Introduction:
Microservices architecture has become a popular approach for developing scalable and modular applications. Golang, with its efficiency and concurrency support, is well-suited for building microservices. In this article, we’ll explore how to build microservices using five prominent Golang frameworks: Gin, Echo, Revel, Buffalo, and Fiber. We’ll cover essential concepts and provide sample code snippets to guide you through the process.
Gin: Creating a Microservice with Gin:
package main import "github.com/gin-gonic/gin" func main() { router := gin.Default() router.GET("/api/user/:id", func(c *gin.Context) { // Fetch user details from the database based on ID // Return user information as JSON }) // Implement other microservices... router.Run(":8080") }
Features:
- Lightweight and fast routing.
- Robust middleware support.
- Ideal for building RESTful microservices.
Echo: Creating a Microservice with Echo:
package main import "github.com/labstack/echo/v4" func main() { e := echo.New() e.GET("/api/user/:id", func(c echo.Context) error { // Fetch user details from the database based on ID // Return user information as JSON return nil }) // Implement other microservices... e.Start(":8080") }
Features:
- High-performance with minimal overhead.
- Lightweight and extensible.
- Ideal for building RESTful microservices.
Revel: Creating a Microservice with Revel:
package controllers import "github.com/revel/revel" type UserController struct { *revel.Controller } func (c UserController) GetUser(id int) revel.Result { // Fetch user details from the database based on ID // Return user information as JSON return c.RenderJSON(map[string]interface{}{}) } // Implement other microservices...
Features:
- Full-stack framework with built-in modules.
- Ideal for building both frontend and backend microservices.
- Convention over configuration.
Buffalo: Creating a Microservice with Buffalo:
package actions import "github.com/gobuffalo/buffalo" type UserController struct { buffalo.Resource } func (v UserResource) Show() error { // Fetch user details from the database based on ID // Return user information as JSON return nil } // Implement other microservices...
Features:
- Opinionated framework with code generation.
- Ideal for building full-stack microservices.
- Built-in support for web services.
Fiber: Creating a Microservice with Fiber:
package main import "github.com/gofiber/fiber/v2" func main() { app := fiber.New() app.Get("/api/user/:id", func(c *fiber.Ctx) error { // Fetch user details from the database based on ID // Return user information as JSON return nil }) // Implement other microservices... app.Listen(":8080") }
Features:
- Fast and memory-efficient.
- Express-like syntax for familiarity.
- Ideal for building high-concurrency microservices.
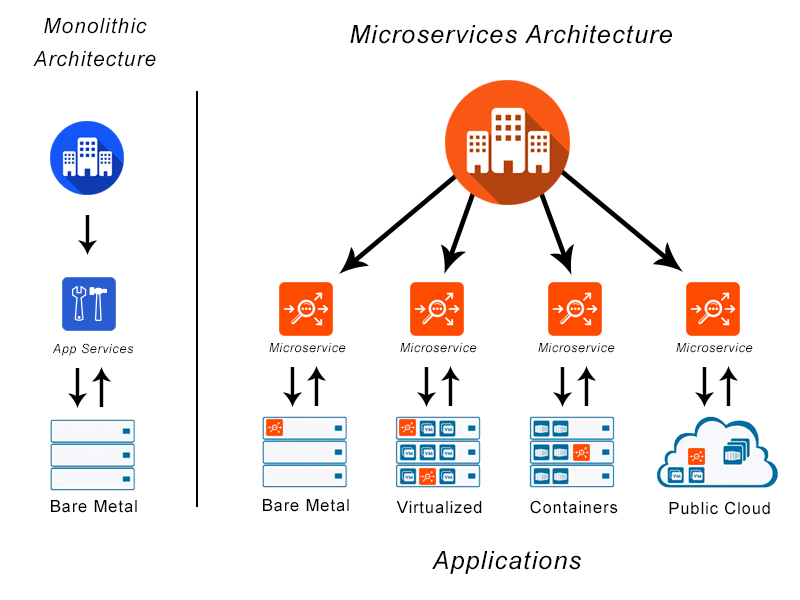
Conclusion
Building microservices with Golang frameworks offers a flexible and efficient way to create scalable and modular applications. Whether you choose Gin, Echo, Revel, Buffalo, or Fiber, each framework provides tools and features to simplify the development of microservices. Customize the provided code snippets according to your microservices’ logic and requirements, and leverage the strengths of each framework for optimal results. Happy microservice development!