Understanding Java SE and Java EE: A Comprehensive Overview
Java, a programming language that emerged in the mid-1990s, has become a cornerstone in the software development landscape. Its adaptability, platform independence, and robust features have led to the creation of two distinct editions catering to different development needs: Java SE (Standard Edition) and Java EE (Enterprise Edition).
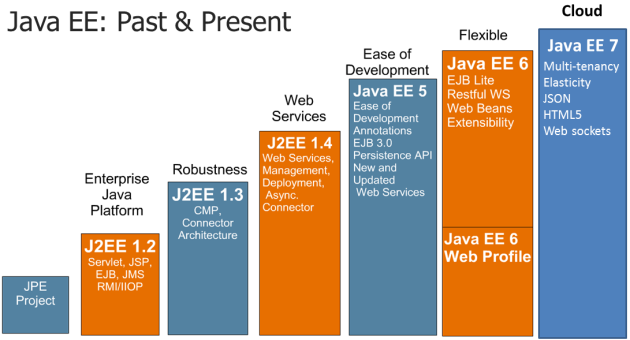
Java SE: A Foundation for General-Purpose Development
1. Platform Independence:
Java SE, the foundation of the Java platform, embodies the “write once, run anywhere” principle. It achieves platform independence through the compilation of source code into an intermediate form called bytecode, which can be executed on any device equipped with a Java Virtual Machine (JVM). This flexibility makes Java SE suitable for a wide range of applications, from desktop software to embedded systems.
2. Core Components:
Java SE provides essential libraries, frameworks, and tools for general-purpose development. Developers can leverage core components such as java.lang, java.util, and java.io to handle fundamental operations like data manipulation, collections, and input/output.
3. Object-Oriented Programming:
Java SE embraces the principles of Object-Oriented Programming (OOP). This paradigm promotes code organization, reusability, and modularity. Concepts like classes, objects, encapsulation, inheritance, and polymorphism form the building blocks for creating structured and maintainable code.
4. Graphical User Interface (GUI) Development:
Java SE includes libraries like Swing and JavaFX, empowering developers to build graphical user interfaces for desktop applications. These libraries facilitate the creation of interactive and visually appealing applications.
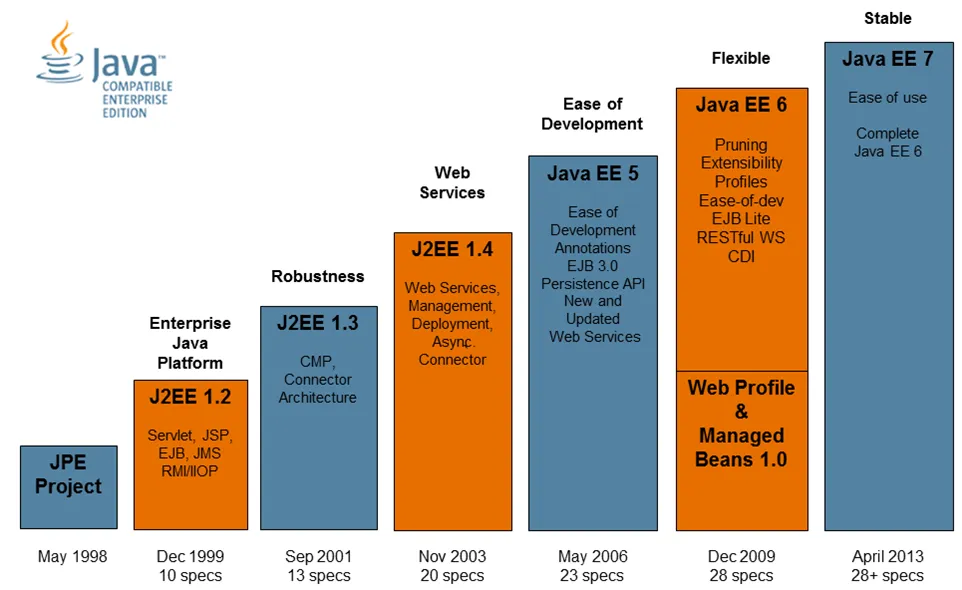
Java EE: Empowering Enterprise-Level Solutions
1. Scalability and Distribution:
Java EE, designed for enterprise-level development, extends the capabilities of Java SE to address the complexities of large-scale applications. It provides features like distributed computing, load balancing, and clustering, enabling applications to scale horizontally to meet increasing demands.
2. Component-Based Development:
Enterprise JavaBeans (EJB) is a key component of Java EE, allowing developers to create modular, reusable, and scalable components. This component-based approach simplifies the development of complex business applications by promoting code organization and maintainability.
3. Web Services and Connectivity:
Java EE incorporates technologies like Java API for RESTful Web Services (JAX-RS) and Java API for XML Web Services (JAX-WS), facilitating the creation and consumption of web services. Additionally, it supports the Java Connector Architecture (JCA), enabling seamless integration with various backend systems and databases.
4. Transaction Management:
In enterprise scenarios, transaction management is crucial for ensuring data consistency and integrity. Java EE provides a standardized approach to transaction management, allowing developers to define and control transactions across multiple operations.
5. Security Features:
Security is paramount in enterprise applications. Java EE includes robust security features, such as role-based access control, encryption, and authentication. These features help protect sensitive data and ensure secure communication within the application.
6. Java Message Service (JMS):
Java EE includes JMS, which enables asynchronous communication between components. This is essential for building responsive and loosely coupled systems, particularly in distributed and enterprise environments.
Choosing the Right Edition for the Job
In practice, developers often choose between Java SE and Java EE based on the nature of the project. Java SE is well-suited for standalone applications, small to medium-sized projects, and scenarios where platform independence is a primary concern. On the other hand, Java EE shines in enterprise-level development, where scalability, distributed computing, and integration capabilities are crucial.
