buffalo: Buffalo is a web development eco-system for Go that aims to streamline the process of building modern web applications. It includes a web framework, a set of generators, and tools for database migrations, asset management, and testing. Buffalo’s batteries-included approach simplifies the development of Go web applications.
- Gorm: Gorm is a popular ORM framework for Go that provides a simple and expressive API for interacting with databases. It supports various database backends, including MySQL, PostgreSQL, and SQLite, and offers features such as automatic migrations, associations, and preloading.
These frameworks represent just a fraction of the tools available in the Go ecosystem. With its growing popularity and active community, developers can expect to see continued innovation and expansion of the Go framework landscape.
C++ Frameworks:
C++, a powerful and flexible programming language known for its performance and versatility, has a wide range of frameworks and libraries available for various domains. Some popular C++ frameworks include:
- Qt: Qt is a cross-platform application framework for C++ that enables developers to build graphical user interfaces (GUIs) and cross-platform applications. It provides a comprehensive set of tools and libraries for desktop, mobile, and embedded development, making it a popular choice for software development across industries.
- Boost: Boost is a collection of libraries for C++ that provides support for a wide range of tasks, including concurrency, networking, filesystem operations, and data structures. It serves as an essential toolkit for C++ developers, offering high-quality, peer-reviewed libraries that extend the capabilities of the language.
- Poco: Poco (POrtable COmponents) is a C++ class library and framework for building network-centric, portable applications. It provides abstractions for networking, threads, file systems, and other common tasks, allowing developers to write portable and efficient code across different platforms.
- SFML: SFML (Simple and Fast Multimedia Library) is a multimedia framework for C++ that provides a simple and intuitive API for window management, graphics rendering, audio playback, and input handling. It is well-suited for developing games and multimedia applications in C++, with support for both 2D and 3D graphics.
- CppCMS: CppCMS is a web application framework for C++ that focuses on performance, scalability, and security. It provides features such as template-based content generation, session management, and data caching, making it suitable for building high-performance web applications in C++.
These frameworks represent just a subset of the tools available in the C++ ecosystem. With its long history and wide adoption, C++ continues to be a popular choice for building performance-critical and resource-constrained applications across various domains.
Sample code of Rust, Golang, C++
Programming languages come with their unique syntax, paradigms, and features. Rust, Golang (Go), and C++ are three popular languages, each with its strengths and areas of application. Let’s explore a simple example code snippet in each language to understand their syntax and approach to solving problems.
Rust:
Rust is a systems programming language focused on safety, performance, and concurrency. Here’s a simple Rust program that prints “Hello, world!” to the console:
fn main() { println!("Hello, world!"); }
In this code:
fn main()
defines the entry point of the program.println!
is a macro for printing formatted text to the console.!
indicates thatprintln
is a macro, not a function.
Rust’s syntax is expressive yet concise, with a strong emphasis on safety and memory management.
Golang (Go):
Go is a statically typed, compiled language designed for simplicity and efficiency. Here’s the equivalent “Hello, world!” program in Go:
package main import "fmt" func main() { fmt.Println("Hello, world!") }
In this code:
package main
declares that this file is part of the main package.import "fmt"
imports the “fmt” package, which provides formatted I/O functions.func main()
defines the entry point of the program.fmt.Println
prints the string “Hello, world!” to the console.
Go’s syntax is straightforward and readable, with a focus on simplicity and ease of use.
C++:
C++ is a general-purpose language known for its performance and flexibility. Here’s the “Hello, world!” program in C++:
#include <iostream> int main() { std::cout << "Hello, world!" << std::endl; return 0; }
In this code:
#include <iostream>
includes the iostream header, which provides I/O functions.int main()
defines the entry point of the program.std::cout << "Hello, world!" << std::endl;
prints the string “Hello, world!” to the console.return 0;
indicates successful program termination.
C++’s syntax can be more verbose compared to Rust and Go, but it offers fine-grained control and high performance.
Rust, Golang (Go), and C++ are three powerful languages with their unique features and syntax. Rust emphasizes safety and concurrency, Go focuses on simplicity and efficiency, while C++ provides flexibility and performance. Understanding their strengths and weaknesses can help developers choose the right tool for their projects.
Try running these simple programs in each language to experience firsthand how they differ in syntax and approach.
More Complicated code of Rust, Golang, and C++
Certainly! Let’s explore more complex examples in Rust, Golang (Go), and C++. We’ll create programs that perform basic file I/O operations, demonstrating each language’s approach to handling such tasks.
