Networking programming is a critical aspect of software development, enabling applications to communicate over networks and share data. C# provides robust tools and libraries for building networking applications, whether you’re creating a client-server architecture, implementing communication between devices, or accessing remote services. In this article, we’ll explore the fundamentals of networking programming with C#.
Understanding Sockets in C#
At the core of networking programming lies the concept of sockets. A socket is a communication endpoint that allows processes on different devices to exchange data. C# provides the Socket
class in the System.Net.Sockets
namespace, offering a powerful and flexible interface for network communication.
Example: Creating a Simple TCP Server
Let’s start by creating a basic TCP server in C#. The server listens for incoming connections and echoes back any data it receives.
using System; using System.Net; using System.Net.Sockets; using System.Text; class TCPServer { static void Main() { TcpListener tcpListener = new TcpListener(IPAddress.Any, 8080); tcpListener.Start(); Console.WriteLine("Server is listening on port 8080..."); while (true) { TcpClient client = tcpListener.AcceptTcpClient(); HandleClient(client); } } static void HandleClient(TcpClient client) { NetworkStream stream = client.GetStream(); byte[] buffer = new byte[1024]; int bytesRead; while ((bytesRead = stream.Read(buffer, 0, buffer.Length)) > 0) { string data = Encoding.ASCII.GetString(buffer, 0, bytesRead); Console.WriteLine($"Received: {data}"); byte[] response = Encoding.ASCII.GetBytes($"Server received: {data}"); stream.Write(response, 0, response.Length); } client.Close(); } }
In this example, the server listens on port 8080 for incoming TCP connections. When a client connects, the server reads data from the client, echoes it back, and then closes the connection.
Building a TCP Client
To complete the example, let’s create a simple TCP client in C# that connects to the server and sends a message.
using System; using System.Net.Sockets; using System.Text; class TCPClient { static void Main() { TcpClient client = new TcpClient("127.0.0.1", 8080); NetworkStream stream = client.GetStream(); string message = "Hello, server!"; byte[] data = Encoding.ASCII.GetBytes(message); stream.Write(data, 0, data.Length); byte[] responseBuffer = new byte[1024]; int bytesRead = stream.Read(responseBuffer, 0, responseBuffer.Length); string response = Encoding.ASCII.GetString(responseBuffer, 0, bytesRead); Console.WriteLine($"Server response: {response}"); client.Close(); } }
This client connects to the server’s IP address (“127.0.0.1” for localhost) and port 8080. It sends a message to the server, reads the server’s response, and prints it.
Asynchronous Socket Programming
For scalable and responsive applications, especially in scenarios with many simultaneous connections, asynchronous socket programming is crucial. C# provides the SocketAsyncEventArgs
class and the SocketAsyncOperation
enumeration for efficient asynchronous socket operations.
Example: Asynchronous TCP Server
Let’s modify our TCP server to use asynchronous socket operations.
using System; using System.Net; using System.Net.Sockets; using System.Text; using System.Threading.Tasks; class AsyncTCPServer { static async Task Main() { TcpListener tcpListener = new TcpListener(IPAddress.Any, 8080); tcpListener.Start(); Console.WriteLine("Server is listening on port 8080..."); while (true) { TcpClient client = await tcpListener.AcceptTcpClientAsync(); _ = HandleClientAsync(client); } } static async Task HandleClientAsync(TcpClient client) { using NetworkStream stream = client.GetStream(); byte[] buffer = new byte[1024]; int bytesRead; while ((bytesRead = await stream.ReadAsync(buffer, 0, buffer.Length)) > 0) { string data = Encoding.ASCII.GetString(buffer, 0, bytesRead); Console.WriteLine($"Received: {data}"); byte[] response = Encoding.ASCII.GetBytes($"Server received: {data}"); await stream.WriteAsync(response, 0, response.Length); } client.Close(); } }
This modified server uses asynchronous methods for accepting clients and handling client communication, ensuring efficient use of resources and responsiveness.