As of my last knowledge update in January 2022, it’s worth noting that the distinction between Java SE and Java EE is evolving. The Eclipse Foundation has taken over the stewardship of Jakarta EE, the successor to Java EE, aiming to drive innovation and open collaboration in the enterprise Java space.
In conclusion, Java SE and Java EE cater to different facets of software development, offering a versatile and comprehensive toolkit for developers. Whether building a desktop application, a mobile app, or a large-scale enterprise system, the Java platform provides the tools and capabilities needed to meet diverse development requirements.
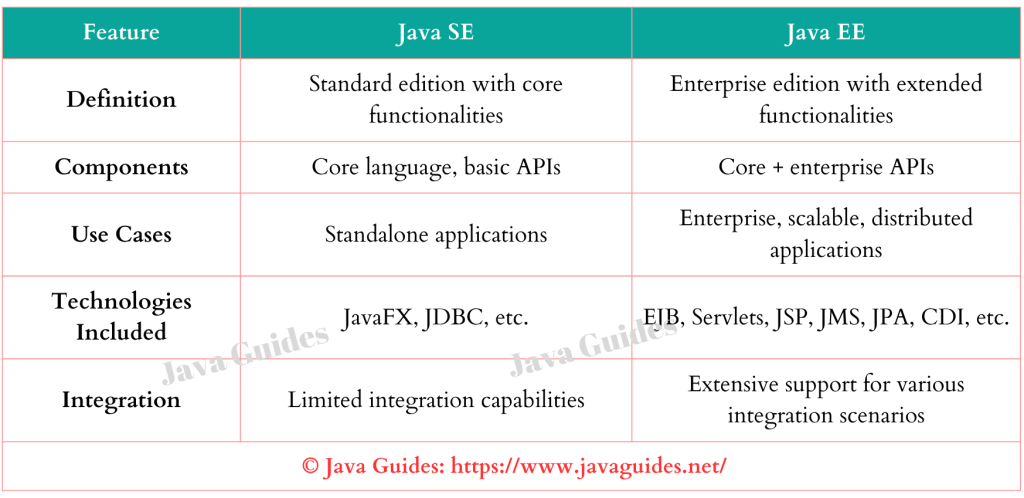
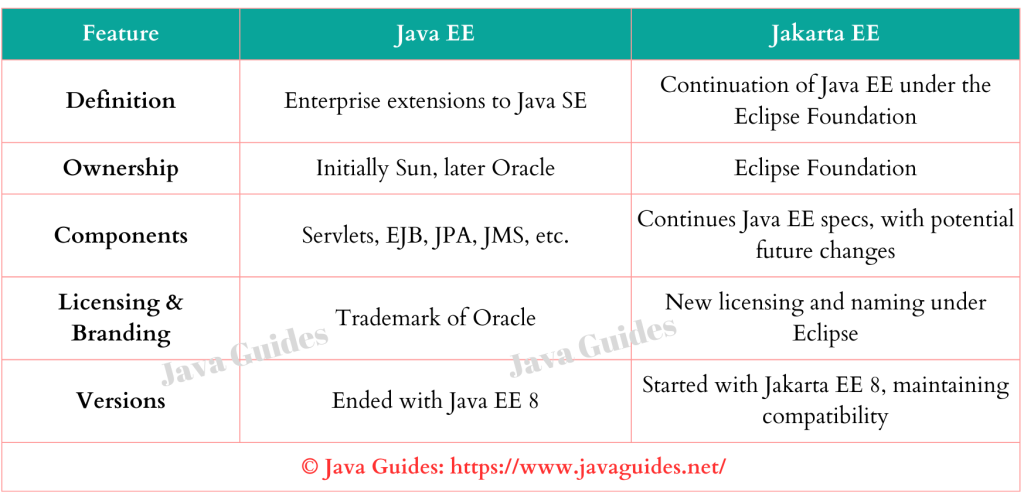
Below are simplified code samples for both Java SE and Java EE.
Java SE (Standard Edition) Sample Code:
public class HelloWorld { public static void main(String[] args) { // Simple Java SE program that prints "Hello, World!" to the console System.out.println("Hello, World!"); } }
This Java SE code represents a basic “Hello, World!” program. When executed, it prints the specified message to the console.
Java EE (Enterprise Edition) Sample Code (Servlet):
import java.io.IOException; import java.io.PrintWriter; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; @WebServlet("/HelloServlet") public class HelloServlet extends HttpServlet { protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // Set the response content type response.setContentType("text/html"); // Get a PrintWriter object to send HTML response PrintWriter out = response.getWriter(); // HTML content out.println("<html>"); out.println("<head><title>HelloServlet</title></head>"); out.println("<body>"); out.println("<h2>Hello from HelloServlet!</h2>"); out.println("<p>This is a simple Java EE Servlet example.</p>"); out.println("</body>"); out.println("</html>"); } }
In this Java EE example, we have a simple servlet named HelloServlet
. When accessed, it responds with an HTML page containing a greeting message.
To run these examples:
- Java SE:
- Save the Java SE code in a file named
HelloWorld.java
. - Compile using
javac HelloWorld.java
. - Run using
java HelloWorld
.
- Java EE (Servlet):
- Create a dynamic web project in your Java EE IDE.
- Copy the
HelloServlet
code into the appropriate package in your project. - Deploy the project to a Java EE application server (e.g., Apache Tomcat).
- Access the servlet through a web browser using the specified URL pattern (e.g.,
http://localhost:8080/your-web-app-context/HelloServlet
).
These examples serve as a starting point, and in real-world scenarios, Java EE applications involve more complex architectures, additional technologies, and adherence to enterprise-level standards.