The Persistent Relevance of PHP in Web Development
PHP, which stands for Hypertext Preprocessor, has been a stalwart in the realm of web development for decades. Despite the ever-evolving landscape of programming languages and frameworks, PHP has maintained its relevance, and as we step into 2024, it continues to be a crucial player in the development of dynamic and interactive websites.
1. Simplicity and Versatility
One of PHP’s enduring strengths lies in its simplicity. It was designed to be easy to learn and use, making it accessible for both beginners and experienced developers. Its syntax, resembling C and Perl, facilitates a quick learning curve for those already familiar with these languages.
PHP’s versatility is another key factor contributing to its sustained popularity. It can seamlessly integrate with HTML, allowing developers to embed PHP code directly into their HTML files. This enables the creation of dynamic content and the execution of server-side scripts without the need for external files.
2. Vibrant Ecosystem and Community Support
PHP boasts a robust ecosystem of libraries, frameworks, and tools that expedite development processes. Frameworks like Laravel, Symfony, and CodeIgniter provide structured solutions for building web applications, ensuring scalability and maintainability. These frameworks come equipped with features such as routing, templating, and database abstraction, streamlining the development workflow.
The PHP community, known for its fervor and inclusivity, plays a crucial role in the language’s endurance. Countless forums, blogs, and online resources provide support, tutorials, and solutions to common issues. The collaborative spirit within the community fosters an environment where developers can share knowledge and enhance their skills continually.
3. Content Management Systems (CMS) and Popular Platforms
PHP is the backbone of numerous Content Management Systems (CMS) that power a significant portion of websites. WordPress, one of the most widely used CMS platforms, relies heavily on PHP for its core functionality. This enduring presence in the CMS landscape ensures that PHP remains a staple in web development, especially for content-driven websites.
4. Server-Side Scripting Prowess
PHP’s strength in server-side scripting contributes to its effectiveness in creating dynamic web pages. It allows developers to execute code on the server, generating content that is then sent to the client’s browser. This capability is crucial for building interactive web applications and managing data securely on the server.
5. Continuous Improvement and Modernization
PHP has not rested on its laurels; it has undergone significant updates and improvements over the years. The release of PHP 7 brought substantial performance enhancements and introduced features like scalar type declarations and return type declarations, enhancing code quality and readability.
The language’s adaptability to emerging trends, such as cloud computing and containerization, further cements its relevance in contemporary web development.
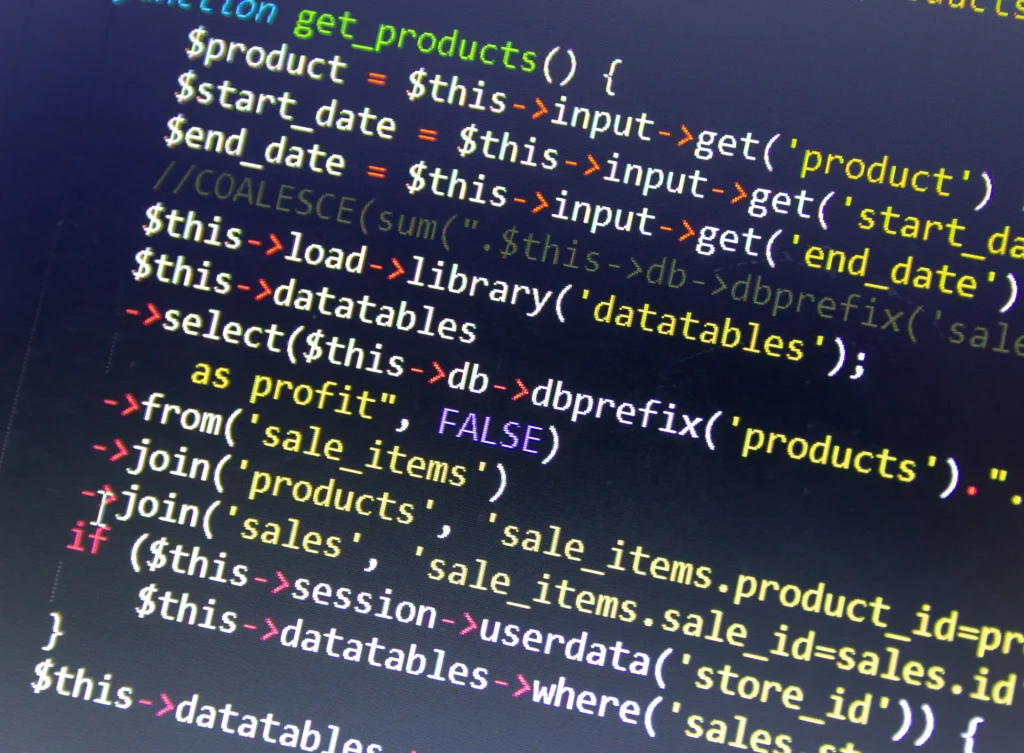
PHP’s enduring relevance in 2024 can be attributed to its simplicity, versatility, vibrant community, integration with popular platforms, server-side scripting capabilities, and commitment to continuous improvement. As the web development landscape evolves, PHP continues to stand as a reliable and effective tool for developers seeking to create dynamic, feature-rich websites and applications. Its legacy endures, and its future looks promising in the ever-expanding world of digital innovation.
Below is a sample PHP code for a simple web page that uses HTML and PHP to display a dynamic message:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>PHP Sample Code</title> <style> body { font-family: Arial, sans-serif; background-color: #f4f4f4; text-align: center; margin: 50px; } h1 { color: #333; } p { color: #666; } </style> </head> <body> <?php // PHP code to display a dynamic message $currentHour = date('G'); if ($currentHour < 12) { $greeting = 'Good morning'; } elseif ($currentHour < 18) { $greeting = 'Good afternoon'; } else { $greeting = 'Good evening'; } $username = isset($_GET['username']) ? htmlspecialchars($_GET['username']) : 'Guest'; // Displaying the dynamic message echo "<h1>$greeting, $username!</h1>"; ?> <p>This is a simple PHP sample code demonstrating dynamic content generation in a web page.</p> </body> </html>
This code creates a basic HTML structure and uses PHP to generate a dynamic greeting message based on the current time of day. It also allows users to customize the greeting by passing a ‘username’ parameter in the URL.
Save this code in a PHP file (e.g., index.php
) and run it on a web server with PHP support. When you access the page, you’ll see a greeting message that changes based on the time of day and any provided username in the URL.
Feel free to use and modify this code as a starting point for your PHP projects or to demonstrate the integration of PHP with HTML for dynamic web content.