Introduction
Node.js, with its asynchronous capabilities and powerful ecosystem, is an excellent choice for building applications that involve file uploads. In this article, we’ll explore how to create a Node.js application that allows users to upload multiple files. We’ll use the popular Express framework and the multer
middleware to handle file uploads.
Setting Up the Project
Before we begin, make sure you have Node.js installed on your machine. Follow these steps to set up a new project:
- Create a new directory for your project and navigate into it using the terminal:
mkdir file-upload-app cd file-upload-app
- Initialize a new Node.js project:
npm init -y
- Install Express and Multer:
npm install express multer
- Create the main file for your application, e.g.,
app.js
.
Implementing File Uploads with Multer and Express
Now, let’s set up our Express application to handle file uploads.
1. Importing Modules
In your app.js
file, start by importing the necessary modules:
const express = require('express'); const multer = require('multer'); const path = require('path'); const app = express(); const port = 3000;
2. Configuring Multer for File Uploads
Configure Multer to specify where the uploaded files should be stored and how they should be named. Add the following code:
const storage = multer.diskStorage({ destination: function (req, file, cb) { cb(null, 'uploads/'); // Files will be saved in the 'uploads' directory }, filename: function (req, file, cb) { cb(null, Date.now() + path.extname(file.originalname)); // Unique file names }, }); const upload = multer({ storage: storage });
3. Creating File Upload Endpoints
Create the endpoints for handling file uploads. We’ll create both a single-file upload and a multiple-file upload endpoint:
app.post('/upload-single', upload.single('file'), (req, res) => { res.json({ message: 'File uploaded successfully', file: req.file }); }); app.post('/upload-multiple', upload.array('files', 5), (req, res) => { res.json({ message: 'Files uploaded successfully', files: req.files }); });
In the above code:
/upload-single
is configured to handle a single file upload./upload-multiple
is configured to handle multiple files with a maximum of 5 files.
4. Setting Up Static File Serving
To serve the uploaded files, add the following code to serve the ‘uploads’ directory as a static folder:
app.use('/uploads', express.static('uploads'));
5. Starting the Server
Finally, start the Express server:
app.listen(port, () => { console.log(`Server listening at http://localhost:${port}`); });
Testing File Uploads
- Run your Node.js application:
node app.js
- Use tools like Postman or HTML forms to test the file upload endpoints:
- For single-file upload, send a POST request to
http://localhost:3000/upload-single
with a form containing a file input named ‘file’. - For multiple-file upload, send a POST request to
http://localhost:3000/upload-multiple
with a form containing multiple file inputs named ‘files’.
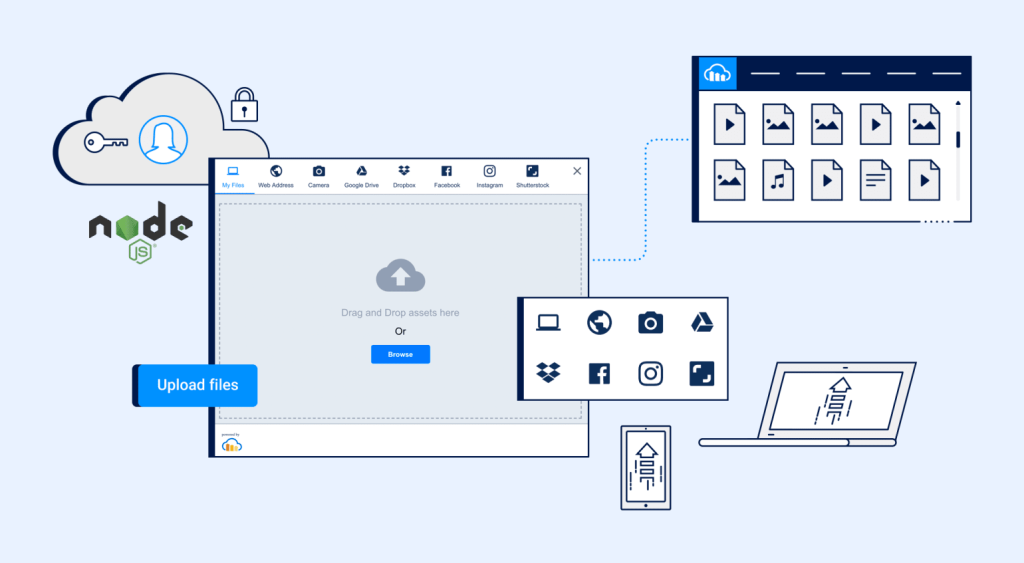
You have now successfully implemented file uploads with Node.js, Express, and Multer. This basic setup can be extended to include additional features such as file type validation, handling large files, and integrating with cloud storage services based on your application requirements.