Introduction
C# (C sharp) is a versatile and powerful programming language that serves as the foundation for many types of software development. Whether you’re a beginner or an experienced programmer exploring a new language, understanding the fundamentals of C# is essential. In this article, we’ll delve into the core concepts of C# and provide code examples to help you grasp its fundamentals.
- Hello World in C#: Let’s start with the traditional “Hello World” program to introduce you to the basic syntax of C#.
using System; class Program { static void Main() { Console.WriteLine("Hello, World!"); } }
In this simple program, using System;
includes the necessary namespace for console input and output. The class Program
contains the Main
method, which is the entry point of the program. The Console.WriteLine
statement prints the “Hello, World!” message to the console.
- Variables and Data Types: C# supports various data types, including integers, floating-point numbers, characters, strings, and more. Here’s an example demonstrating variable declaration and initialization:
int age = 25; double height = 1.75; char gender = 'M'; string name = "John Doe"; Console.WriteLine($"Name: {name}, Age: {age}, Height: {height}, Gender: {gender}");
In this example, we declare variables of different data types and use string interpolation to display their values.
- Control Flow Statements: C# provides several control flow statements, such as
if
,else
,switch
,for
,while
, anddo-while
. Here’s an example usingif
andelse
:
int num = 10; if (num > 0) { Console.WriteLine("Positive number"); } else if (num < 0) { Console.WriteLine("Negative number"); } else { Console.WriteLine("Zero"); }
This code snippet checks the value of num
and prints whether it is positive, negative, or zero.
- Arrays and Loops: Arrays are fundamental in C#, and loops allow you to iterate through them. Here’s an example using an array and a
for
loop:
int[] numbers = { 1, 2, 3, 4, 5 }; for (int i = 0; i < numbers.Length; i++) { Console.Write(numbers[i] + " "); }
This code initializes an array of integers and uses a for
loop to print each element.
- Functions and Methods: Functions (or methods) allow you to encapsulate code for reuse. Here’s a simple function that calculates the square of a number:
static int Square(int x) { return x * x; } // Example usage: int result = Square(4); Console.WriteLine("Square of 4 is: " + result);
In this example, the Square
function takes an integer parameter x
and returns its square.
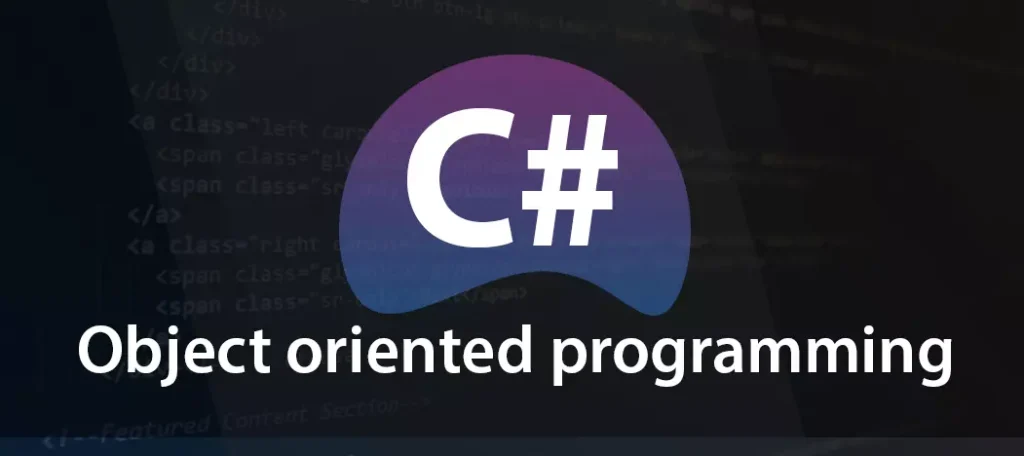
Conclusion
These code examples cover some of the fundamental concepts in C#. As you continue to explore the language, delve into more advanced topics like classes, inheritance, and exception handling. Mastering the basics lays a solid foundation for becoming a proficient C# developer, enabling you to tackle a wide range of programming challenges.