Delegates and Events: Enhancing Code Flexibility
In C#, delegates provide a powerful mechanism for creating type-safe function pointers, enabling more flexible and dynamic programming. Events, closely tied to delegates, facilitate the implementation of the observer pattern. Consider the following code snippet showcasing delegates and events:
using System; // Define a delegate delegate void MyDelegate(string message); class EventPublisher { // Declare an event based on the delegate public event MyDelegate Notify; public void DoSomething() { // Invoke the event Notify?.Invoke("Something happened!"); } } class EventSubscriber { public void Subscribe(EventPublisher publisher) { // Attach a method to the event publisher.Notify += DisplayMessage; } void DisplayMessage(string message) { Console.WriteLine($"Received message: {message}"); } } class Program { static void Main() { EventPublisher publisher = new EventPublisher(); EventSubscriber subscriber = new EventSubscriber(); // Subscribe the subscriber to the publisher's event subscriber.Subscribe(publisher); // Trigger the event publisher.DoSomething(); } }
This example demonstrates the usage of delegates and events. The MyDelegate
delegate defines the signature for methods that can be subscribed to the event. The EventPublisher
class triggers the event, and the EventSubscriber
class subscribes to it, reacting to the event when it occurs.
Collections and Generics: Enhancing Data Management
Collections and generics are fundamental in C# for efficient data management. Generics enable the creation of type-safe and reusable code, while collections provide dynamic storage for various data structures. Here’s a brief example showcasing a generic list:
using System; using System.Collections.Generic; class Program { static void Main() { // Create a generic list of integers List<int> numbers = new List<int> { 1, 2, 3, 4, 5 }; // Add an element to the list numbers.Add(6); // Iterate through the list foreach (var number in numbers) { Console.Write(number + " "); } } }
In this example, the List<int>
is a generic collection that holds integers. The program adds an element to the list and then iterates through it, printing each element.
Error Handling and Exceptions: Ensuring Code Resilience
C# supports robust error handling through exceptions. Developers can catch and handle errors gracefully, improving the reliability of their applications. Consider the following code snippet demonstrating exception handling:
using System; class Program { static void Main() { try { // Potential code that may throw an exception int result = Divide(10, 0); // This line won't be executed if an exception occurs Console.WriteLine("Result: " + result); } catch (DivideByZeroException ex) { Console.WriteLine("Error: " + ex.Message); } } static int Divide(int numerator, int denominator) { if (denominator == 0) { throw new DivideByZeroException("Denominator cannot be zero."); } return numerator / denominator; } }
In this example, the Divide
method throws a DivideByZeroException
if the denominator is zero. The try-catch
block in the Main
method catches and handles the exception, preventing the program from crashing.
Multithreading: Enhancing Performance with Concurrent Execution
Multithreading is crucial for optimizing performance in modern applications. C# provides robust support for multithreading through the Thread
class and other synchronization mechanisms. Here’s a simplified example illustrating the basics:
using System; using System.Threading; class Program { static void Main() { // Create a new thread and start it Thread newThread = new Thread(PrintNumbers); newThread.Start(); // Main thread continues its own execution for (int i = 0; i < 5; i++) { Console.Write("Main thread: " + i + " "); } } static void PrintNumbers() { for (int i = 0; i < 5; i++) { Console.Write("Secondary thread: " + i + " "); } } }
In this example, the program creates a new thread (newThread
) that executes the PrintNumbers
method concurrently with the main thread. This illustrates the basics of multithreading in C#.
Advanced Libraries: Leveraging Networking and UI Development
C# offers advanced libraries that facilitate networking and UI development. The following example showcases a basic HTTP client using the HttpClient
class for networking:
using System; using System.Net.Http; using System.Threading.Tasks; class Program { static async Task Main() { // Create an instance of HttpClient using (HttpClient client = new HttpClient()) { // Make a GET request to a URL HttpResponseMessage response = await client.GetAsync("https://jsonplaceholder.typicode.com/todos/1"); // Check if the request was successful if (response.IsSuccessStatusCode) { // Read and display the content string content = await response.Content.ReadAsStringAsync(); Console.WriteLine(content); } } } }
In this example, the program uses the HttpClient
class to make an asynchronous GET request to a URL, demonstrating basic networking capabilities.
For UI development, C# offers libraries like Windows Presentation Foundation (WPF) or Windows Forms. These libraries empower developers to create rich and interactive user interfaces for desktop applications.
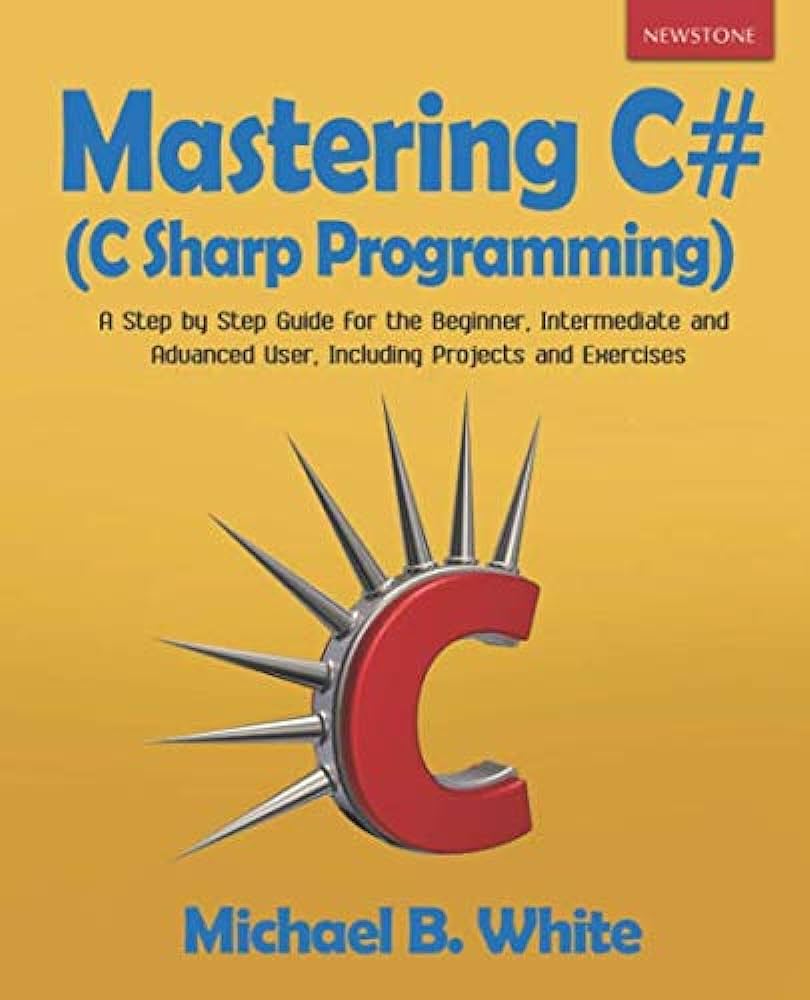
In conclusion, mastering these C# essentials, from fundamental concepts like delegates and collections to advanced features like multithreading and advanced libraries, equips developers with the tools needed to build robust and scalable applications. Continuous exploration and hands-on practice are key to becoming proficient in C# development.