Introduction
Using a framework in any programming language, including Go, can offer several advantages that simplify development, enhance productivity, and improve the overall quality of your software. While Go is known for its simplicity and ease of use, frameworks provide a structured way to build applications. Here are some reasons why you might consider using a Go framework:
Rapid Development:
- Frameworks often come with built-in tools and features that streamline common development tasks. This can lead to faster development cycles and quicker time-to-market for your applications.
Code Organization:
- Frameworks typically provide a structure for organizing code. This helps maintain a clean and modular codebase, making it easier to understand, extend, and maintain over time. A well-organized codebase is crucial, especially as projects grow in size and complexity.
Convention over Configuration:
- Many Go frameworks follow the “convention over configuration” principle, reducing the need for developers to make explicit configuration decisions. This can lead to more consistent project structures and less boilerplate code.
Middleware Support:
- Go frameworks often include middleware support for common tasks such as authentication, logging, and request processing. This allows developers to plug in and configure pre-built components easily, saving time and effort.
Routing and URL Handling:
- Frameworks often come with robust routing mechanisms that simplify URL handling and endpoint routing. This is crucial for building RESTful APIs and web applications with clean and maintainable URL structures.
Database Abstraction:
- Many Go frameworks provide abstractions for working with databases, making it easier to perform database operations without directly dealing with low-level details. This can improve code readability and reduce the chance of SQL injection vulnerabilities.
Testing Support:
- Frameworks often include tools and conventions for testing. This encourages developers to write tests for their code, contributing to the overall stability and reliability of the application.
Security Features:
- Go frameworks may come with built-in security features or recommendations, helping developers follow best practices for securing their applications. This is especially important when dealing with user authentication, authorization, and data validation.
Community Support:
- Popular Go frameworks have active communities, which means you can benefit from a wealth of shared knowledge, resources, and third-party plugins. This community support can be valuable for troubleshooting, learning, and staying up-to-date with best practices.
Scalability:
- Some frameworks are designed with scalability in mind. They provide patterns and practices that can be followed to build scalable applications. This is important as your application grows and needs to handle increased traffic and data.
Popular Go frameworks include Gin, Echo, Revel, and Buffalo, each with its own set of features and strengths. When choosing a framework, consider your project requirements, the learning curve, and how well the framework aligns with your development philosophy and goals. While frameworks can be beneficial, it’s essential to evaluate whether the additional abstraction layers fit the specific needs of your project.
Here are five popular Go (Golang) frameworks, each with its own strengths and use cases:
Gin:
- Reasons:
- Performance: Gin is known for its fast performance, making it suitable for building high-performance RESTful APIs.
- Minimalistic Design: Gin follows a minimalistic design philosophy, keeping the codebase small and simple. This appeals to developers who prefer lightweight frameworks.
- Middleware Support: It provides a robust middleware system, allowing developers to easily integrate various functionalities into their applications.
- Router: Gin’s router is efficient and supports RESTful routing patterns, making it easy to define and manage routes.
Echo:
- Reasons:
- High Performance: Echo is designed for speed and efficiency, making it well-suited for building scalable web applications and APIs.
- Lightweight: Similar to Gin, Echo follows a minimalistic approach, offering just what you need without unnecessary complexity.
- Extensibility: Echo is built with extensibility in mind, allowing developers to easily add middleware and customize components to fit their specific requirements.
- WebSocket Support: It includes built-in support for WebSocket, making it a good choice for real-time applications.
Revel:
- Reasons:
- Full-Stack Framework: Revel is a full-stack framework, providing features for both the frontend and backend. It includes a templating engine, ORM, and built-in testing tools.
- Convention over Configuration: Revel follows the convention over configuration paradigm, reducing the need for explicit configuration.
- Hot Code Reload: Revel supports hot code reloading during development, allowing developers to see changes instantly without restarting the server.
- Built-in Testing: It comes with comprehensive testing support, helping developers maintain code quality through unit and integration testing.
Buffalo:
- Reasons:
- Opinionated Framework: Buffalo is an opinionated web development framework that provides a set of conventions and best practices, making it easy to get started quickly.
- Code Generation: Buffalo includes a powerful code generation tool that automates many common development tasks, saving time and reducing boilerplate code.
- Modularity: It follows a modular design, allowing developers to use only the components they need, making it flexible for various project sizes.
- Asset Pipeline: Buffalo comes with an asset pipeline for managing frontend assets, making it convenient for handling JavaScript, CSS, and other static files.
Fiber:
- Reasons:
- Performance: Fiber is built with a focus on speed and low memory consumption, making it a performant choice for high-concurrency applications.
- Express-Like Syntax: Fiber’s syntax is inspired by Express.js, making it familiar to developers who have experience with Node.js.
- Middleware Support: It provides a middleware system for easily adding functionalities to the application, enhancing its extensibility.
- Fast JSON Rendering: Fiber includes a fast JSON renderer, making it suitable for building APIs that require efficient JSON processing.
