Amazon Simple Storage Service (S3) is a highly scalable and secure object storage service provided by Amazon Web Services (AWS). Integrating PHP with AWS S3 enables you to manage and store files in the cloud seamlessly. In this guide, we’ll cover the essential steps to get you started with PHP and AWS S3 integration.
1. Set Up AWS Account and S3 Bucket
If you don’t have an AWS account, sign up for one at AWS Console. After signing in, navigate to the S3 service and create a new bucket. Take note of your AWS access key, secret key, and the region where your S3 bucket is located.
2. Install AWS SDK for PHP
AWS provides an official SDK for PHP, making it easy to interact with S3. You can install it using Composer, the PHP dependency manager.
composer require aws/aws-sdk-php
This command installs the AWS SDK for PHP and its dependencies.
3. Set Up AWS SDK Credentials
Create a file named config.php
to store your AWS credentials securely.
<?php // config.php return [ 'credentials' => [ 'key' => 'YOUR_AWS_ACCESS_KEY', 'secret' => 'YOUR_AWS_SECRET_KEY', ], 'region' => 'YOUR_AWS_REGION', 'version' => 'latest', ];
Replace 'YOUR_AWS_ACCESS_KEY'
, 'YOUR_AWS_SECRET_KEY'
, and 'YOUR_AWS_REGION'
with your AWS access key, secret key, and region.
4. Upload File to S3
Now, let’s create a PHP script to upload a file to your S3 bucket. Create a file named upload.php
.
<?php // upload.php require 'vendor/autoload.php'; $config = require 'config.php'; use Aws\S3\S3Client; use Aws\S3\Exception\S3Exception; // Create an S3 client $s3 = new S3Client($config); // Specify your S3 bucket name $bucket = 'your-s3-bucket-name'; // Specify the file to upload and the destination in S3 $key = 'path/to/your/file.txt'; $file = '/path/to/local/file.txt'; try { $result = $s3->putObject([ 'Bucket' => $bucket, 'Key' => $key, 'Body' => fopen($file, 'rb'), 'ACL' => 'public-read', // Adjust permissions as needed ]); echo "File uploaded successfully: {$result['ObjectURL']}"; } catch (S3Exception $e) { echo "Error uploading file: {$e->getMessage()}"; }
Adjust the $bucket
, $key
, and $file
variables according to your setup. This script uses the putObject
method to upload a file to the specified S3 bucket.
5. Download File from S3
Create a file named download.php
to download a file from S3.
<?php // download.php require 'vendor/autoload.php'; $config = require 'config.php'; use Aws\S3\S3Client; use Aws\S3\Exception\S3Exception; // Create an S3 client $s3 = new S3Client($config); // Specify your S3 bucket name $bucket = 'your-s3-bucket-name'; // Specify the file to download from S3 $key = 'path/to/your/file.txt'; try { $result = $s3->getObject([ 'Bucket' => $bucket, 'Key' => $key, ]); header("Content-Type: {$result['ContentType']}"); header("Content-Disposition: attachment; filename=\"{$key}\""); echo $result['Body']; } catch (S3Exception $e) { echo "Error downloading file: {$e->getMessage()}"; }
Adjust the $bucket
and $key
variables. This script uses the getObject
method to retrieve a file from S3 and sends it as a downloadable response.
6. Additional Operations
Explore other operations provided by the AWS SDK for PHP to manage objects, set permissions, and perform more advanced tasks with AWS S3.
- List Objects in a Bucket:
$objects = $s3->listObjectsV2(['Bucket' => $bucket]); foreach ($objects['Contents'] as $object) { echo $object['Key'] . PHP_EOL; }
- Delete Object:
$s3->deleteObject(['Bucket' => $bucket, 'Key' => $key]); echo "Object deleted successfully.";
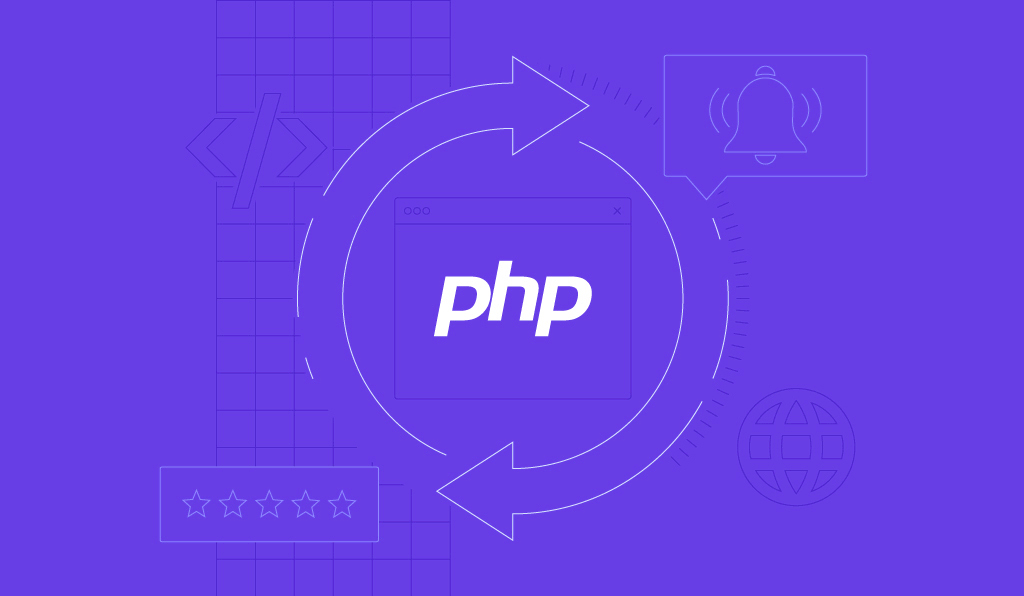
Integrating PHP with AWS S3 allows you to leverage the power and scalability of cloud storage for your applications. AWS provides extensive documentation and resources, allowing you to explore additional features and optimize your file storage and retrieval processes based on your application’s needs.