Data Science, a multidisciplinary field that combines statistics, mathematics, and computer science, leverages Python as a primary tool for data analysis and visualization. In this article, we will explore the fundamentals of data science with Python and delve into the art of visualization using popular libraries, accompanied by code samples for practical understanding.
1. Introduction to Data Science with Python:
Data Science involves extracting meaningful insights and knowledge from data. Python has emerged as a dominant language in the field due to its rich ecosystem of libraries, ease of use, and versatility.
2. Python Libraries for Data Science:
- NumPy: Provides support for large, multi-dimensional arrays and matrices, along with mathematical functions.
- Pandas: A powerful library for data manipulation and analysis, offering data structures like DataFrame and Series.
- Matplotlib and Seaborn: Libraries for data visualization, enabling the creation of various plots and charts.
- Scikit-learn: A comprehensive machine learning library that includes tools for data mining and data analysis.
3. Sample Code: Loading and Exploring Data:
import pandas as pd # Load dataset (example: Iris dataset) url = 'https://archive.ics.uci.edu/ml/machine-learning-databases/iris/iris.data' column_names = ['sepal_length', 'sepal_width', 'petal_length', 'petal_width', 'class'] iris_data = pd.read_csv(url, header=None, names=column_names) # Display the first few rows of the dataset print(iris_data.head())
4. Data Visualization with Matplotlib:
import matplotlib.pyplot as plt # Scatter plot plt.scatter(iris_data['sepal_length'], iris_data['sepal_width']) plt.title('Scatter Plot of Sepal Length vs Sepal Width') plt.xlabel('Sepal Length') plt.ylabel('Sepal Width') plt.show()
5. Data Visualization with Seaborn:
import seaborn as sns # Box plot sns.boxplot(x='class', y='petal_length', data=iris_data) plt.title('Box Plot of Petal Length by Class') plt.show()
6. Exploratory Data Analysis (EDA):
EDA involves analyzing and visualizing data to uncover patterns, trends, and anomalies. Seaborn’s pair plot is a powerful tool for visualizing relationships between multiple variables.
# Pair plot sns.pairplot(iris_data, hue='class', markers='o') plt.suptitle('Pair Plot of Iris Dataset', y=1.02) plt.show()
7. Machine Learning with Scikit-learn:
Let’s use a simple machine learning model, such as the k-nearest neighbors (KNN) classifier, to demonstrate the application of Scikit-learn.
from sklearn.model_selection import train_test_split from sklearn.neighbors import KNeighborsClassifier from sklearn.metrics import accuracy_score # Prepare data X = iris_data.drop('class', axis=1) y = iris_data['class'] # Split data into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Create and train the KNN classifier knn_classifier = KNeighborsClassifier(n_neighbors=3) knn_classifier.fit(X_train, y_train) # Make predictions y_pred = knn_classifier.predict(X_test) # Evaluate accuracy accuracy = accuracy_score(y_test, y_pred) print(f'Accuracy: {accuracy}')
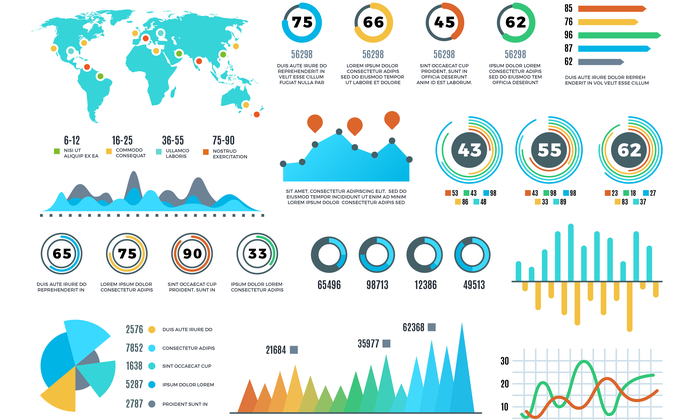
8. Conclusion:
Python’s data science ecosystem, encompassing libraries such as NumPy, Pandas, Matplotlib, Seaborn, and Scikit-learn, provides a robust platform for conducting data analysis and visualization. This article introduced the basics of data science with Python and showcased the power of visualization through code samples. From loading and exploring datasets to creating insightful visualizations and applying machine learning models, Python empowers data scientists to unravel meaningful insights and drive informed decision-making. As you embark on your data science journey, experimenting with various datasets and exploring advanced techniques will deepen your understanding and proficiency in this dynamic field.