Introduction
C# is a powerful and versatile programming language, but even experienced developers can fall into common traps that lead to bugs, performance issues, or maintenance challenges. In this article, we’ll explore the top 5 most common mistakes in C# and discuss how to avoid them.
1. Neglecting Null Checks
Null reference exceptions are notorious for causing runtime errors and unexpected crashes. Failing to check for null values can lead to unintended consequences. Consider the following example:
string name = GetNameFromDatabase(); int nameLength = name.Length; // NullReferenceException if name is null
To prevent null reference exceptions, always perform null checks, especially when dealing with data retrieved from external sources or user inputs:
string name = GetNameFromDatabase(); int nameLength = name?.Length ?? 0; // Safe null check
Here, the null-conditional operator (?.
) ensures that Length
is only accessed if name
is not null. The null-coalescing operator (??
) provides a default value (0 in this case) if the variable is null.
2. Misusing String Concatenation
Concatenating strings inappropriately can result in inefficient code, especially when dealing with large datasets. Using the +
operator for repeated string concatenation inside loops can lead to performance bottlenecks:
string result = ""; foreach (var item in items) { result += item; // Inefficient string concatenation }
Instead, use the StringBuilder
class for better performance:
StringBuilder resultBuilder = new StringBuilder(); foreach (var item in items) { resultBuilder.Append(item); } string result = resultBuilder.ToString();
StringBuilder
efficiently handles string concatenation by allocating memory dynamically, resulting in better performance, especially for large datasets.
3. Overlooking Exception Handling
Ignoring or improperly handling exceptions can make debugging challenging and lead to unpredictable behavior. It’s crucial to catch and handle exceptions appropriately:
try { // Code that might throw an exception } catch (Exception ex) { Console.WriteLine($"An error occurred: {ex.Message}"); }
However, catching the base Exception
class is generally discouraged. It’s better to catch specific exceptions relevant to the operation to handle errors more effectively.
4. Misusing the ‘using’ Statement
The using
statement in C# is primarily used for managing resources like file streams or database connections. However, it’s a common mistake to misuse it with objects that don’t implement IDisposable
, leading to unnecessary complexity:
using (var obj = new SomeNonDisposableObject()) { // Code that doesn't require resource management }
In this case, omitting the using
statement is more appropriate:
var obj = new SomeNonDisposableObject(); // Code without 'using' statement
Only use the using
statement for objects that implement IDisposable
and require proper resource management.
5. Neglecting Asynchronous Programming Best Practices
With the widespread use of asynchronous programming in modern C# applications, neglecting best practices can lead to issues like deadlocks or inefficient resource usage. One common mistake is blocking on asynchronous code using .Result
or .Wait()
:
Task<int> result = SomeAsyncMethod(); int value = result.Result; // Blocking call
This can lead to deadlocks, especially in UI applications. Instead, use await
to asynchronously wait for the result:
int value = await SomeAsyncMethod(); // Non-blocking call
Always ensure that asynchronous code is handled appropriately to maintain responsiveness and avoid potential issues.
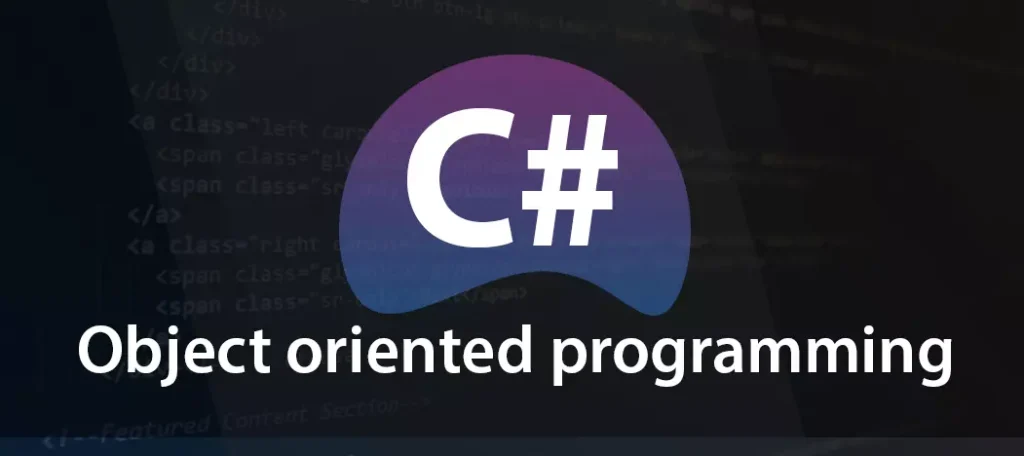
Conclusion
Avoiding common mistakes in C# requires a combination of good coding practices, familiarity with language features, and adherence to best practices. By being mindful of null checks, string concatenation, exception handling, proper use of the using
statement, and understanding asynchronous programming, developers can write more robust, maintainable, and efficient C# code. Regular code reviews, testing, and staying informed about language updates contribute to building high-quality C# applications that are less prone to common pitfalls.