Introduction
Microservices architecture has gained immense popularity for its ability to break down complex applications into smaller, independent services. Node.js, with its lightweight and scalable nature, is an excellent choice for building microservices. In this article, we’ll explore the key concepts of microservices and demonstrate how to implement them using Node.js.
Understanding Microservices Architecture
Microservices is an architectural style where an application is composed of small, independent services that communicate through well-defined APIs. Each service focuses on a specific business capability and can be developed, deployed, and scaled independently. This modular approach enhances flexibility, maintainability, and scalability.
Key Components of a Microservices Architecture
1. Service Independence
Each microservice is a self-contained unit with its own database and business logic. This independence allows development teams to work on different services simultaneously without affecting each other.
2. APIs and Communication
Microservices communicate through APIs. Well-defined APIs enable seamless integration and interaction between services. Common communication patterns include HTTP/REST or message queues.
3. Decentralized Data Management
Unlike traditional monolithic applications with a single database, microservices often have their own databases. This decentralization of data ensures that services are loosely coupled and can evolve independently.
4. Autonomous Deployment
Microservices can be deployed independently. This allows for more frequent releases, reduces downtime, and makes it easier to roll back changes if needed.
Implementing Microservices with Node.js
Now, let’s dive into a basic example of implementing microservices using Node.js. We’ll create two simple services – one for user management and another for product management.
User Service (user-service.js)
const express = require('express'); const app = express(); const port = 3001; app.get('/users', (req, res) => { // Logic to fetch users from the database res.json({ users: [{ id: 1, name: 'John Doe' }, { id: 2, name: 'Jane Smith' }] }); }); app.listen(port, () => { console.log(`User Service listening at http://localhost:${port}`); });
Product Service (product-service.js)
const express = require('express'); const app = express(); const port = 3002; app.get('/products', (req, res) => { // Logic to fetch products from the database res.json({ products: [{ id: 101, name: 'Laptop' }, { id: 102, name: 'Smartphone' }] }); }); app.listen(port, () => { console.log(`Product Service listening at http://localhost:${port}`); });
In this example:
- Each service is a standalone Node.js application using the Express framework.
- The user service exposes an endpoint
/users
to retrieve user data. - The product service exposes an endpoint
/products
to retrieve product data.
These services can now run independently, communicate through their APIs, and be scaled or updated without affecting each other.
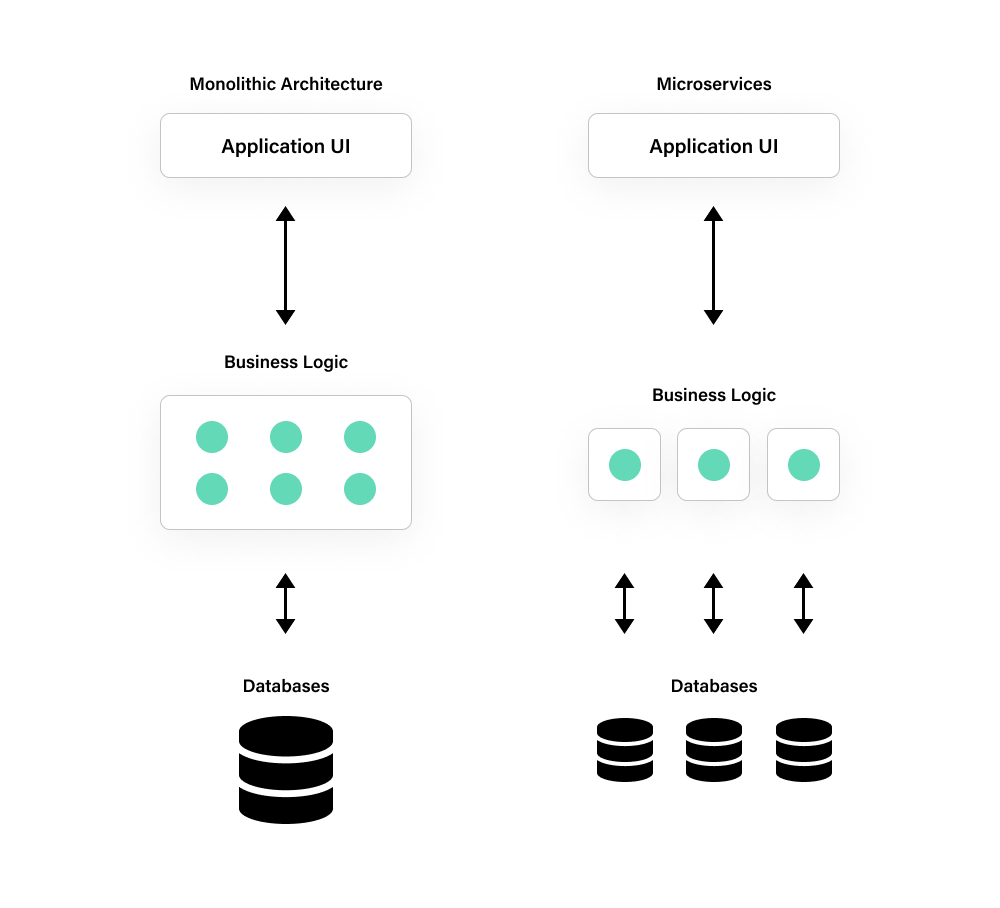
Conclusion
Node.js provides an ideal environment for building microservices, thanks to its non-blocking I/O, scalability, and ease of development. Implementing microservices with Node.js allows for a modular and flexible architecture, empowering development teams to create and maintain complex applications with efficiency and agility. As you explore microservices further, consider additional aspects such as service discovery, load balancing, and containerization to enhance the overall architecture.