In an era where digital threats are ever-evolving, Python has become a weapon of choice for cybersecurity professionals and developers. Its versatility, readability, and extensive libraries make it a potent tool for various cybersecurity tasks. In this article, we’ll explore the role of Python in cybersecurity and delve into practical applications with code examples.
1. Introduction to Cybersecurity with Python:
Cybersecurity involves protecting systems, networks, and programs from digital attacks. Python, with its simplicity and powerful libraries, plays a crucial role in various aspects of cybersecurity, from penetration testing to automation and incident response.
2. Python Libraries for Cybersecurity:
- Scapy: A powerful packet manipulation library for network analysis and penetration testing.
- Requests: A versatile HTTP library for sending HTTP requests, often used for web application security testing.
- Cryptography: A library for secure communication and encryption.
- PyCryptoDome: A collection of cryptographic algorithms and protocols.
- PyCap: Python bindings for libpcap, useful for network traffic analysis.
3. Sample Code: Network Packet Sniffing with Scapy:
from scapy.all import sniff # Define a packet handler function def packet_handler(packet): if packet.haslayer('IP'): ip_src = packet['IP'].src ip_dst = packet['IP'].dst print(f"Source IP: {ip_src}, Destination IP: {ip_dst}") # Start sniffing network traffic sniff(prn=packet_handler, store=0, count=5)
This code uses Scapy to sniff network packets and extract source and destination IP addresses. In a cybersecurity context, such packet analysis can reveal potential threats or vulnerabilities.
4. Web Scraping for Security Intelligence:
import requests from bs4 import BeautifulSoup # Fetch the latest cybersecurity news url = 'https://example.com/cybersecurity-news' response = requests.get(url) soup = BeautifulSoup(response.text, 'html.parser') # Extract headlines headlines = soup.find_all('h2', class_='news-title') for headline in headlines: print(headline.text)
Web scraping can be used to gather intelligence on cybersecurity news and threat reports, helping security professionals stay informed about the latest developments.
5. Cryptographic Operations with PyCryptoDome:
from Crypto.Cipher import AES from Crypto.Random import get_random_bytes # Encrypt a message using AES-GCM key = get_random_bytes(16) cipher = AES.new(key, AES.MODE_GCM) plaintext = b'This is a confidential message' ciphertext, tag = cipher.encrypt_and_digest(plaintext) # Decrypt the message decipher = AES.new(key, AES.MODE_GCM, nonce=cipher.nonce) decrypted_text = decipher.decrypt_and_verify(ciphertext, tag) print(f"Decrypted Message: {decrypted_text.decode('utf-8')}")
Python’s Cryptography library, in this example using PyCryptoDome, enables secure encryption and decryption of sensitive information.
6. Network Scanning with Nmap and Python:
import nmap # Create an Nmap scanner object nm = nmap.PortScanner() # Scan a target host for open ports target_host = 'example.com' nm.scan(target_host, arguments='-p 1-1000') # Display open ports for port in nm[target_host]['tcp']: state = nm[target_host]['tcp'][port]['state'] print(f"Port {port} is {state}")
Python can interact with external tools like Nmap to perform network scanning and identify potential vulnerabilities in a system.
7. Automation with Python Scripts:
Automating repetitive tasks is a key aspect of cybersecurity. Python scripts can automate tasks such as log analysis, vulnerability scanning, and incident response.
8. Cybersecurity Challenges with Python:
While Python is a powerful ally in cybersecurity, it’s essential to be aware of potential challenges, such as code execution vulnerabilities, insecure dependencies, and the need for continuous updates to address emerging threats.
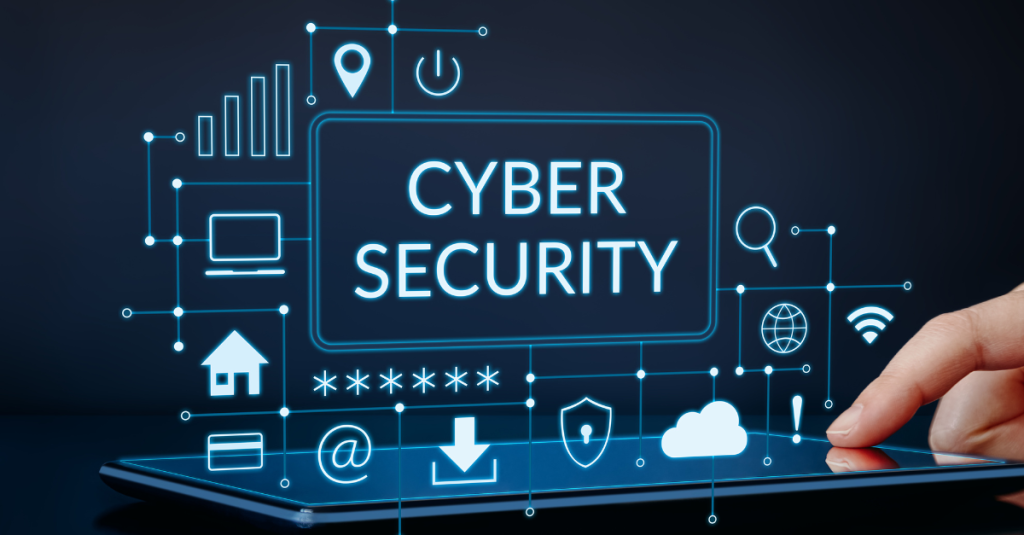
9. Conclusion:
Python’s role in cybersecurity extends from network analysis to encryption and automation. Its simplicity, extensive libraries, and active community make it an invaluable tool for both cybersecurity professionals and developers. This article provided a glimpse into the world of cybersecurity with Python, showcasing practical applications and code examples. As the cybersecurity landscape evolves, Python continues to be at the forefront, empowering defenders to stay ahead of digital threats and secure the ever-expanding digital realm.