Introduction
Node.js, with its modular and scalable architecture, thrives on the concepts of modules and packages. In this article, we’ll explore how Node.js handles modules, the npm package manager, and the seamless integration of external packages to enhance your application’s functionality.
1. Understanding Node.js Modules
1.1 Creating a Module:
A module in Node.js is a reusable piece of code that encapsulates related functionality. Modules can be created by placing code in separate files. Let’s create a simple module that calculates the area of a rectangle.
// rectangle.js const calculateArea = (length, width) => { return length * width; }; module.exports = calculateArea;
1.2 Importing a Module:
To use the module in another file, we import it using the require
function.
// main.js const calculateArea = require('./rectangle'); const length = 5; const width = 10; const area = calculateArea(length, width); console.log(`The area of the rectangle is: ${area}`);
In this example, calculateArea
is a function exported from the rectangle.js
module and imported into the main.js
file.
2. Node.js Package Manager (npm)
2.1 npm Basics:
npm (Node Package Manager) is a powerful tool for managing packages and dependencies in Node.js. It comes bundled with Node.js installations.
- Initializing a Package:
To start using npm in your project, initialize apackage.json
file by runningnpm init
and following the prompts.
npm init
- Installing Packages:
Usenpm install
to install packages. For example, let’s install thelodash
library.
npm install lodash
2.2 Using Installed Packages:
Once a package is installed, you can use it in your code by requiring it.
// main.js const _ = require('lodash'); const numbers = [1, 2, 3, 4, 5]; const sum = _.sum(numbers); console.log(`The sum of the numbers is: ${sum}`);
In this example, we’re using the lodash
library to calculate the sum of an array.
3. Creating Your Own npm Package
3.1 Creating a Package:
You can create and publish your own npm package. Here’s a simple example:
- Create a new directory for your package and navigate to it.
mkdir my-package cd my-package
- Inside the directory, create a file (e.g.,
index.js
) with your module code.
// index.js const greet = () => { console.log('Hello from my package!'); }; module.exports = greet;
- Initialize the package by running
npm init
and follow the prompts.
npm init
- Create a GitHub repository for your package.
- Publish your package to npm.
npm login npm publish --access public
3.2 Using Your Published Package:
Once your package is published, others can use it by installing it from npm.
npm install your-package-name
Then, in their code:
const greet = require('your-package-name'); greet(); // Outputs: Hello from my package!
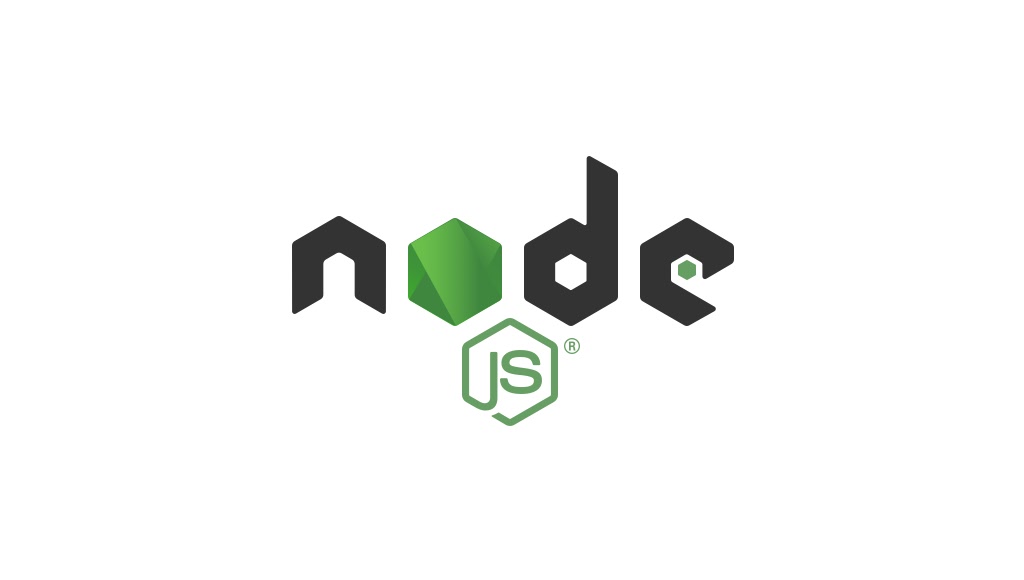
4. Conclusion
Node.js modules and npm packages form the backbone of building modular and scalable applications. By breaking down code into smaller, manageable modules, you can enhance reusability and maintainability. npm, with its vast repository of packages, simplifies the process of integrating external functionality into your projects.
Whether you’re importing modules within your project or leveraging external packages, Node.js’s modular approach and npm’s package management capabilities empower developers to build sophisticated and efficient applications. Embrace these concepts, explore the rich npm ecosystem, and elevate your Node.js development experience to new heights.