Introduction
Node.js has become a go-to platform for building scalable and high-performance web applications. Its non-blocking, event-driven architecture is well-suited for handling concurrent connections and real-time applications. To enhance the development process, various frameworks have emerged, providing developers with tools and structures to streamline the creation of robust applications. Here are the top 5 Node.js frameworks that stand out in the web development landscape.
1. Express.js
Express.js is undoubtedly the most popular and widely used Node.js framework. It is a minimal and flexible framework that provides a robust set of features for web and mobile applications. With a minimalist approach, Express allows developers to build APIs and web applications quickly. It offers middleware support, routing, template engines, and a vibrant ecosystem of extensions through npm (Node Package Manager).
Key Features:
- Minimal and unopinionated design.
- Middleware support for extending functionality.
- Efficient routing system.
- Widely adopted, with a large and active community.
Sample Code:
const express = require('express'); const app = express(); app.get('/', (req, res) => { res.send('Hello, Express!'); }); app.listen(3000, () => { console.log('Server running on port 3000'); });
2. Koa.js
Developed by the creators of Express.js, Koa.js is a more modern and lightweight framework. It focuses on being more expressive, robust, and scalable by utilizing ECMAScript features like async/await. Koa provides a clean and modular structure for building web applications, making it a suitable choice for developers who prefer a more modern JavaScript experience.
Key Features:
- Lightweight and modular.
- Utilizes async/await for cleaner code.
- Improved error handling.
- Generators for better middleware.
Sample Code:
const Koa = require('koa'); const app = new Koa(); app.use(async ctx => { ctx.body = 'Hello, Koa!'; }); app.listen(3000, () => { console.log('Server running on port 3000'); });
3. Nest.js
Nest.js is a progressive Node.js framework for building efficient, scalable server-side applications. It embraces the use of TypeScript and follows the modular architecture inspired by Angular. Nest.js provides a set of well-organized modules, decorators, and a powerful dependency injection system. It is particularly well-suited for building enterprise-level applications with a structured and maintainable codebase.
Key Features:
- Uses TypeScript for strong typing.
- Modular architecture with decorators.
- Built-in support for GraphQL and WebSockets.
- Dependency injection for managing components.
Sample Code:
import { NestFactory } from '@nestjs/core'; import { AppModule } from './app.module'; async function bootstrap() { const app = await NestFactory.create(AppModule); await app.listen(3000); } bootstrap();
4. Sails.js
Sails.js is a full-featured MVC (Model-View-Controller) framework for building data-driven APIs and real-time applications. It is built on top of Express and provides an easy-to-use blueprint API for generating controllers, models, and services. Sails.js simplifies the development process by incorporating conventions, making it suitable for developers familiar with traditional MVC frameworks.
Key Features:
- Automatic RESTful API generation.
- Real-time features with WebSockets.
- Data-driven architecture.
- Waterline ORM for database access.
Sample Code:
// api/controllers/UserController.js module.exports = { async find(req, res) { const users = await User.find(); res.json(users); }, async create(req, res) { const newUser = await User.create(req.body).fetch(); res.json(newUser); } };
5. Adonis.js
Adonis.js is a full-stack web framework that follows the conventions of Rails and Laravel. It provides a robust set of tools for building scalable and maintainable applications. Adonis.js comes with a built-in ORM (Lucid), powerful middleware support, and a command-line interface (CLI) for scaffolding various components. It is an opinionated framework that aims to boost developer productivity.
Key Features:
- Full-stack framework with built-in ORM.
- Authentication and authorization support.
- Command-line interface for scaffolding.
- Convention over configuration for simplicity.
Sample Code:
// app/Controllers/Http/UserController.js 'use strict'; const User = use('App/Models/User'); class UserController { async index() { const users = await User.all(); return users; } async store({ request }) { const data = request.only(['username', 'email', 'password']); const user = await User.create(data); return user; } } module.exports = UserController;
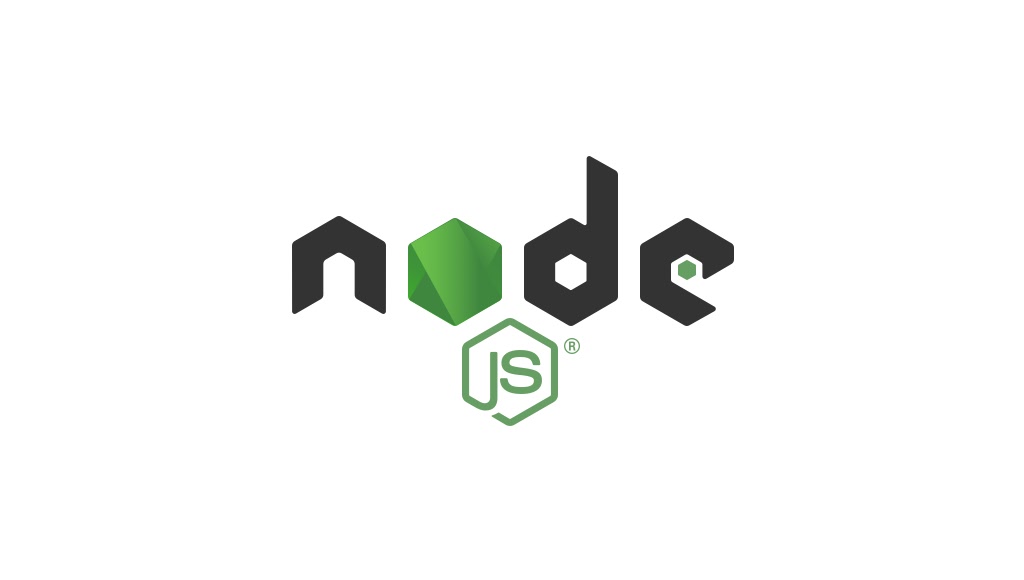
Conclusion
Choosing the right Node.js framework depends on the specific requirements of your project and your development preferences. Whether you prioritize minimalism, modularity, or a full-stack experience, the frameworks mentioned above cover a spectrum of needs. Explore these frameworks, consider your project’s goals, and leverage the strengths of these tools to build efficient and scalable web applications with Node.js.