Rust:
In Rust, we’ll create a program that reads a text file and counts the number of words in it. Here’s the code:
use std::fs::File; use std::io::{BufRead, BufReader}; fn main() { if let Ok(file) = File::open("example.txt") { let reader = BufReader::new(file); let mut word_count = 0; for line in reader.lines() { if let Ok(line) = line { word_count += line.split_whitespace().count(); } } println!("Total words: {}", word_count); } else { eprintln!("Error: Unable to open file."); } }
In this Rust code:
- We import necessary modules for file I/O (
std::fs::File
) and buffered reading (std::io::{BufRead, BufReader}
). - We attempt to open the file “example.txt” and handle any errors that occur.
- We read each line from the file, split it into words, and count the total number of words.
- Finally, we print the total word count.
Golang (Go):
In Go, we’ll accomplish the same task of counting words in a text file:
package main import ( "bufio" "fmt" "os" "strings" ) func main() { file, err := os.Open("example.txt") if err != nil { fmt.Fprintf(os.Stderr, "Error: %v\n", err) return } defer file.Close() scanner := bufio.NewScanner(file) wordCount := 0 for scanner.Scan() { words := strings.Fields(scanner.Text()) wordCount += len(words) } fmt.Printf("Total words: %d\n", wordCount) }
In this Go code:
- We open the file “example.txt” and handle any errors.
- We use a scanner to read the file line by line.
- For each line, we split it into words using
strings.Fields
and count the total number of words. - Finally, we print the total word count.
C++:
In C++, we’ll implement the word counting program using standard file streams and string manipulation:
#include <iostream> #include <fstream> #include <string> #include <sstream> int main() { std::ifstream file("example.txt"); if (!file.is_open()) { std::cerr << "Error: Unable to open file." << std::endl; return 1; } std::string line; int wordCount = 0; while (std::getline(file, line)) { std::istringstream iss(line); std::string word; while (iss >> word) { ++wordCount; } } std::cout << "Total words: " << wordCount << std::endl; return 0; }
In this C++ code:
- We open the file “example.txt” and handle any errors.
- We read each line from the file using
std::getline
. - For each line, we use a
std::istringstream
to split it into words and count the total number of words. - Finally, we print the total word count.
These examples demonstrate how Rust, Golang (Go), and C++ handle file I/O and string manipulation tasks with their respective standard libraries. Each language offers its unique syntax and features while achieving similar functionality.
Work on Database PostgreSQL with Rust, Golang, and C++
Certainly! Let’s modify the previous examples to work with PostgreSQL databases. We’ll create programs that connect to a PostgreSQL database, read data from a table, and perform basic operations like counting the number of rows.
Rust:
In Rust, we’ll use the postgres
crate to interact with PostgreSQL databases. Here’s a simple example that connects to a PostgreSQL database, reads data from a table, and counts the number of rows:
extern crate postgres; use postgres::{Client, NoTls}; fn main() { let mut client = Client::connect("postgresql://username:password@localhost/dbname", NoTls) .expect("Error connecting to database"); let rows = client.query("SELECT * FROM tablename", &[]).expect("Error fetching data"); println!("Total rows: {}", rows.len()); }
Replace "postgresql://username:password@localhost/dbname"
with your PostgreSQL connection string and "SELECT * FROM tablename"
with your SQL query.
Golang (Go):
In Go, we’ll use the github.com/lib/pq
package to interact with PostgreSQL databases. Here’s an example that connects to a PostgreSQL database, reads data from a table, and counts the number of rows:
package main import ( "database/sql" "fmt" _ "github.com/lib/pq" ) func main() { connStr := "user=username password=password dbname=dbname sslmode=disable" db, err := sql.Open("postgres", connStr) if err != nil { fmt.Println("Error connecting to database:", err) return } defer db.Close() var rowCount int err = db.QueryRow("SELECT COUNT(*) FROM tablename").Scan(&rowCount) if err != nil { fmt.Println("Error fetching data:", err) return } fmt.Println("Total rows:", rowCount) }
Replace "user=username password=password dbname=dbname sslmode=disable"
with your PostgreSQL connection string and "SELECT COUNT(*) FROM tablename"
with your SQL query.
