JSON (JavaScript Object Notation) is a lightweight data interchange format widely used for data exchange between a server and a web application, and even between different parts of an application. In PHP, working with JSON is seamless due to built-in functions that make encoding and decoding JSON data straightforward. In this article, we’ll explore the basics of handling JSON in PHP with sample code for common use cases.
Encoding PHP Arrays into JSON
PHP provides the json_encode
function to convert PHP data structures, such as arrays or objects, into a JSON-formatted string.
<?php // Sample PHP array $data = [ 'name' => 'John Doe', 'age' => 30, 'city' => 'Example City', ]; // Encode PHP array into JSON $jsonData = json_encode($data); // Display the JSON data echo $jsonData; ?>
In this example, the PHP array $data
is encoded into a JSON string using json_encode
. The output will be:
{"name":"John Doe","age":30,"city":"Example City"}
Decoding JSON into PHP Arrays
Conversely, PHP provides json_decode
to parse a JSON string and convert it into a PHP array or object.
<?php // Sample JSON string $jsonData = '{"name":"John Doe","age":30,"city":"Example City"}'; // Decode JSON into PHP array $data = json_decode($jsonData, true); // Display the PHP array print_r($data); ?>
The output will be:
Array ( [name] => John Doe [age] => 30 [city] => Example City )
Handling JSON Errors
When working with JSON encoding or decoding, it’s crucial to handle potential errors. The json_last_error
function provides information about the last JSON operation.
<?php // Sample JSON string with an error $jsonData = '{"name":"John Doe","age":30,"city":}'; // Decode JSON with error handling $data = json_decode($jsonData, true); // Check for JSON decoding errors if (json_last_error() !== JSON_ERROR_NONE) { echo 'JSON decoding error: ' . json_last_error_msg(); } else { print_r($data); } ?>
In this case, the JSON string contains an error, and the output will be:
JSON decoding error: Syntax error
Working with JSON in Real Applications
When interacting with APIs or handling data in web applications, encoding and decoding JSON become essential. Here’s an example of a simple use case where PHP fetches data from an API, decodes the JSON response, and processes the data:
<?php // API endpoint $apiEndpoint = 'https://jsonplaceholder.typicode.com/todos/1'; // Fetch JSON data from the API $jsonData = file_get_contents($apiEndpoint); // Decode JSON into PHP array $data = json_decode($jsonData, true); // Display relevant information echo 'Title: ' . $data['title'] . "\n"; echo 'Completed: ' . ($data['completed'] ? 'Yes' : 'No') . "\n"; ?>
This example fetches a todo item from the JSONPlaceholder API, decodes the JSON response, and displays the title and completion status.
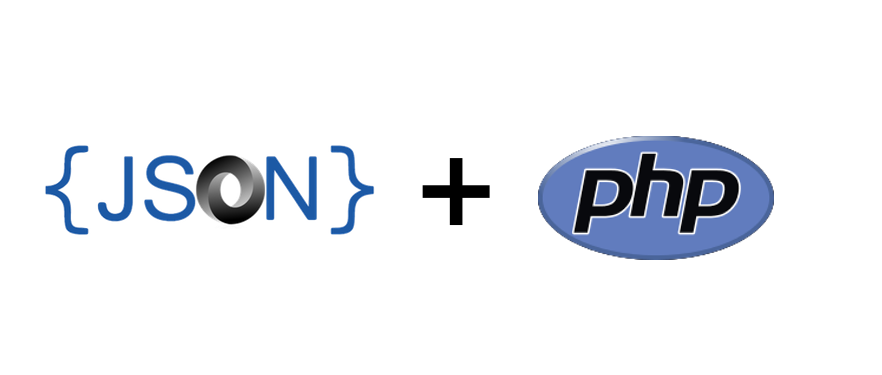
Conclusion
Understanding how to handle JSON in PHP is crucial for web development, especially when dealing with data exchange or API integration. PHP’s built-in functions make it easy to encode and decode JSON, and handling errors ensures robustness in your applications. Whether you’re working with data from APIs, storing configuration settings, or exchanging information between different parts of your application, mastering JSON in PHP is a valuable skill for any PHP developer.