Mastering C# Best Practices and Coding Standards: A Blueprint for Clean and Efficient Code
Creating clean, maintainable, and efficient code is essential for the success of any software project. In the realm of C#, adhering to best practices and coding standards becomes a crucial aspect of producing high-quality code. In this article, we’ll explore a set of C# best practices and coding standards that will guide you towards writing clean and robust code.
1. Naming Conventions:
Camel Case for Variables and Methods:
// Good int totalCount; void calculateTotal(); // Avoid int total_count; void Calculate_Total();
Pascal Case for Classes and Methods:
// Good class CustomerService { void ProcessOrder(); } // Avoid class customer_service { void processOrder(); }
2. Code Readability:
- Use meaningful names for variables, methods, and classes.
- Keep methods and classes concise, adhering to the Single Responsibility Principle (SRP).
- Avoid unnecessary abbreviations.
3. Consistent Formatting:
- Use consistent indentation (spaces or tabs), and choose a standard width.
- Be consistent with braces placement (Allman style or K&R style).
4. Comments and Documentation:
- Write comments for complex code sections or to clarify the purpose of a method.
- Use XML comments for documenting public APIs.
5. Exception Handling:
- Only catch exceptions that you can handle.
- Avoid catching general exceptions (
catch(Exception ex)
). - Log exceptions with relevant information.
6. Code Reusability:
- Promote code reusability by designing classes with a single responsibility.
- Utilize inheritance and interfaces wisely for common functionalities.
- Consider creating utility classes or extension methods for reusable code.
7. Null Checking:
- Prefer using null-conditional operators (
?.
and??
) for null checking. - Avoid using
null
to represent an empty collection; use empty collections instead.
8. LINQ Usage:
- Use LINQ for querying collections instead of traditional loops where appropriate.
- Be mindful of performance implications; prefer
ToList()
orToArray()
when iterating multiple times.
9. Unit Testing:
- Write unit tests for critical functionalities.
- Follow the Arrange-Act-Assert (AAA) pattern in your tests.
- Keep unit tests isolated and independent of each other.
10. Code Reviews:
- Conduct regular code reviews to ensure adherence to coding standards.
- Foster a culture of constructive feedback within the development team.
11. Version Control:
- Use meaningful commit messages.
- Regularly update your local branch from the main branch.
- Avoid committing large binary files.
12. Security Considerations:
- Sanitize input to prevent SQL injection and other security vulnerabilities.
- Use parameterized queries when interacting with databases.
13. Asynchronous Programming:
- Prefer asynchronous methods (
async
andawait
) for I/O-bound operations. - Be cautious with
async void
; preferasync Task
for methods that don’t return a value.
14. Dispose of Resources:
- Implement the
IDisposable
interface or useusing
statements for classes that manage resources. - Be mindful of memory leaks, especially in long-running applications.
15. Continuous Learning:
- Stay updated with the latest C# features and best practices.
- Embrace new language features introduced in newer versions of C#.
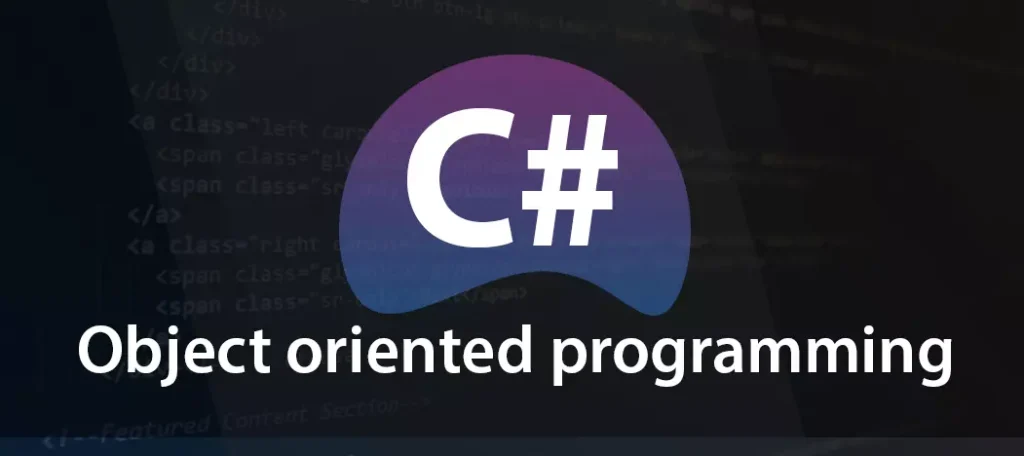
Conclusion: Elevating Your C# Code to Excellence
Adhering to best practices and coding standards in C# is not just about writing code that works; it’s about creating code that is maintainable, scalable, and a joy to work with. By adopting these guidelines, you pave the way for collaboration, reduce technical debt, and ensure that your codebase remains healthy throughout its lifecycle. Whether you are a seasoned developer or just starting with C#, following these best practices will set you on the path to writing clean and efficient code.