Building a UDP Server and Client
In addition to TCP, C# supports UDP (User Datagram Protocol), a connectionless communication protocol suitable for scenarios where real-time communication is essential but a reliable connection is not. Let’s explore a simple UDP server and client example:
UDP Server
using System; using System.Net; using System.Net.Sockets; using System.Text; class UDPServer { static void Main() { UdpClient udpListener = new UdpClient(8080); Console.WriteLine("Server is listening on port 8080..."); IPEndPoint clientEndpoint = new IPEndPoint(IPAddress.Any, 0); while (true) { byte[] receivedData = udpListener.Receive(ref clientEndpoint); string message = Encoding.ASCII.GetString(receivedData); Console.WriteLine($"Received from {clientEndpoint}: {message}"); // Echo back to the client udpListener.Send(receivedData, receivedData.Length, clientEndpoint); } } }
UDP Client
using System; using System.Net; using System.Net.Sockets; using System.Text; class UDPClient { static void Main() { UdpClient udpClient = new UdpClient(); IPEndPoint serverEndpoint = new IPEndPoint(IPAddress.Parse("127.0.0.1"), 8080); string message = "Hello, UDP Server!"; byte[] data = Encoding.ASCII.GetBytes(message); udpClient.Send(data, data.Length, serverEndpoint); byte[] receivedData = udpClient.Receive(ref serverEndpoint); string response = Encoding.ASCII.GetString(receivedData); Console.WriteLine($"Server response: {response}"); } }
These UDP examples showcase simple communication between a server and a client without the need for a continuous connection.
Security Considerations
In real-world networking applications, security is paramount. Always consider using secure protocols (e.g., HTTPS) and implement encryption, authentication, and authorization mechanisms to protect sensitive data.
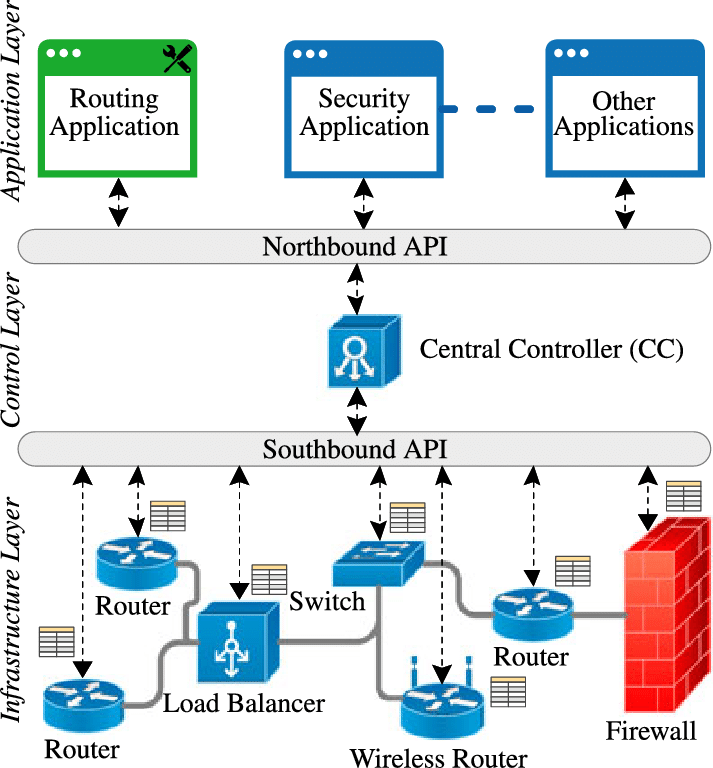
Conclusion
Networking programming with C# provides a robust foundation for building connected applications. From basic TCP communication to asynchronous socket programming and UDP communication, C# offers a versatile set of tools for various networking scenarios. As you explore networking in C#, continue to deepen your understanding and consider the security implications of your applications. With these skills, you’ll be well-equipped to develop efficient and reliable networked solutions.