C++:
In C++, we’ll use the pqxx
library to interact with PostgreSQL databases. Here’s an example that connects to a PostgreSQL database, reads data from a table, and counts the number of rows:
#include <iostream> #include <pqxx/pqxx> int main() { try { pqxx::connection conn("postgresql://username:password@localhost/dbname"); if (!conn.is_open()) { std::cerr << "Error connecting to database" << std::endl; return 1; } pqxx::work txn(conn); pqxx::result result = txn.exec("SELECT COUNT(*) FROM tablename"); int rowCount = result[0][0].as<int>(); std::cout << "Total rows: " << rowCount << std::endl; txn.commit(); } catch (const std::exception &e) { std::cerr << "Error: " << e.what() << std::endl; return 1; } return 0; }
Replace "postgresql://username:password@localhost/dbname"
with your PostgreSQL connection string and "SELECT COUNT(*) FROM tablename"
with your SQL query.
These examples demonstrate how to connect to a PostgreSQL database and perform basic operations using Rust, Golang (Go), and C++. Adjust the connection string and SQL query according to your PostgreSQL setup and requirements.
Companies use Rust, Golang (Go), and C++
Exploring Companies Using Rust, Golang, and C++
Programming languages play a crucial role in the tech industry, powering a wide range of applications and systems. Rust, Golang (Go), and C++ are three popular languages, each with its unique strengths and areas of application. Let’s take a look at some notable companies that use these languages in their products and services.
Rust:
Rust has gained popularity in recent years, particularly in industries where safety, concurrency, and performance are paramount. Some companies that use Rust in their projects include:
- Mozilla: As the creator of Rust, Mozilla uses the language extensively in various projects, including Firefox, Servo (a next-generation web browser engine), and Mozilla VPN. Rust’s focus on safety and performance aligns well with Mozilla’s commitment to building secure and efficient software.
- Dropbox: Dropbox utilizes Rust for performance-critical components of its infrastructure, such as networking and storage systems. Rust’s memory safety features and concurrency model help ensure the reliability and efficiency of Dropbox’s services.
- Cloudflare: Cloudflare employs Rust in projects like Cloudflare Workers, a serverless compute platform, and Quiche, an implementation of the QUIC transport protocol. Rust’s performance and safety features make it well-suited for building high-performance, network-oriented applications.
- AWS (Amazon Web Services): AWS has incorporated Rust into its services, including the Firecracker microVM platform and the Bottlerocket Linux-based operating system for containerized workloads. Rust’s lightweight, secure, and efficient nature makes it a natural fit for cloud infrastructure projects.
Golang (Go):
Golang (Go) has gained popularity for its simplicity, efficiency, and built-in support for concurrency. Some companies that use Go in their software development include:
- Google: Unsurprisingly, Google, the creator of Go, uses the language extensively in various internal projects and services. Go powers critical components of Google’s infrastructure, including the Kubernetes container orchestration platform and the Istio service mesh.
- Uber: Uber utilizes Go for building scalable backend services and microservices that power its ride-hailing and food delivery platforms. Go’s simplicity and efficiency make it well-suited for handling high volumes of concurrent requests in distributed systems.
- Netflix: Netflix leverages Go for building tools and services that support its streaming platform and content delivery network. Go’s concurrency model and standard library facilitate the development of scalable and reliable systems at Netflix’s scale.
- Docker: Docker, the popular containerization platform, is written primarily in Go. Go’s performance and concurrency features enable Docker to provide fast and efficient container management and orchestration capabilities.
C++:
C++ remains a popular choice for performance-critical applications and systems programming. Some companies that use C++ extensively include:
- Microsoft: Microsoft employs C++ in numerous projects, including the Windows operating system, Microsoft Office suite, and Xbox gaming platform. C++’s performance and control make it suitable for developing low-level components and high-performance applications.
- Facebook: Facebook uses C++ in its backend infrastructure and performance-critical services, such as the Messenger messaging platform and the HHVM (HipHop Virtual Machine) runtime for PHP. C++’s efficiency and control enable Facebook to handle massive amounts of user data and requests.
- Adobe: Adobe relies on C++ for building flagship products like Adobe Photoshop, Adobe Illustrator, and Adobe Premiere Pro. C++’s performance and flexibility allow Adobe to deliver professional-grade software with advanced features and capabilities.
- Electronic Arts (EA): EA develops video games using C++ for platforms like PC, consoles, and mobile devices. C++’s performance and control enable EA to create immersive gaming experiences with stunning graphics and responsive gameplay.
These examples highlight the diverse range of companies and industries that rely on Rust, Golang (Go), and C++ for building software and services. Whether it’s for web development, cloud infrastructure, system programming, or game development, each language offers unique advantages that cater to different use cases and requirements.