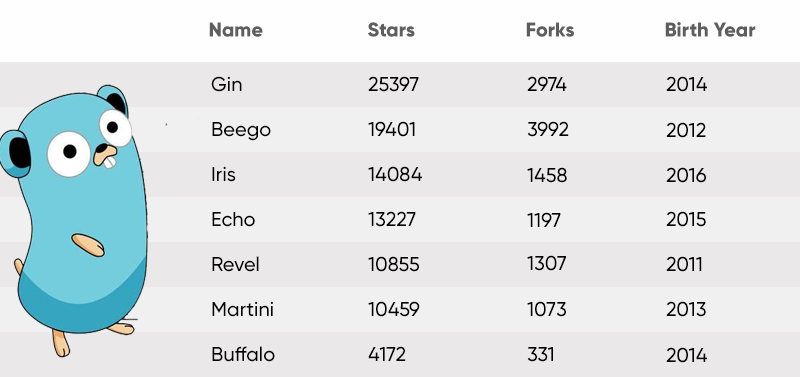
When choosing a framework, consider factors such as your project requirements, the framework’s learning curve, and how well it aligns with your development preferences. Additionally, the popularity and features of frameworks can evolve, so it’s a good practice to check for the latest information and community feedback.
Exploring Top 5 Golang Frameworks: Gin, Echo, Revel, Buffalo, and Fiber
Choosing the right framework is crucial when developing applications in Go (Golang). In this article, we’ll delve into five popular Golang frameworks: Gin, Echo, Revel, Buffalo, and Fiber. Each framework has its own strengths and is suitable for different use cases. Let’s explore them along with some sample code snippets to highlight their features.
Gin
Gin is a high-performance web framework known for its minimalistic design and efficiency. It’s particularly popular for building RESTful APIs due to its speed and simplicity.
package main import "github.com/gin-gonic/gin" func main() { router := gin.Default() router.GET("/hello", func(c *gin.Context) { c.JSON(200, gin.H{"message": "Hello, Gin!"}) }) router.Run(":8080") }
Features:
- Fast routing with minimal memory footprint.
- Robust middleware support.
- RESTful routing patterns.
Echo
Echo is a high-performance framework designed for speed and efficiency. It’s lightweight and well-suited for building scalable web applications and APIs.
package main import "github.com/labstack/echo/v4" func main() { e := echo.New() e.GET("/hello", func(c echo.Context) error { return c.JSON(200, map[string]string{"message": "Hello, Echo!"}) }) e.Start(":8080") }
Features:
- High performance with minimal overhead.
- Lightweight and extensible.
- Built-in support for WebSocket.
Revel
Revel is a full-stack framework providing conventions and tools for both frontend and backend development. It’s opinionated, making it easy to get started quickly.
package controllers import "github.com/revel/revel" type App struct { *revel.Controller } func (c App) Index() revel.Result { return c.RenderText("Hello, Revel!") }
Features:
- Full-stack framework with built-in templating and ORM.
- Hot code reloading during development.
- Built-in testing tools.
Buffalo
Buffalo is an opinionated web development framework that follows conventions and provides a powerful code generation tool. It’s modular and adaptable for projects of various sizes.
buffalo new myapp cd myapp buffalo dev
Features:
- Opinionated framework with conventions.
- Code generation for common tasks.
- Modular design for flexibility.
Fiber
Fiber is a fast and lightweight framework inspired by Express.js. It is designed for high-concurrency applications and comes with an Express-like syntax.
package main import "github.com/gofiber/fiber/v2" func main() { app := fiber.New() app.Get("/hello", func(c *fiber.Ctx) error { return c.JSON(map[string]string{"message": "Hello, Fiber!"}) }) app.Listen(":8080") }
Features:
- Fast and memory-efficient.
- Express-like syntax for familiarity.
- Fast JSON rendering.
Conclusion:
The choice of a Golang framework depends on your project’s requirements, your development preferences, and the specific features offered by each framework. Whether you prioritize speed, simplicity, or a full-stack approach, these frameworks provide a solid foundation for building scalable and efficient Go applications. Experiment with them to find the one that best suits your needs and coding style.
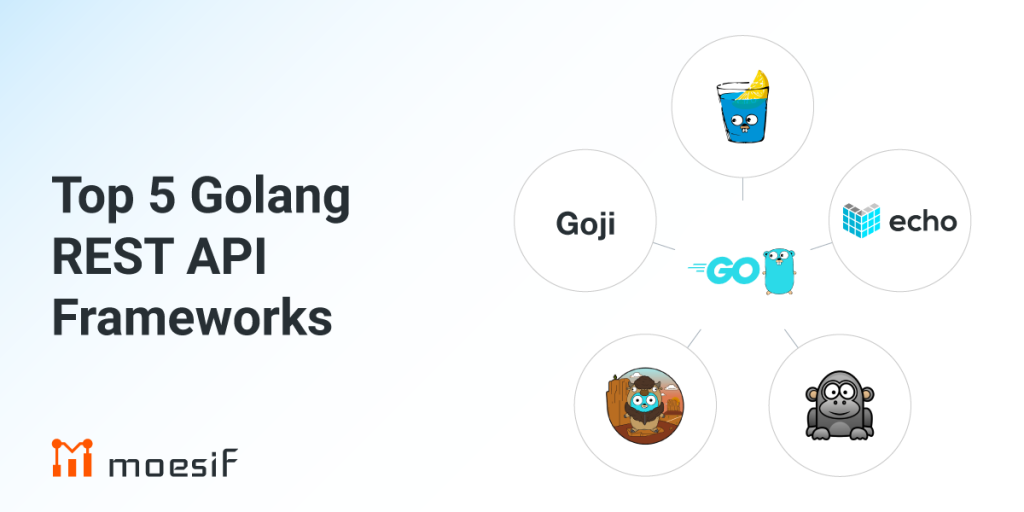