ML.NET is an open-source, cross-platform machine learning framework developed by Microsoft. It allows developers to integrate machine learning models into their .NET applications, enabling them to leverage the power of machine learning for tasks such as classification, regression, clustering, recommendation, and more. ML.NET is designed to be accessible to both novice and experienced developers, providing a user-friendly API and seamless integration with the broader .NET ecosystem.
Key Features of ML.NET:
- Cross-Platform Support:
ML.NET is designed to work across various platforms, including Windows, macOS, and Linux, providing flexibility for developers. - Integration with .NET Ecosystem:
ML.NET seamlessly integrates with other .NET libraries and frameworks, making it easy to incorporate machine learning capabilities into existing applications. - User-Friendly API:
ML.NET provides a simple and intuitive API, allowing developers to build and train machine learning models with ease. It follows a code-first approach, enabling developers to define models using C# code. - Wide Range of Algorithms:
ML.NET supports a variety of machine learning algorithms for different tasks. It includes algorithms for classification, regression, clustering, anomaly detection, and more. - Custom Model Training:
Developers can train custom machine learning models using their own data. ML.NET supports both supervised and unsupervised learning scenarios. - Model Explainability:
ML.NET includes features for explaining and interpreting machine learning models, helping developers understand how models make predictions. - Integration with TensorFlow and ONNX:
ML.NET can consume models trained in popular frameworks like TensorFlow and ONNX (Open Neural Network Exchange), allowing developers to use pre-trained models in their applications. - Scalability:
ML.NET is designed to scale with the needs of applications, whether they are small projects or large-scale enterprise solutions.
Getting Started with ML.NET:
To get started with ML.NET, developers typically follow these steps:
- Install ML.NET:
Use the NuGet Package Manager or the .NET CLI to install the ML.NET NuGet package. - Define Data Classes:
Define classes that represent the structure of the input data and the output predictions. - Load and Prepare Data:
Load and prepare the training and testing datasets for model training. - Define a Model:
Choose a machine learning algorithm and define the model using the ML.NET API. - Train the Model:
Train the model using the training dataset. - Evaluate and Use the Model:
Evaluate the model’s performance on a separate testing dataset. Once satisfied, use the model to make predictions in production.
Example of ML.NET Code:
Here’s a simple example of using ML.NET for sentiment analysis:
using Microsoft.ML; using Microsoft.ML.Data; public class SentimentData { [LoadColumn(0)] public bool Sentiment; [LoadColumn(1)] public string Text; } public class SentimentPrediction { [ColumnName("PredictedLabel")] public bool Prediction; } class Program { static void Main() { var mlContext = new MLContext(); // Load data var data = mlContext.Data.LoadFromTextFile<SentimentData>("sentiment_data.csv", separatorChar: ','); // Define data processing pipeline var pipeline = mlContext.Transforms.Text.FeaturizeText("Features", "Text") .Append(mlContext.Transforms.Conversion.MapValueToKey("Label")) .Append(mlContext.Transforms.Conversion.MapKeyToValue("PredictedLabel")) .Append(mlContext.Transforms.Conversion.MapValueToKey("PredictedLabel")); // Choose a machine learning algorithm var trainer = mlContext.Transforms.Conversion.MapKeyToValue("PredictedLabel") .Append(mlContext.Transforms.Conversion.MapValueToKey("Label")) .Append(mlContext.Transforms.Conversion.MapKeyToValue("Label")) .Append(mlContext.BinaryClassification.Trainers.SdcaLogisticRegression()); // Create and train the model var model = pipeline.Append(trainer).Fit(data); // Make predictions var sentiment = new SentimentData { Text = "I love ML.NET!" }; var prediction = mlContext.Model.MakePredictionFunction<SentimentData, SentimentPrediction>(model).Predict(sentiment); Console.WriteLine($"Predicted sentiment: {prediction.Prediction}"); } }
In this example, we load sentiment data, define a data processing pipeline, choose a binary classification algorithm, train the model, and then use the trained model to predict the sentiment of new text.
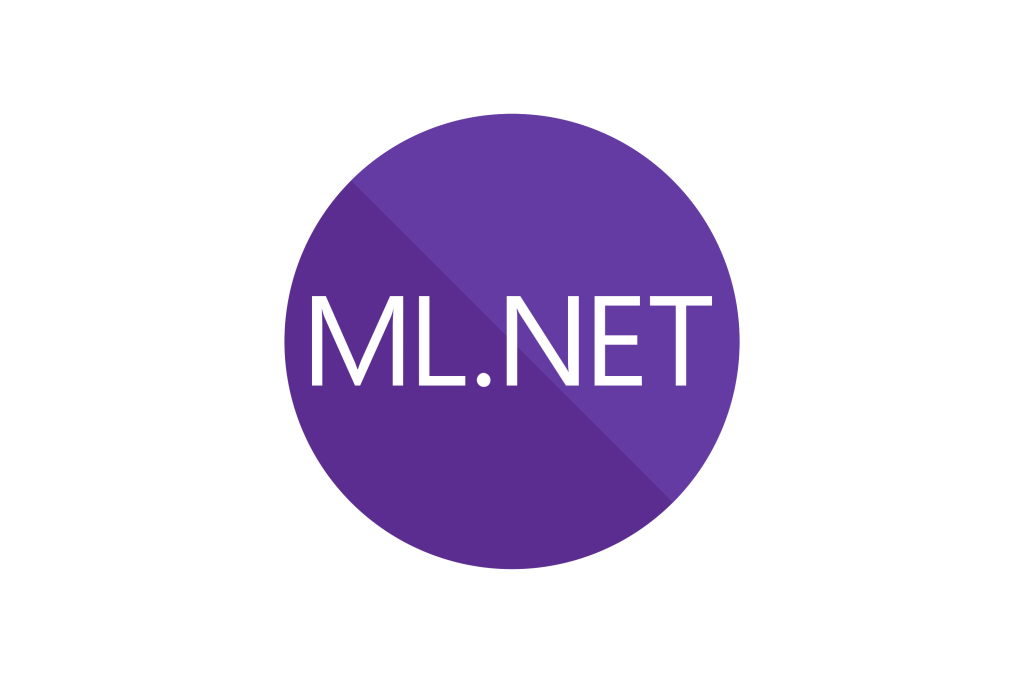
Conclusion:
ML.NET provides a powerful and accessible framework for incorporating machine learning capabilities into C# applications. Whether you’re a beginner looking to explore machine learning or an experienced developer integrating ML into a production system, ML.NET offers a versatile and user-friendly platform for building intelligent applications.