The PHP Command Line Interface (CLI) is a powerful tool that allows developers to run PHP scripts directly from the command line, outside the context of a web server. In this guide, we’ll explore the fundamentals of PHP CLI, its use cases, and some common tasks you can perform.
1. Basics of PHP CLI
Running a PHP Script
To run a PHP script from the command line, use the following command:
php script.php
Replace script.php
with the filename of your PHP script.
Hello World Example
Create a simple PHP script (e.g., hello.php
) with the following content:
<?php echo "Hello, World!\n"; ?>
Run it using:
php hello.php
Passing Arguments
You can pass arguments to a PHP script:
php script.php arg1 arg2
Access these arguments in your script using the $argv
array:
<?php echo "Arguments: " . implode(', ', $argv) . "\n"; ?>
Interactive Mode
PHP CLI also provides an interactive mode for testing code snippets:
php -a
2. Use Cases for PHP CLI
1. Script Automation
Automate repetitive tasks, such as file processing, data migrations, or system maintenance, by creating PHP scripts that can be scheduled or triggered manually.
2. Cron Jobs
Schedule tasks at specific intervals using cron jobs. For example, run a script every day at midnight:
0 0 * * * php /path/to/script.php
3. Command Line Tools
Develop command line tools for your applications, allowing users to perform various operations without accessing the web interface.
3. Working with CLI Input and Output
Reading User Input
Use fgets()
to read user input from the command line:
<?php echo "Enter your name: "; $name = trim(fgets(STDIN)); echo "Hello, $name!\n"; ?>
Output Formatting
Use ANSI escape codes for formatting output. For example, to display text in red:
<?php echo "\033[31mThis text is red.\033[0m\n"; ?>
4. Handling Signals
PHP CLI scripts can respond to signals, such as SIGINT
(Ctrl+C). Use the pcntl_signal()
function to set up signal handlers:
<?php declare(ticks=1); function handleSignal($signal) { switch ($signal) { case SIGINT: echo "Received SIGINT. Exiting...\n"; exit; } } pcntl_signal(SIGINT, 'handleSignal'); // Your script logic goes here ?>
5. Working with External Commands
PHP CLI allows you to execute external commands using exec()
or shell_exec()
:
<?php $result = shell_exec('ls -l'); echo $result; ?>
Exercise caution when executing external commands to avoid security risks.
6. Error Handling
Implement robust error handling in your CLI scripts. Use try
, catch
, and finally
blocks to handle exceptions:
<?php try { // Your code that may throw exceptions } catch (Exception $e) { echo "Error: " . $e->getMessage() . "\n"; } finally { // Cleanup or additional actions } ?>
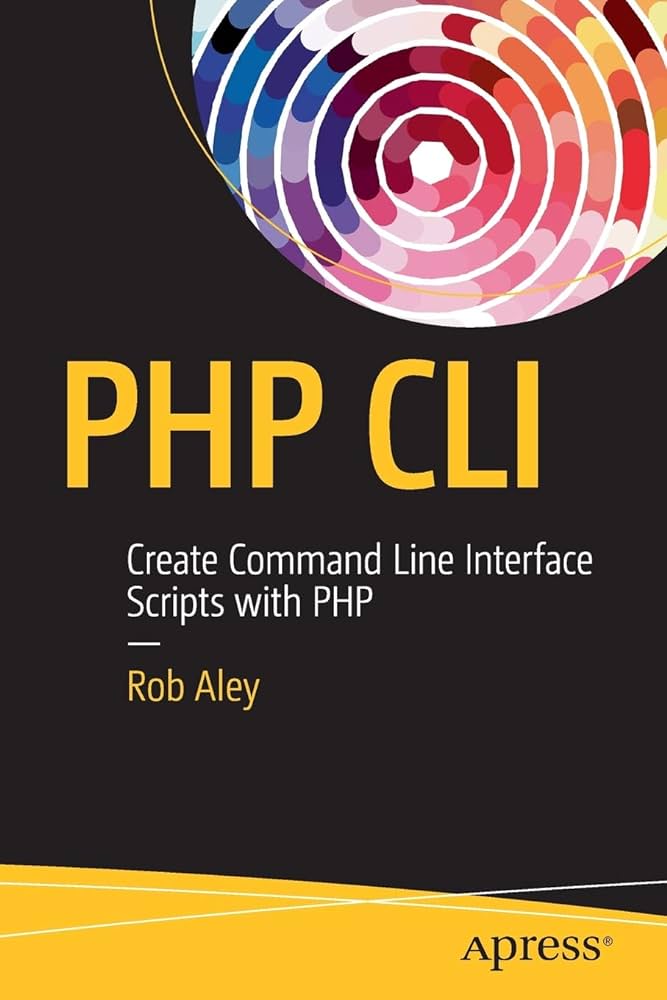
Conclusion
The PHP Command Line Interface is a versatile tool for running PHP scripts outside a web server context. Whether automating tasks, building command line tools, or interacting with users via the console, PHP CLI offers a range of functionalities. Familiarize yourself with its features and consider its application in your development workflow for efficient and flexible scripting.